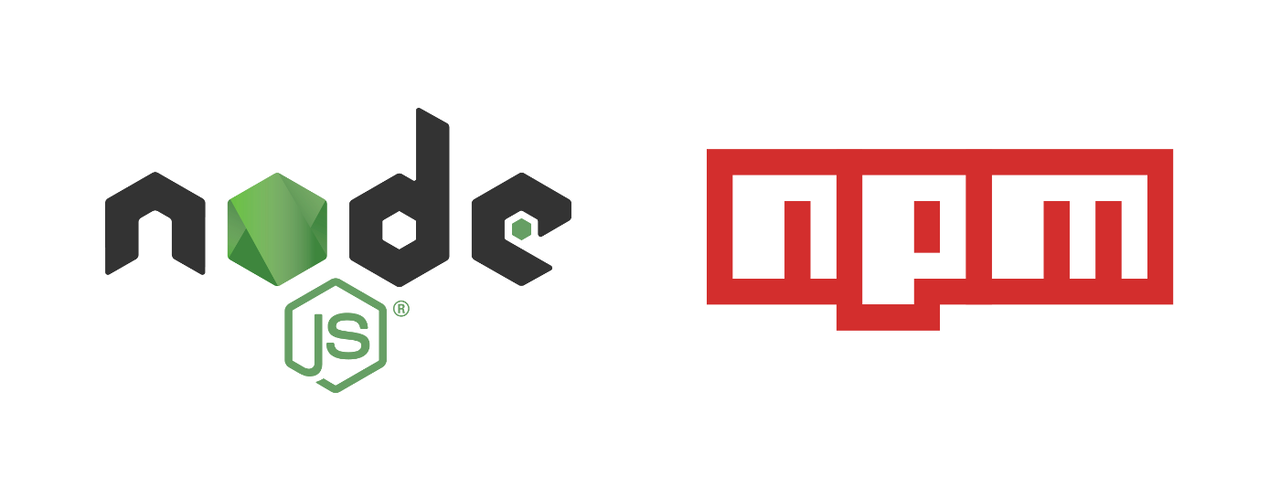
October 03, 2024
Using TensorFlow.js for Machine Learning in the Browser
TensorFlow.js is a robust tool for bringing Machine Learning to the browser directly. Using JavaScript, you can build, train and run different machine learning models with TensorFlow.js. This is like a dream come true for web developers who want to integrate AI with their web applications despite eliminating server-side processing.
In this blog post, I'll walk you through the basics of TensorFlow.js and how use can use it in a browser to perform real-time machine-learning tasks.
What is TensorFlow.js?
JavaScript's very important library, TensorFlow.js, helps the coders to create and run machine learning models within web browsers. This library supports client-side machine learning rather than server-side processing, this allows users to perform computations on their devices instead of performing on remote servers. This improves performance and real-time interactivity as users will perform computations on their own devices and don't have to send their data to any external remote device.
You can run pre-trained models or build your own models using TensorFlow.js. Whenever there are tasks about image recognition, object detection, and text classification in a web application; TensorFlow.js is a winner.
Setting Up TensorFlow.js
TensorFlow.js is very simple and easy to use. If you want to work in a Node.js environment, then you can install it through npm, or you can also integrate it into your projects using the HTML <script> tag.
Using HTML <Script> tag:
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs"></script>
And if you're using npm:
npm install @tensorflow/tfjs
So, after you've installed TensorFlow.js now you can build and train your ML models right in web browsers. This removes the need for server-side computing.
Building a Simple Machine Learning Model
To show how TensorFlow.js work, let's build a simple machine learning model, Linear Regression, in the browser. This model will take input from two variables, learn their relationship and, after that, predict a value based on them.
First, let's use tf.sequential() method to define the model with one layer:
const model = tf.sequential();
model.add(tf.layers.dense({units: 1, inputShape: [1]}));
Next, we'll specify the loss function and an optimizer to compile the model:
model.compile({loss: 'meanSquaredError', optimizer: 'sgd'});
Next, we'll create some data for model training:
const xs = tf.tensor2d([1, 2, 3, 4], [4, 1]);
const ys = tf.tensor2d([1, 3, 5, 7], [4, 1]);
Now, it's time to train the model with above created data:
model.fit(xs, ys, {epochs: 500}).then(() => {
model.predict(tf.tensor2d([5], [1, 1])).print();
});
In the above example code, the model was trained for a linear relationship between input xs and output ys. Once training is completed, it can now make predictions for unknown data. In this way model predicts the value for input 5.
Conclusion
Using TensorFlow.js, now integrating machine learning in web browsers become easier. Developers can maintain data integrity and privacy while running models directly on the browser, as they don't have to move on external servers. Not just image recognition or object detection, you can also do a wide range of tasks by using more advanced models. And that's only possible using TensorFlow.js.
394 views