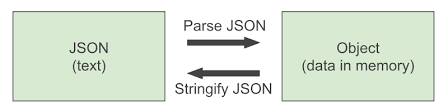
November 06, 2024
Working with JSON in JavaScript: Parsing and Stringifying Data
Hi fellas, did your mind ever popped how different web apps used to exchange data at the backend? Its JSON that's behind everything. JSON (JavaScript Object Notation) is a lightweight data exchange format and the backbone of data communication in modern age web development. This makes it important for web developers to excel in parsing and stringifying JSON data.
What is JSON?
JSON, a text-based format helps to represent structured data as arrays and key-value pairs. It's readable for both machines and humans, due to its data interchange ability. JSON has a very simple formula; in key-value pairs, keys are strings and values can be strings, numbers, arrays, objects, or Booleans. Therefore; from API (Application Program Interface) responses to configuring files, JSON's versatility makes it a popular option among various applications.
Parsing JSON
In JavaScript, using built-in JSON.parse() method you can easily parse JSON. With this function, converting JSON string into JavaScript object is a breeze to access and manipulate data effortlessly.
Here's a quick example:
const jsonString = '{"name": "John", "age": 30}';
const jsonObject = JSON.parse(jsonString);
console.log(jsonObject.name); // Output: John
Here, I took a JSON string and converted it into an object. In this way, you can easily access properties like age and name.
But in some case parsing JSON can also cause errors if your JSON string is malformed. But to tackle this situation, wrap the parsing code in try-catch block:
try {
const jsonObject = JSON.parse(jsonString);
} catch (error) {
console.error("Invalid JSON string:", error);
}
Stringifying JSON
As its name show stringifying, this helps to convert JavaScript object back to JSON string, and for this you can use JSON.stringify() method. This is especially useful when sending data back to a server or saving it locally.
Here's how it works:
const userObject = { name: "Jane", age: 25 };
const userString = JSON.stringify(userObject);
console.log(userString); // Output: {"name":"Jane","age":25}
In this case, the userObject is transformed into a JSON string, preserving its structure. You can also customize the output by adding spacing to make it more readable:
const prettyString = JSON.stringify(userObject, null, 2);
console.log(prettyString);
/*
Output:
{
"name": "Jane",
"age": 25
}
*/
Common Pitfalls
However, working with JSON is simple and easy but there are some common pitfalls too that you should be aware of. JSON does not support circular references in objects, which can lead to errors during the process of stringifying data. Moreover, JSON also can't represent functions and undefined values that can be a limitation in different cases.
Conclusion
Web developers must understand JSON to efficiently handle and communicate data. Excelling in JSON.parse() and JSON.stringify() will let you deal with data easily, making your web applications more durable and efficient. So, dig in and experience JavaScript's appealing JSON features!
531 views