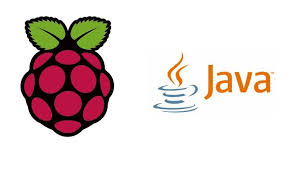
December 06, 2024
Control an LED using Java and Raspberry Pi GPIO Pins
Hi fellas, are you curious whether Java code can control hardware like LEDs? Your curiosity is going to stop by the end of this blogpost. Stay tuned with me.
Raspberry Pi lets you do it! You can control LEDs, generators, and sensors using Raspberry Pi's GPIO pins. Due to Java's platform neutrality, you can create software and hardware code in one language.
In this blog post, I'll show you how to use Pi4J to control an LED in Java. The final result will be in a blinking LED!
Materials Required
Before we start creating the project, you must have the following materials:
- Raspberry Pi with GPIO access
- Breadboard
- LED (any color)
- 220-ohm resistor (to protect the LED)
- Jumper wires
- Java Development Kit (JDK) installed on your Raspberry Pi
- Pi4J library, a Java library for controlling GPIO pins
Setting up the Hardware
Let's start by setting up the hardware first before writing the code to make it functional like; wiring the LED to the Raspberry Pi.
- First, place the LED on the breadboard.
- Now, connect the longer leg (anode) of the LED to GPIO pin 17 on the Raspberry Pi.
- Next, you've to attach a 220-ohm resistor to the shorter leg (cathode) of the LED.
- And, at the end, connect the other end of the resistor to any ground (GND) pin on the Raspberry Pi.
Java Code Implementation
I'll use the Pi4J library to control the GPIO pins. Let's see the code to blink the LED on and off after every second.
import com.pi4j.io.gpio.*;
import com.pi4j.io.gpio.event.*;
public class LEDControl {
public static void main(String[] args) throws InterruptedException {
final GpioController gpio = GpioFactory.getInstance();
final GpioPinDigitalOutput led =
gpio.provisionDigitalOutputPin(RaspiPin.GPIO_01, "LED", PinState.LOW);
while (true) {
led.toggle(); // Blink LED
Thread.sleep(1000); // 1-second interval
}
}
}
Let me clarify you what I've done in the above code. I've imported Pi4J classes to access GPIO functions. I've created the GPIO controller instance that helps to manage the pins. After that, I've setup GPIO 01 (pin 17) as output pin to manage the LED. And the last point to discuss is, the while(true) loop toggles LED state every second using Thread.sleep(1000).
Compiling and Running the Code
Now we've reached the final stage of our project, run the code on your Raspberry Pi: Letââ¬â¢s see how:
- Open the terminal on your Raspberry Pi.
- Compile the Java code
javac LEDControl.java
- Run the code with superuser permissions (necessary for GPIO access):
sudo java -classpath .:pi4j-core.jar LEDControl
Conclusion
Raspberry Pi hardware control using Java brings up new options for you. You've started by blinking an LED! Advanced projects like controlling numerous LEDs, motors, and sensors are coming in the way. Only your creativity limits the potential of Java and physical computing. So, what are you developing next?
289 views