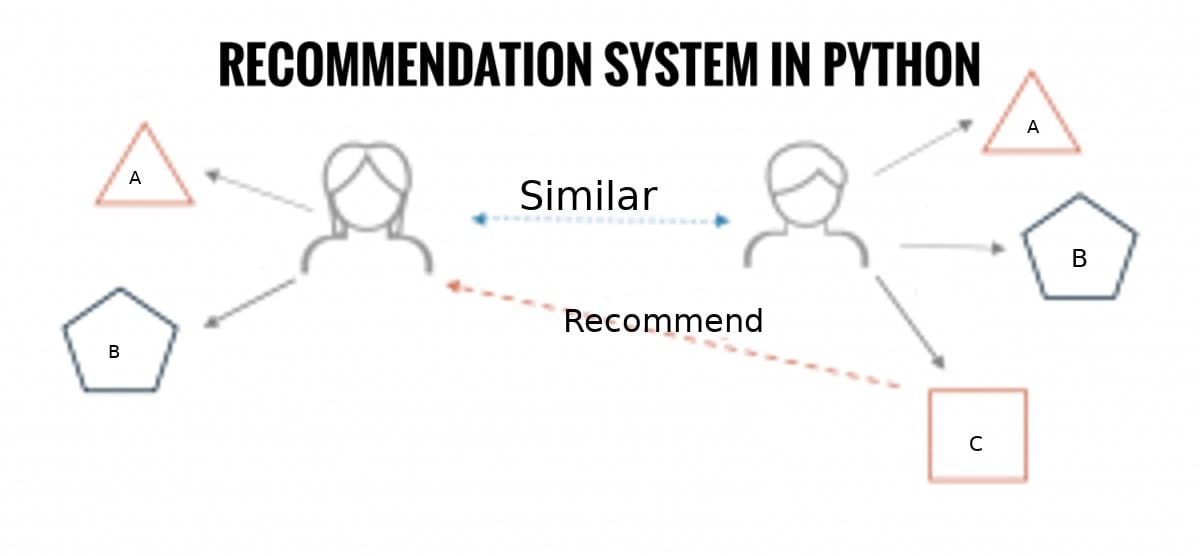
December 10, 2024
Implementing a Recommendation System in Python: From Scratch to Ready-to-Use
Have you ever thought about how Netflix, Amazon, or other platforms recommend you the right movie or products and know what you want? You can do this too by creating your own of recommendation systems. Today, in this blog post I will show you how to construct a recommendation system from the start using Python. Let's get into it!
Basics of Recommendation Systems
A recommendation system uses algorithms to suggest you movies, products, and articles by analyzing different factors. Let's see the three primary recommendation systems exist:
- Content-Based Filtering: Recommends things based on historical user preferences.
- Collaborative Filtering: Recommends items based on comparable user interests.
- Hybrid Models: Combine content-based and collaborative approaches for enhanced suggestions.
In this article, I'll discuss collaborative filtering, which uses other users' activity to promote a user. This algo is popular as it works well with user interaction data like ratings or buying history.
Setting Up Your Python Environment
So, before getting started, you need to install some Python libraries for arithmetic, and machine learning tasks. You can run this command to install them:
pip install pandas numpy scikit-learn
Data Preparation
Once you've setup the environment, now it's time to load your dataset in model. Any recommendation system relies on data. I've used the movie reviews dataset. This is how you can load:
import pandas as pd
# Load the dataset
ratings = pd.read_csv('movie_ratings.csv')
print(ratings.head())
Building a Collaborative Filtering Recommendation System
Collaborative filtering suppose persons with similar preferences will evaluate things similarly. You can use user- or item-based collaborative filtering. To find people with similar interests, I'll examine user-based collaborative filtering.
- Calculate Similarity: A similarity measure, like cosine similarity, can help you find people who rate things in the same way.
from sklearn.metrics.pairwise import cosine_similarity
# Creating a user-item matrix
user_item_matrix = ratings.pivot(index='user_id', columns='movie_id', values='rating')
user_similarity = cosine_similarity(user_item_matrix.fillna(0))
- Predict Ratings: Predict ratings by averaging the ratings of similar users.
import numpy as np
# Making predictions by averaging ratings of similar users
def predict_ratings(user_id, movie_id):
# Similar users' ratings for the movie
similar_users = user_similarity[user_id]
# Weighted average for recommendation
weighted_ratings = np.dot(similar_users, user_item_matrix[movie_id].fillna(0))
return weighted_ratings / np.sum(similar_users)
- Generate Recommendations: Use the predicted ratings to recommend items with the highest scores that the user hasn't rated yet.
Evaluating the Recommendation System
MSE, which compares expected and actual ratings, can evaluate a recommendation system. Here's a short example code:
from sklearn.metrics import mean_squared_error
# Example evaluation
mse = mean_squared_error(actual_ratings, predicted_ratings)
print(f"Mean Squared Error: {mse}")
Conclusion
So, this was a simple yet working Python recommendation system written from scratch! From loading and preparing data to developing, analyzing, and upgrading a recommendation system, above discussed steps will got you all the basics. But there is more to explore for you, so try hybrid models or alternative algorithms for better recommendations.
67 views