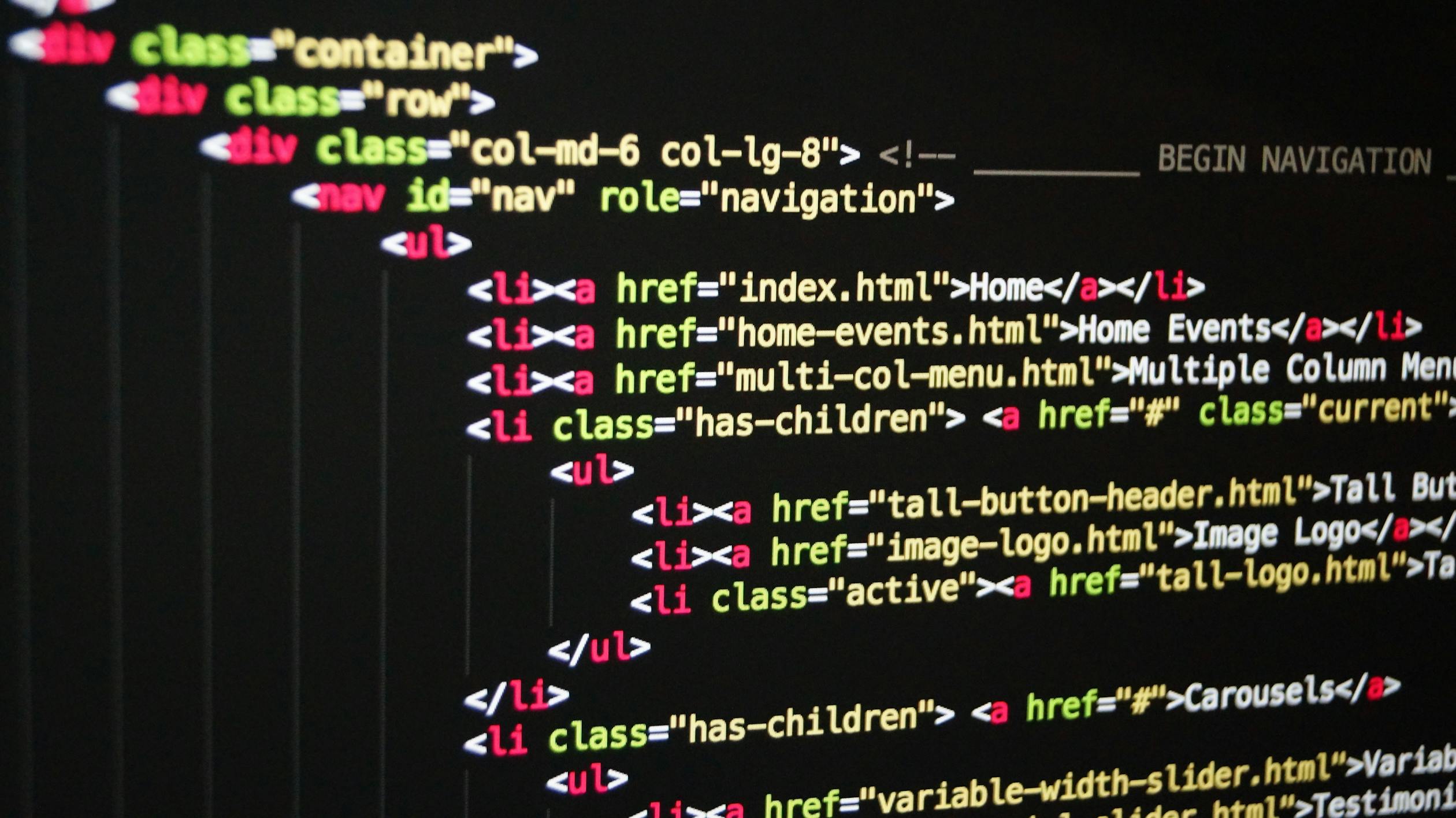
September 26, 2023
Building Secure Web Apps with JSON Web Tokens (JWT) Authentication
Security is a top concern in web development, and implementing robust authentication mechanisms is essential. In this article, I'll guide you through implementing JSON Web Token (JWT) authentication in a React.js application to secure your user data.
Introduction
JWT is a compact and self-contained token format that is widely used for securely transmitting information between parties. It's commonly used for authentication and authorization purposes in web applications.
Generating JWT Tokens in the Backend
To implement JWT authentication, you'll first need to generate JWT tokens on the server-side, typically during the login process. Here's a simplified example using Node.js and the jsonwebtoken
library:
const jwt = require('jsonwebtoken');
// Secret key for signing and verifying tokens
const secretKey = 'your-secret-key';
// Generate a JWT token
function generateToken(user) {
const payload = {
userId: user.id,
username: user.username,
};
// Token expiration time (e.g., 1 hour)
const expiresIn = '1h';
return jwt.sign(payload, secretKey, { expiresIn });
}
Authenticating Users in React.js
Once you have a JWT token, you can use it to authenticate users in your React.js application. Here's an example of how to store and use JWT tokens using the localStorage
API:
function Login() {
const handleLogin = async () => {
// Call your backend to authenticate the user
const response = await fetch('/login', {
method: 'POST',
// Include login credentials
body: JSON.stringify({ username, password }),
headers: {
'Content-Type': 'application/json',
},
});
if (response.ok) {
const data = await response.json();
// Store the JWT token in localStorage
localStorage.setItem('token', data.token);
} else {
// Handle login error
}
}
return (
//markup here
)
}
Securing API Requests
To secure API requests, you can include the JWT token in the Authorization
header of your HTTP requests. Here's an example using the fetch
API:
function fetchData() {
const token = localStorage.getItem('token');
if (!token) {
// Redirect to the login page or handle unauthorized access
return;
}
fetch('/api/data', {
headers: {
Authorization: `Bearer ${token}`,
},
})
.then((response) => {
if (response.ok) {
// Handle successful response
} else {
// Handle API error or unauthorized access
}
})
.catch((error) => {
// Handle network error
});
}
Implementing JWT authentication in a React.js application is a crucial step in ensuring the security of your user data. By generating tokens on the server-side and securely storing and transmitting them on the client-side, you can build a secure web application.
447 views