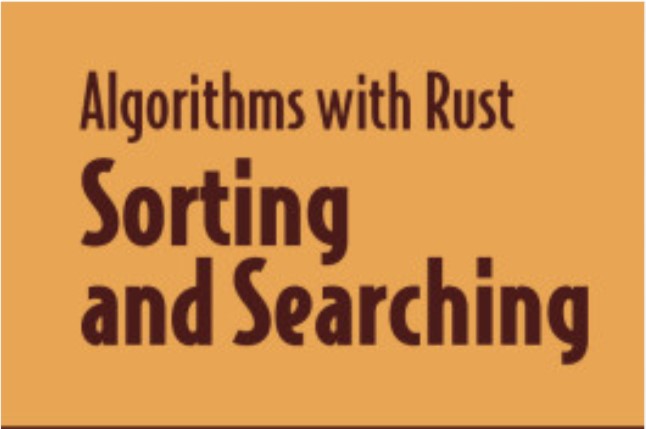
January 14, 2025
Writing High-Performance Algorithms in Rust: Case Studies in Sorting and Searching
For building high-performance systems, Rust is the best language to use. Why? Rust is great for developing algorithms because it is fast, safe for memory, and has zero-cost interfaces. Using examples from real life, this article will show how to sort and search in Rust.
Why Rust for High-Performance Algorithms?
Rust is good for making fast apps because it manages system resources in a low-level way. Unlike garbage collection languages, Rust does not use extra computational cost to make sure memory safety. This stops data races and memory leaks at build time.
"Fearless concurrency" in Rust lets you use parallelism without crashing, which is great for optimizing algorithms. The rare mix of speed and safety makes Rust a great algorithmic development tool. As the Rust community grows, testing and measuring packages and tools make high-performance algorithms better.
Case Study 1: Efficient Sorting in Rust
Rust's design makes it easy to sort things quickly, which is something that many programs need. There are safety assurances in Rust that other languages do not have for algorithms like QuickSort and MergeSort. Consider this basic QuickSort Rust code:
fn quicksort(arr: &mut [i32]) {
if arr.len() <= 1 { return; }
let pivot = partition(arr);
quicksort(&mut arr[0..pivot]);
quicksort(&mut arr[pivot + 1..]);
}
Rust also has complex optimization features like unsafe blocks for manual memory management and SIMD for parallel processing. When performance benefits are greater than safety concerns, these tools optimize. The criteria crate in Rust helps developers figure out how fast loop unrolling and in-place sorting work.
Case Study 2: Optimized Searching in Rust
Fast searching is important for application speed, and Rust's ability to protect memory makes it a great tool for implementing search algorithms. The binary search method splits the search space in half every time. That's why, it is simple to use the binary search function in Rust's standard library:
fn binary_search(arr: &[i32], target: i32) -> Option<usize> {
let mut left = 0;
let mut right = arr.len();
while left < right {
let mid = (left + right) / 2;
if arr[mid] == target { return Some(mid); }
else if arr[mid] < target { left = mid + 1; }
else { right = mid; }
}
None
}
In other low-level languages, out-of-bounds mistakes are widespread. Rust prevents them. Rust's standard library offers fast B-trees and hash maps for complicated data structures.
Conclusion
Safety and control make Rust ideal for high-performance algorithm development. Rust can build credible, efficient apps beyond sorting and searching. Why not try Rust, a high-performance computing leader with a strong community and abundant tooling?
247 views