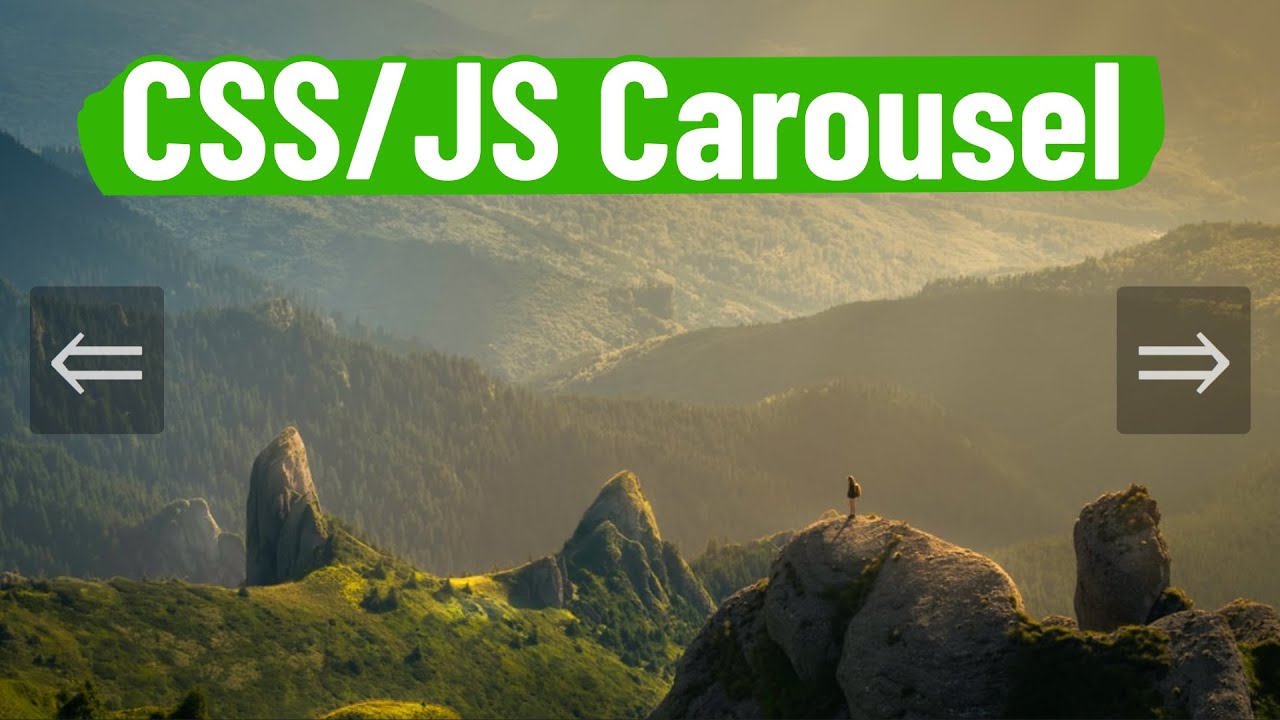
October 02, 2024
How to Build an Interactive Carousel with JavaScript and CSS Grid
Hi naive programmers! Do you want to give your users a single page to interact with a series of images and text, then Carousels are best for this purpose. Meanwhile, these are also popular in web design.
Now the question is how you can make one. No worries, I've come up with very easy steps to make responsive and engaging carousels using JavaScript and CSS grids. Moreover, these carousels that you'll learn to build can be navigated both manually and automatically.
Step 1: Setting Up the HTML Structure
Before getting started, you first need to build an HTML structure for setting up your interactive and engaging carousel; for this, you've to build an HTML container to place your carousel and its contents.
<div class="carousel">
<div class="carousel-item">Item 1</div>
<div class="carousel-item">Item 2</div>
<div class="carousel-item">Item 3</div>
</div>
<button id="prev">Previous</button>
<button id="next">Next</button>
For placing carousel items and buttons to move them left/right, the above code uses a container (.carousel). And (carousel-item) is used to hold carousel items.
Step 2: Designing the Layout with CSS Grid
Next, it's time for some designing. I'll use the CSS grid to design the carousel's layout. With a CSS grid, you can align your carousel items and navigation buttons. The grid format makes your carousel responsive and makes it able to work on different screen sizes. Let's see the code:
.carousel {
display: grid;
grid-template-columns: repeat(3, 100%);
overflow: hidden;
width: 100%;
}
.carousel-item {
display: flex;
justify-content: center;
align-items: center;
height: 300px;
background-color: #f0f0f0;
}
The above piece of code indicates (grid-template-columns: repeat(3, 100%)) to make sure each carousel item takes up full container space, which makes it responsive. And (overflow: hidden) hides each item that goes outside the container.
Step 3: JavaScript for Carousel Interaction
So, we've set up a container using HTML and designed the layout of the carousel using CSS; now it's time to make this carousel active, and that's possible using JavaScript. The carousel should respond to the navigation buttons designed and should be functional to move the carousel items back and forth.
const carousel = document.querySelector('.carousel');
let currentIndex = 0;
document.getElementById('next').addEventListener('click', () => {
currentIndex = (currentIndex + 1) % 3;
carousel.style.transform = `translateX(-${currentIndex * 100}%)`;
});
document.getElementById('prev').addEventListener('click', () => {
currentIndex = (currentIndex - 1 + 3) % 3;
carousel.style.transform = `translateX(-${currentIndex * 100}%)`;
});
The translateX property used above slides the items left/right. The currentIndex is responsible for visible items, while %3 loops back the carousel to the first item after the user has navigated to the last item.
Step 4: Adding Auto-Slide Feature
So, the above method of moving the Carousel items slide was manual, but if you want to make your carousel more interactive and engaging, then you can use the automatic sliding of items, too. Let's see the following code:
setInterval(() => {
currentIndex = (currentIndex + 1) % 3;
carousel.style.transform = `translateX(-${currentIndex * 100}%)`;
}, 3000);
Here, the setInterval function slides the carousel items forward after every 3 seconds. But if you want to make your users see the carousel items for long then you can stop the auto-slide when the user hovers on it.
carousel.addEventListener('mouseover', () => clearInterval(autoSlide));
carousel.addEventListener('mouseout', () => autoSlide = setInterval(autoSlideFn, 3000));
Conclusion
Making an engaging carousel using a perfect combo of JavaScript and CSS is a simple way to bring traffic to your website and make it more interactive. I hope by following the above steps you can make a perfect and responsive carousel without any hassle. You can also make it manual or auto-sliding as per your preferences.
Now you've learned the basics; it's your turn to experiment with the code and make your own customization to suit your website's design.
445 views