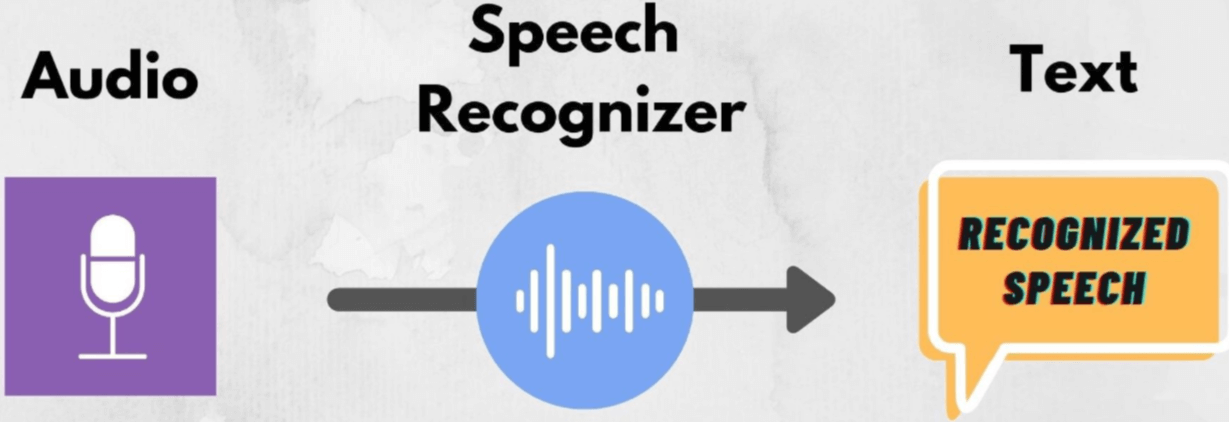
December 18, 2024
Voice to Text in Python: Build Your Speech Recognition Tool
Have you ever wanted to convert your speech to text with a few lines of code? Python lets you quickly construct a speech recognition tool that can use voice commands or transcribe audio to text. What about building your own Python voice-to-text tool today? Let's do it...
Prerequisites
Install Python 3+ and learn Python libraries before we begin. These libraries are essential for speech recognition: SpeechRecognition and pyaudio for microphone capture.
What you need:
- Python 3+
- SpeechRecognition and pyaudio libraries
Setting Up Your Environment
First, install the necessary libraries using pip. Open your command line and run:
pip install SpeechRecognition pyaudio
Pyaudio installation on Windows could require extra steps. Pythonlibs and Gohlke's Pythonlibs provide precompiled pyaudio for manual installation.
Writing the Code
Now, let's dive into the Python code to create a basic speech-to-text tool.
Initialize SpeechRecognition
Import the speech_recognition module and create a recognizer object. The object will record and recognize your speech.
import speech_recognition as sr
recognizer = sr.Recognizer()
Convert Speech to Text
Next, let's write the code to capture audio and convert it to text. We'll use the microphone as our audio source:
with sr.Microphone() as source:
print("Say something...")
recognizer.adjust_for_ambient_noise(source) # Optional: Adjusts for ambient noise
audio = recognizer.listen(source)
Once we've captured the audio, we can pass it to the recognizer to convert it into text:
try:
print("You said: " + recognizer.recognize_google(audio))
except sr.UnknownValueError:
print("Sorry, I did not understand that.")
except sr.RequestError:
print("Sorry, the speech service is unavailable.")
In the above code, I transcribe the voice-to-text using Google's free speech recognition tool. To address audio quality and service disruption concerns, I've added error handling.
Testing and Enhancing the Tool
It is now time to try out the tool. Run the Python script and talk into your microphone when requested. Your words should appear on the screen as text.
Set up your microphone properly and make sure your area is quiet enough for accurate identification if you make mistakes. Noise reduction and microphone sensitivity can enhance accuracy.
Potential Use Cases and Expanding Features
This tool has many practical uses. Note-taking, voice-based app instructions, and disability accessibility capabilities like dictation are possible.
To analyze and process text further, use NLP technologies. Build a voice-activated assistant that understands your orders.
Conclusion
With a few Python lines, you can create a voice-to-text tool! This is only the start, you may modify and enhance this tool infinitely. Speech recognition in Python is useful for personal and professional projects. Try out your new tool and see what happens when you use your voice to manipulate the code!
262 views