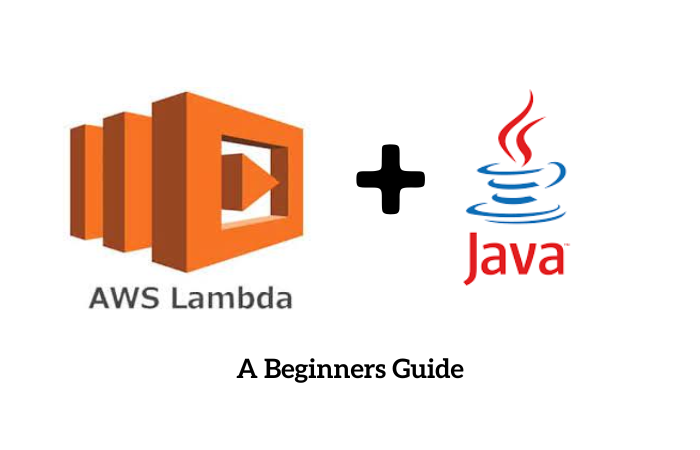
December 02, 2024
How to Write a Simple Lambda Function in Java and Deploy it on AWS?
Are you tired of running heavier codes with the fear of servers, scaling, or infrastructure? Afraid of whether your server will support your written code or not? Now the problem is solved, today I've came up with a serverless computing service, AWS Lambda. Using this, you don't have to worry about the architecture, server, or anything, just focus on writing and running the code.
I'll discuss with you how you can create a simple Lamba function in Java and implement it on AWS step-by-step. Let's get into it.
Prerequisites
First of all set up the environment, just make sure you have the following tools installed and configured on your system:
- Java SDK (JDK 8+ or 11)
- AWS CLI installed and configured with necessary IAM permissions
- Maven (or Gradle) to package the code
- An AWS account with access to Lambda and IAM
Once your environment is ready, now you can move on to the fun part, writing the Lambda function!
Step 1: Writing the Lambda Function in Java
I'll start by writing a simple Java Lambda function that will take an input string and will show a greeting message as output.
Create a New Maven Project
For this, you've to start by setting up a Maven project or a simple Java class. In the Maven pom.xml file, you must have to add the AWS Lambda dependencies:
<dependencies>
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-lambda-java-core</artifactId>
<version>1.2.1</version>
</dependency>
</dependencies>
Write the Lambda Handler
Next, create a Handler class that implements the RequestHandler interface. This interface is provided by AWS and lets you to manage incoming events.
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
public class HelloWorldHandler implements RequestHandler<String, String> {
@Override
public String handleRequest(String input, Context context) {
return "Hello, " + input + "!";
}
}
This function takes an input string (like "World") and returns "Hello, World!".
Step 2: Package the Lambda Function
Once your code is ready, now it's time to package it into a JAR file so AWS Lambda can run it. Use Maven for this step.
Open a terminal, navigate to your project directory, and run:
mvn clean package
This command will generate a JAR file in the target folder.
Step 3: Implement the Lambda Function on AWS
It's time to implement the function to AWS Lambda.
- First, open the AWS Lambda Console.
- Now, click Create Function and then select Author from Scratch.
- After that, provide a function name (e.g., HelloWorldFunction) and select Java 11 as the runtime.
- Upload the JAR file you created in the target folder.
- In the Handler field, enter:
HelloWorldHandler::handleRequest
- At the end, configure basic settings like, 128 MB memory and 30 seconds timeout.
Click Create Function, and your Lambda function will be ready!
Step 4: Testing the Lambda Function
So, you've implemented your function, let's test it.
- Open the AWS Lambda Console and click your function.
- Click Test and create a new test event.
- Now try with giving an input of (World)
- Click Test and check the output, it will be like, (Hello, World!).
If you see the greeting message, your Lambda function is working perfectly!
Conclusion
You saw that how easy was creating and implementing an AWS Java Lambda function. You can install, package, and run codes without servers in a few steps. AWS Lambda simplifies executing and scales smoothly.
After running your first Lambda function, you can investigate advanced use cases. To learn more, try API Gateway or DynamoDB from AWS!
445 views