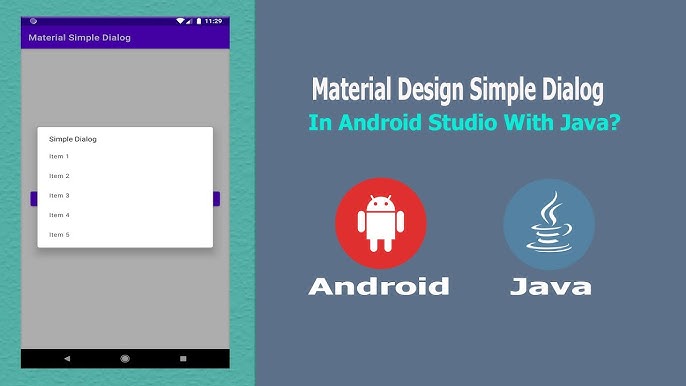
January 22, 2025
Mastering Material Design Dialogs in Android
How do the best Android applications develop clean, useful dialogs that improve user interaction? Google's Material Design approach keeps applications looking current and consistent. User interactions like confirmations, alarms, and form inputs need dialogs, and MaterialAlertDialogBuilder makes custom dialogs easy to create. Let us learn this essential Android development component.
What Are Material Design Dialogs?
Material Design dialogs are popup windows above the primary interface that convey important information or seek user action. Modern app design relies on them for consistent and attractive user interaction. Dialogues let your app communicate, whether it be a confirmation prompt, error message, or custom form.
Material Design for dialogs improves their design and connects your app with Google's user experience guidelines, making it more intuitive and professional.
Setting Up MaterialAlertDialogBuilder
Add Material Components to your project before developing Material Design dialogs. Add the following dependency to your build.gradle file:
implementation 'com.google.android.material:material:<version>'
MaterialAlertDialogBuilder makes basic dialogs easy after setup. As an example:
MaterialAlertDialogBuilder(context)
.setTitle("Sample Title")
.setMessage("This is a basic Material Dialog.")
.setPositiveButton("OK") { dialog, _ -> dialog.dismiss() }
.show()
This piece of code shows how to construct a basic dialog with a title, message, and "OK" button.
Creating Custom Dialogs
Why customize dialogs? Branded apps and user processes benefit from customized dialogs. As you know basic dialogs only support basic tasks.
Step 1: Design the Custom Layout
Start by creating a custom XML layout like custom_dialog.xml:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/dialog_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Custom Title"
android:textSize="18sp"/>
<EditText
android:id="@+id/dialog_input"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter text"/>
</LinearLayout>
Step 2: Inflate the Layout in Your Dialog
Inflate and apply the custom layout into MaterialAlertDialogBuilder:
val customView = LayoutInflater.from(context).inflate(R.layout.custom_dialog, null)
MaterialAlertDialogBuilder(context)
.setView(customView)
.setPositiveButton("Submit") { dialog, _ ->
val input = customView.findViewById<EditText>(R.id.dialog_input).text.toString()
// Handle input
dialog.dismiss()
}
.show()
This method enables you construct a custom dialog for your app, improving functionality and appearance.
Best Practices for Material Dialogs
- Keep it concise: To avoid overwhelming customers, stick to one task per each dialog.
- Use clear labels: Dialogue buttons need to have the words OK or Cancel.
- Match your theme: Dialogs should use the same style and color scheme as the rest of your app to look good.
- Prioritize accessibility: Check that screen readers can understand your dialogs.
Conclusion
MaterialAlertDialogBuilder supports robust user communication with simple and configurable dialogs. Material Design beautifies, simplifies, and meets app dialog needs. Use custom layouts to build distinctive and engaging Android app user experiences now!
474 views