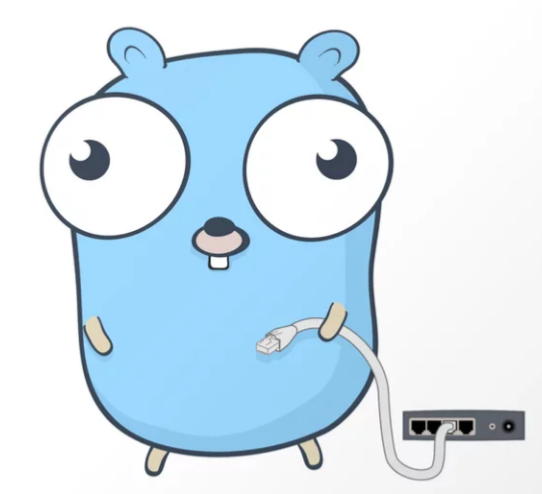
Have you wished to design a flexible app without affecting its essential functionality? You can do that using Go's plugins! Imagine creating a flexible system that dynamically loads new features without redeploying or restarting the app. This blog will explain how Go's plugin package lets you develop modular, dynamically loadable components that make applications extendable and easy to update.
What is the plugin Package?
Go's plugin package allows shared object file loading and control at runtime. Create and load application pieces without main functionality. These "plugins," or components, can include bespoke business logic or functionality.
Go dynamically loads plugins, making it perfect for command-line tools, server-side programs, and apps that enable third-parties contribute features. Your app becomes versatile and future-proof with plugins.
Basics of Creating and Using Plugins
Go plugins are easy to create and use once you understand them. Breaking down:
Creating a Plugin
You must specify the plugin's functionality before creating it. Creating an app interface or techniques is typical. You can put your code into a .so (shared object) file using Go's tools.
Here's a simple plugin that exposes Hello function:
// hello.go
package main
import "fmt"
func Hello() {
fmt.Println("Hello from the plugin!")
}
To compile the plugin, use the following command:
go build -buildmode=plugin -o hello.so hello.go
Loading a Plugin
So, you can use the plugin.Open() method to load the above developed plugin into Go. At runtime, this allows you to call plugin-exported functions and methods.
Here's a simple example code of loading and using the plugin's Hello function:
// main.go
package main
import (
"fmt"
"plugin"
)
func main() {
plug, err := plugin.Open("hello.so")
if err != nil {
fmt.Println("Error opening plugin:", err)
return
}
helloSymbol, err := plug.Lookup("Hello")
if err != nil {
fmt.Println("Error finding Hello symbol:", err)
return
}
hello := helloSymbol.(func()) // Type assertion to call Hello function
hello()
}
Advanced Plugin Use Cases
Dynamic Updates
A primary advantage of plugins is the ability to update or extend program functionality without changing its core. Update and reload the plugin to add features or fix bugs without altering the main app.
Third-Party Plugin Integration
Go allows third-party plugins. Your application can receive runtime plugins from outside developers. This lets you build a versatile app with many database drivers and custom authentication methods without modifying the source.
Error Handling and Debugging
Plugins are flexible, but error management is vital. Make sure your application gracefully handles plugin failures and compatibility concerns. Plugin debugging can be difficult, so provide strong logs and simple interfaces to help.
Best Practices for Working with Plugins
Interface Design
For plugin integration, interfaces must be explicit and flexible. The plugin should provide the host program basic, well-defined methods. The plugin system gets more manageable and compatible as your project expands.
Versioning
Plugins may change like other apps. Control plugin versioning to prevent issues. Must have backward compatibility and plugin version management.
Security Considerations
Untrusted plugins can be dangerous. Your plugin system should validate plugins before loading them, and limiting their use decreases security threats.
Conclusion
Modular, expandable applications that dynamically load and manage additional components are possible using Go's plugin package. Best practices and plugins may make applications more adaptive and updateable without major modifications. Explore the plugin system now to add this intriguing functionality to Go projects.
384 views