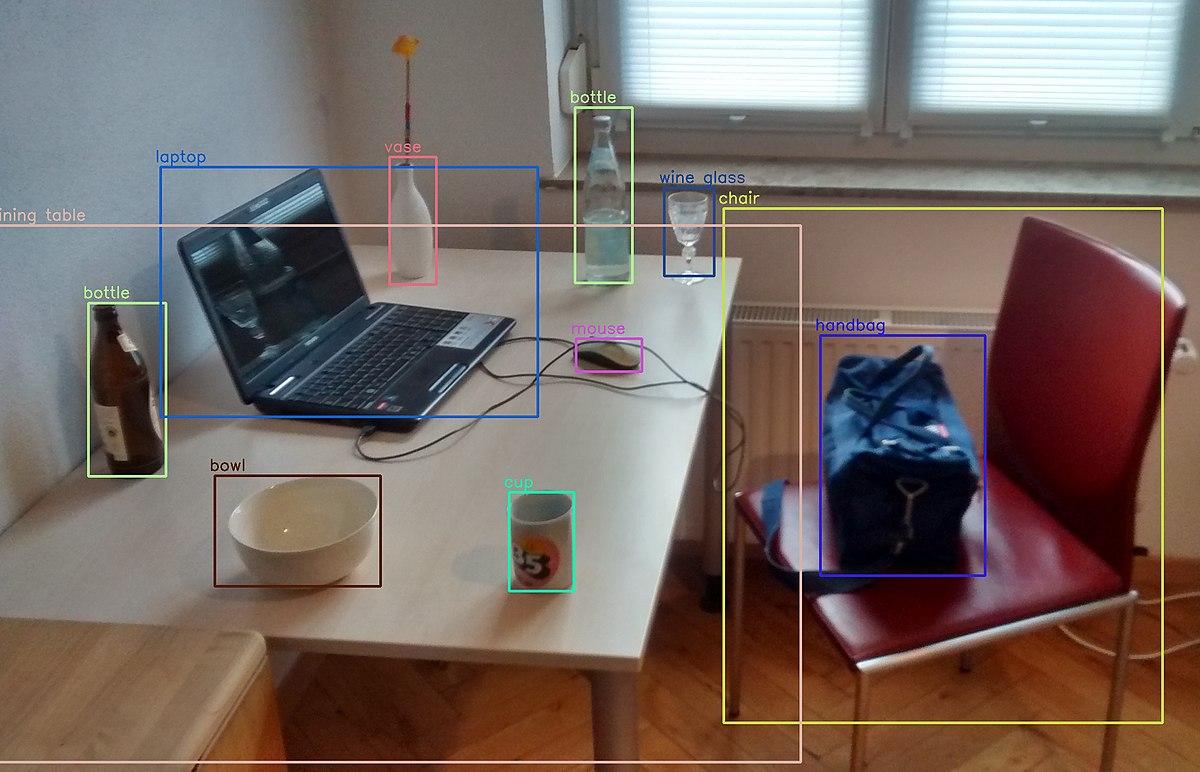
December 13, 2024
Real-Time Object Detection in Python with YOLO and OpenCV
Ever wondered how self-driving vehicles or smart surveillance systems can recognize things in real-time? Object detection is the key, and YOLO is a great tool. This article shows how to use YOLO with OpenCV in Python for fast, accurate, real-time object identification.
Why Use YOLO and OpenCV for Object Detection?
From security to healthcare, object detection is essential. Speed and efficiency distinguish YOLO. Unlike typical object detection algorithms, YOLO scans a picture in one pass, making it quick enough for real-time applications. Python developers may easily integrate YOLO with OpenCV to create complex object detection systems.
Setting Up the Environment
Install Python and libraries before starting the coding. Essential project tools are:
- Python 3.x: Install from python.org.
- OpenCV: Install using pip install opencv-python.
- YOLOv3 weights and configuration files: You can download them from the official YOLO website.
Once these are set up, we're ready to begin coding.
Code Walkthrough for Real-Time Detection
Step-by-step instructions for building a real-time object detection system using YOLO and OpenCV.
Load YOLO Weights and Config Files: The cv2.dnn.readNet method reads the YOLO weights and settings file into OpenCV.
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
Set Up OpenCV for Real-Time Video Capture: We capture webcam or video file feeds using OpenCV. OpenCV continually scans and feeds frames to YOLO for real-time processing.
cap = cv2.VideoCapture(0) # 0 for webcam feed
Running YOLO on Video Frames: YOLO uses a neural network to predict things in each frame. How to process the frame:
blob = cv2.dnn.blobFromImage(frame, 0.00392, (416, 416), (0, 0, 0), True, crop=False)
net.setInput(blob)
outs = net.forward(output_layers)
Display Detected Objects: YOLO draws bounding borders and labels on products it finds. OpenCV can show results live after detection:
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5: # Set confidence threshold
# Draw bounding boxes
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(frame, label, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
This stage examines each frame, finds objects, and overlays bounding boxes and confidence ratings to show real-time object detection.
Performance Optimization Tips
Consider the following to improve real-time detection:
- Reduce Input Image Size: Smaller images are quicker for YOLO to analyze, but accuracy may suffer.
- Set Confidence Threshold: Display only items with confidence over 0.5 to minimize false positives.
- Use a Smaller YOLO Version: YOLOv4-tiny and YOLOv5-lite are lighter variants of YOLO that exchange accuracy for speed, which is useful in real-time applications.
Conclusion
This post has shown you how simple YOLO and OpenCV make real-time object detection in Python. YOLO's efficiency and OpenCV's simplicity let you easily install an object detection system for many applications. Consider customizing YOLO for specific purposes or testing video feeds for complex systems.
With a few lines of code, you can detect things in real time and use computer vision in your applications!
402 views