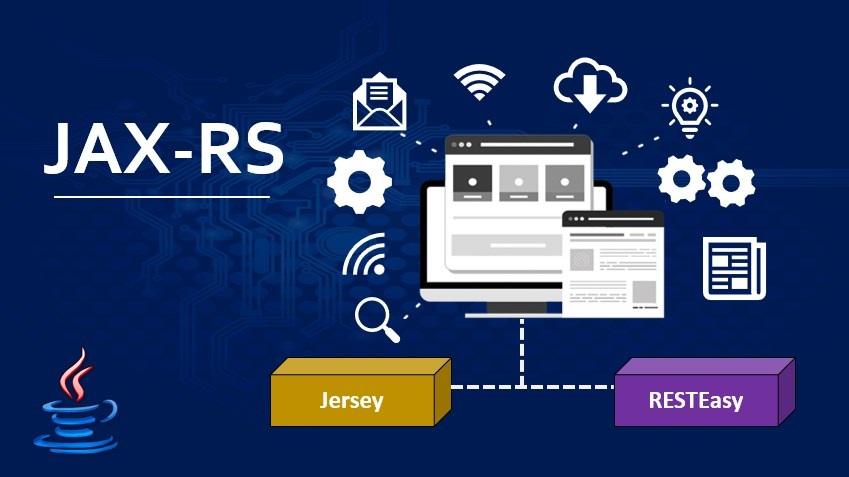
December 03, 2024
Build a basic REST API using Jersey (JAX-RS)
Do you know how many famous web apps communicate with servers easily? It all rotates around REST APIs. If you want to explore RESTful services deeply than Jersey and JAX-RS are great tools for this purpose. In this blogpost, I'll tell you a step-by-step procedure to create a REST API using these structures.
What is Jersey and JAX-RS?
Before seeing its coding implementation, let's first understand what is Jersey and JAX-RS to make things simple for you. Jersey is an open-source Java RESTful web service structure. It is the standard implementation for JAX-RS. Also it's an important element for developing Java REST APIs.
Jersey and JAX-RS both will provide you a robust and flexible solution to create web services, letting developers focus on usefulness rather than complexity.
So, building flexible and maintainable APIs is easy with Jersey and JAX-RS.
Setting Up Your Development Environment
Let's set up the development environment like; you have the right tools, before getting into the coding section. First, you've to install the Java Development Kit (JDK) on your system. Setting up Maven along with JDK is also important, as it helps manage and handle your project dependencies. You can use an IDE like Eclipse or IntelliJ for a simpler development experience.
To create a new Maven project, follow these steps:
- First, open your IDE and select "New Maven Project" from there.
- Choose the archetype for a simple project and move to the setup stage.
Next, you'll need to add Jersey dependencies in your pom.xml file to integrate the required libraries.
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.x</version>
</dependency>
Creating Your First RESTful Service
So, you've set up your development environment successfully, now it's time to create your first RESTful service! Let's see the two simple steps below:
- Step 1: Create a simple Java class named HelloWorldService, and annotate it with @Path to define the API endpoint:
@Path("/hello")
public class HelloWorldService {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String sayHello() {
return "Hello, World!";
}
}
- Step 2: In the next step, configure your application by creating a subclass of ResourceConfig. This will define your application's root path:
@ApplicationPath("/api")
public class MyApplication extends ResourceConfig {
public MyApplication() {
packages("com.example"); // Package where the service is located
}
}
Running the Application
Here comes the most critical phase, running your app where you'll actually have the idea whether your efforts got true or not. To see your API in action, execute the application in a servlet container like Apache Tomcat. After running, you can test the API using tools like Postman or curl.
Conclusion
Now you've idea how Jersey with JAX-RS let you create a simple REST API in just a few simple clicks. This core knowledge lets you design complex APIs with error handling, data persistence, and more. So why not try it by yourself? Your RESTful world awaits!
313 views