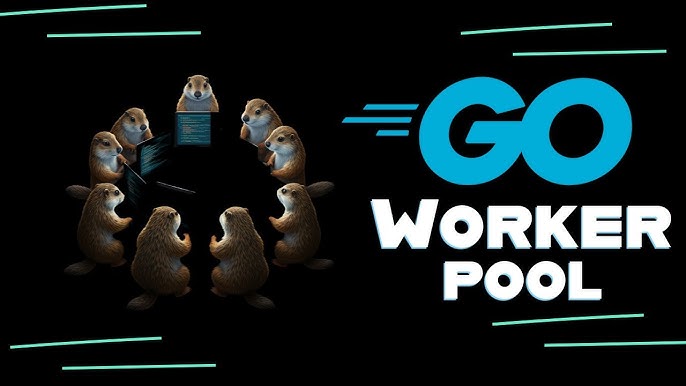
December 31, 2024
Asynchronous Programming with Worker Pools in Go
Have you ever had to multitask without overloading your system? Although Go's goroutines are great for concurrency, managing them may be difficult. Here come worker pools. This article shows how to use an asynchronous worker pool design in Go to do tasks simultaneously without overloading the system.
What is a Worker Pool?
A worker pool handles queued jobs with several "workers" (goroutines). A worker pool reduces CPU and memory misuse by limiting the number of active workers.
Batch processing, background jobs, and parallel tasks that need resource management work well with this strategy. The worker pool keeps the number of active workers low and distributes tasks effectively.
Implementing a Simple Worker Pool in Go
Go uses channels to construct a worker pool for job distribution. Here's how to set up a simple worker pool that processes tasks simultaneously:
package main
import (
"fmt"
"time"
)
type Job struct {
ID int
}
func worker(id int, jobs <-chan Job, results chan<- string) {
for job := range jobs {
time.Sleep(1 * time.Second) // Simulate work
results <- fmt.Sprintf("Worker %d processed job %d", id, job.ID)
}
}
func main() {
jobs := make(chan Job, 5)
results := make(chan string, 5)
// Create workers
for i := 1; i <= 3; i++ {
go worker(i, jobs, results)
}
// Enqueue tasks
for j := 1; j <= 5; j++ {
jobs <- Job{ID: j}
}
close(jobs)
// Collect results
for a := 1; a <= 5; a++ {
fmt.Println(<-results)
}
}
At this point, there are three workers, and each one handles jobs from the jobs channel. After completing a task, workers submit the result to the results channel. Workers share tasks and process them simultaneously using this configuration.
Controlling Concurrency in Worker Pools
Controlling concurrent workers is a major advantage of worker pools. Buffered channels restrict the amount of workers processing tasks at once, protecting your application from overloading.
Adjust worker count to limit pool to 3 active workers:
workerCount := 3
jobs := make(chan Job, 10)
results := make(chan string, 10)
for i := 1; i <= workerCount; i++ {
go worker(i, jobs, results)
}
Error Handling and Graceful Shutdown
Real time task processing issues could come up. Handle these issues and gently close the worker pool after finishing all tasks.
Imagine a worker making a mistake. You can use error handling and graceful termination sync.WaitGroup for all workers to finish:
var wg sync.WaitGroup
func worker(id int, jobs <-chan Job, results chan<- string) {
defer wg.Done() // Decrement the counter when the worker finishes
for job := range jobs {
// Simulate error handling for failed tasks
if job.ID%2 == 0 { // Simulating a failure
results <- fmt.Sprintf("Worker %d failed to process job %d", id, job.ID)
continue
}
time.Sleep(1 * time.Second) // Simulate work
results <- fmt.Sprintf("Worker %d processed job %d", id, job.ID)
}
}
func main() {
jobs := make(chan Job, 5)
results := make(chan string, 5)
// Launch workers
for i := 1; i <= 3; i++ {
wg.Add(1)
go worker(i, jobs, results)
}
// Enqueue tasks
for j := 1; j <= 5; j++ {
jobs <- Job{ID: j}
}
close(jobs)
// Wait for workers to complete
wg.Wait()
close(results)
// Collect results
for res := range results {
fmt.Println(res)
}
}
Conclusion
Go worker pools are an excellent way to control concurrency while running several processes without overloading the system. Monitoring active workers and gracefully shutting off can develop reliable systems. So after reading this article, are you ready to increase Go application concurrency using worker pools!
515 views