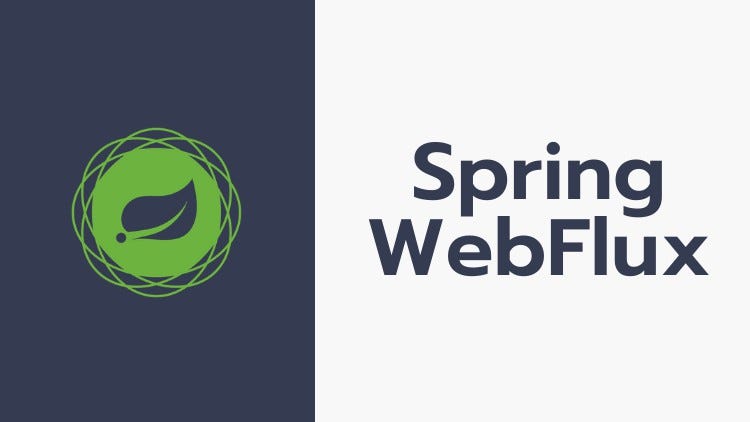
November 28, 2024
Build a simple reactive REST API with Spring WebFlux.
Have you ever thought, how these modern apps can handle thousands of requests at the same time without getting hang. It is reactive programming behind all this, a programming model specially made for non-blocking, asynchronous and event-driven apps. Traditional blocking structures like Spring MVC can trigger scalability issue as well as your website traffic start to grow. This is where Spring WebFlux becomes handy for creating non-blocking and reactive APIs.
So, in this blogpost, I'll tell you how to create a simple yet efficient reactive REST API using Spring WebFlux. Let's get started.
Setting up the Project
Let's start by setting up the environment for project. First, add the required dependencies using Maven or Gradle.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
You can create the project from scratch using Spring Initializr (https://start.spring.io/) or you can also set up the dependencies in your build file manually. Spring WebFlux let's you use these two approaches for building REST APIs:
- Annotation-based with @RestController.
- Functional routing using RouterFunction.
In this guide, we'll use the annotation-based approach to keep things simple.
Creating a Reactive REST Endpoint
Now, to get a product's details, let's create a basic GET endpoint. Spring WebFlux introduces Mono and Flux, which wrap the response in reactive streams:
- Mono: Extract zero or one item.
- Flux: Extracts multiple items in a stream.
Let's understand it with an example of a ProductController using a Mono response:
@RestController
public class ProductController {
@GetMapping("/product/{id}")
public Mono<Product> getProduct(@PathVariable String id) {
return Mono.just(new Product(id, "Laptop", 1500.00));
}
}
The @RestController annotation marks the class as a REST controller, and the Mono stream ensures that the API will show a single product object in a non-blocking way.
Testing the Reactive API
Once your API is ready, now it's time to test it using Postman or Curl:
GET: /product/1
Response:
{
"id": "1",
"name": "Laptop",
"price": 1500.00
}
To write unit tests, you can use the @WebFluxTest annotation, which makes it easy to test WebFlux controllers. Besides this, WebTestClient lets you to send requests and authenticate responses programmatically in your tests.
Conclusion
Spring WebFlux helps design scalable, non-blocking APIs. Wrapping data in Mono or Flux streams helps you manage big amounts of data and simultaneous requests. You can boost the API's performance by connecting to a reactive database like MongoDB or integerating error handling. Explore Spring WebFlux now for creating modern and efficient microservices!
366 views