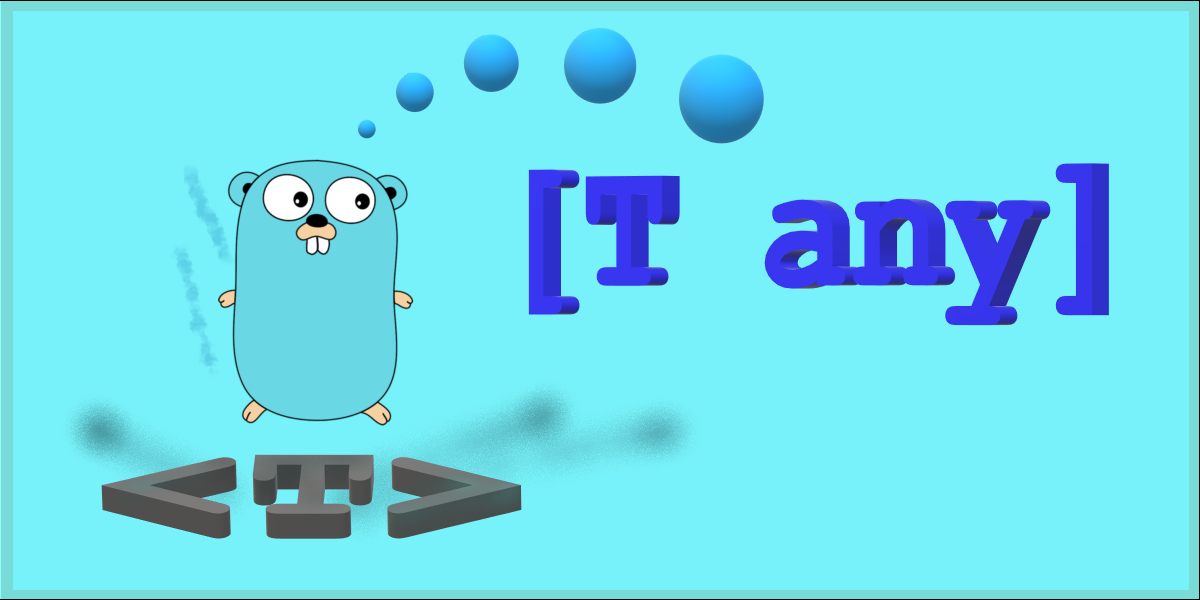
January 02, 2025
Custom Generics in Go 1.18+: Building Type-safe Data Structures
Do you ever write numerous data structures for distinct types? Your situation is not unique. Code duplication was necessary to manage types cleanly and reusablely before Go 1.18. Now that generics are in Go 1.18+, this is no longer a problem. This article will show how to use generics to design type-safe, reusable data structures that decrease duplication and increase maintainability.
What Are Generics and Why Should You Use Them?
Go generics let you construct type-safe functions and data structures for any data type. Generics enable you create a function or type that works on several types without skipping compiler type correctness tests.
Generics can boost different programming aspects:
- Type safety: Correct type use is necessary for the computer, which stops mistakes from happening at runtime.
- Reusability: Apply a single function or data structure to several kinds without rewriting it.
- Flexibility: Generics provide abstract and adaptable data processing methods.
Generics in Go 1.18+ allow developers obtain these advantages while keeping the language simple and efficient.
Setting Up Go 1.18+ for Generics
To use generics, use Go 1.18 or above. Type parameters in Go 1.18+ enable you to build versatile, multi-data-type types. With these declared inside square brackets ([]), the function or type can take any type.
Here's how a basic generic function might look:
func Print[T any](value T) {
fmt.Println(value)
}
In the above code, T is a type parameter and any is a type constraint. This type-safe, reusable function accepts any type.
Building a Custom Type-safe Data Structure
After learning generics, let us design a new data structure. A generic stack can handle any value type, guaranteeing type safety while pushing and popping objects.
Here's how you can build a generic stack in Go:
package main
import "fmt"
// Define a generic Stack type
type Stack[T any] struct {
items []T
}
// Push method adds an item to the stack
func (s *Stack[T]) Push(item T) {
s.items = append(s.items, item)
}
// Pop method removes the last item from the stack
func (s *Stack[T]) Pop() (T, bool) {
if len(s.items) == 0 {
var zeroValue T
return zeroValue, false
}
item := s.items[len(s.items)-1]
s.items = s.items[:len(s.items)-1]
return item, true
}
func main() {
// Using Stack with int type
intStack := Stack[int]{}
intStack.Push(1)
intStack.Push(2)
fmt.Println(intStack.Pop()) // Output: 2, true
// Using Stack with string type
stringStack := Stack[string]{}
stringStack.Push("hello")
stringStack.Push("world")
fmt.Println(stringStack.Pop()) // Output: world, true
}
Advantages of Using Custom Generics for Data Structures
Type safety is the main benefit of generic data structures. The compiler guarantees that Go's generics use compatible types, preventing runtime issues from type mismatches.
Generics also increase code flexibility. Instead of developing data structures for each type, you may utilize a generic data structure like Stack[T] throughout your application.
Generics reduce code duplication. Make one stack implementation and reuse it for integers, strings, and other kinds. The code is cleaner and easier to maintain.
Conclusion
Go 1.18+ generics provide versatile, reusable, and type-safe programming. Generics let you design unique data structures like the Stack that work with any type while retaining Go's robust typing. Generics can reduce code duplication, increase maintainability, and assure robustness and type safety as you build increasingly complex systems. Explore generics in Go now to improve your programming!
190 views