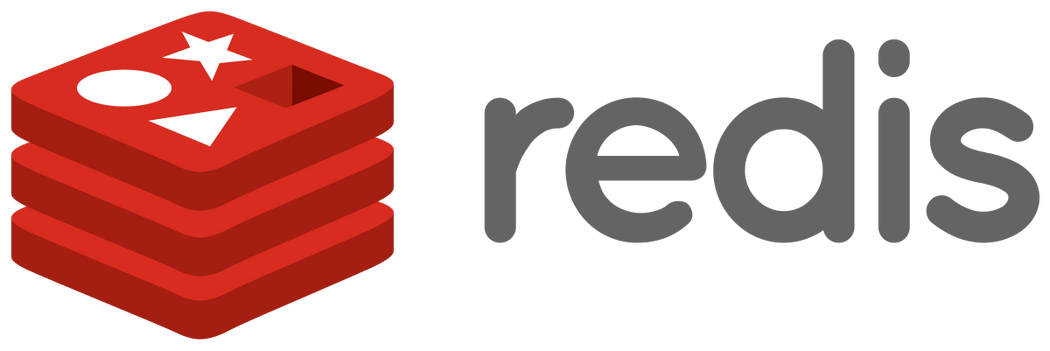
Tired of sluggish apps and excessive latency? With Redis, the world's most popular in-memory data store, and caching, your app can perform lightning-fast. Redis and Go are ideal for building scalable, high-performance apps. This blog post will discuss advanced Redis caching methods to improve your Go app's speed.
Understanding Caching and Redis
Memory-stored caching retrieves data quicker than databases. Web app responsiveness requires it. Redis, an open-source in-memory data structure store, is used for caching. Fast read/write speeds and support for strings, hashes, lists, etc. make it perfect for caching.
Redis stores frequently accessed data like API answers, user sessions, and complex objects in Go to reduce database queries. Go applications that use Redis run better and are more scalable and responsive.
Advanced Redis Caching Techniques in Go
TTL (Time-to-Live) Caching
Redis' TTL (Time-to-Live) feature, which sets cache entry expiration times, is strong. Redis removes expired cached data automatically. TTL keeps cached data current and relevant, reducing the likelihood of stale data.
LRU (Least Recently Used) Caching
Redis provides memory management strategies, including LRU (Least Recently Used) caching. Redis can automatically remove seldom used data to create place for new items when memory runs short. LRU is appropriate for high-performance applications with memory constraints since it caches a limited, relevant dataset.
Hashing with Redis
With Redis, you can store complicated data structures like hashes. Hashes are handy for storing many data under a key, such as user profiles or product information. Compared to storing attribute keys, Redis hashes minimize memory costs. This is useful for storing structured, bigger items.
Pub/Sub for Cache Invalidation
Pub/Sub works for distributed systems with cache invalidation issues. When data changes, Redis' Pub/Sub mechanism may broadcast cache invalidation notifications across services. This eliminates differences by using the latest data in all application instances.
Implementing Advanced Caching Techniques in Go
Here's a quick example of using TTL and LRU with Redis in Go:
package main
import (
"fmt"
"log"
"github.com/go-redis/redis/v8"
"context"
)
var rdb *redis.Client
func main() {
ctx := context.Background()
rdb = redis.NewClient(&redis.Options{
Addr: "localhost:6379",
})
// Set a cache with TTL of 5 seconds
err := rdb.SetEX(ctx, "user:123", "John Doe", 5 * time.Second).Err()
if err != nil {
log.Fatalf("Could not set cache: %v", err)
}
// Get the cache
val, err := rdb.Get(ctx, "user:123").Result()
if err != nil {
log.Fatalf("Could not get cache: %v", err)
}
fmt.Println("Cached value:", val)
// Implementing LRU by limiting cache size
rdb.ConfigSet(ctx, "maxmemory", "100mb")
rdb.ConfigSet(ctx, "maxmemory-policy", "allkeys-lru")
}
In the above example code, we use the SetEX command to store a value in Redis with a 5-second TTL to keep the data fresh and delete it when it expires. Use Get to get the cached value. After than we set a maximum memory limit for Redis to employ the Least Recently Used (LRU) eviction mechanism to enhance speed and cache relevance. TTL-based caching and memory optimization provide a powerful caching method.
Best Practices and Pitfalls to Avoid
Avoid Over-Caching
Caching is powerful, but caching everything might waste RAM. Only cache costly or computationally demanding data. Caching unnecessary data slows speed and raises computational expenses.
Data Inconsistencies
Cache invalidation is important when data changes. One of the largest caching system mistakes is delivering customers old or inaccurate data. Using TTL and Pub/Sub helps reduce this danger.
Error Handling
Consider network problems while integrating Redis into your Go app. Always handle failures graciously to keep your application running while Redis is down.
Performance Monitoring
Check Redis configuration often for best performance. Redis' MONITOR command and others may count cache hits, misses, and memory use.
Conclusion
Go's advanced Redis caching boosts performance and scalability. TTL, LRU, Redis hashes, and Pub/Sub protect and update data. You can follow best practices, monitor performance, and avoid common errors to enhance Redis caching in Go projects.
189 views