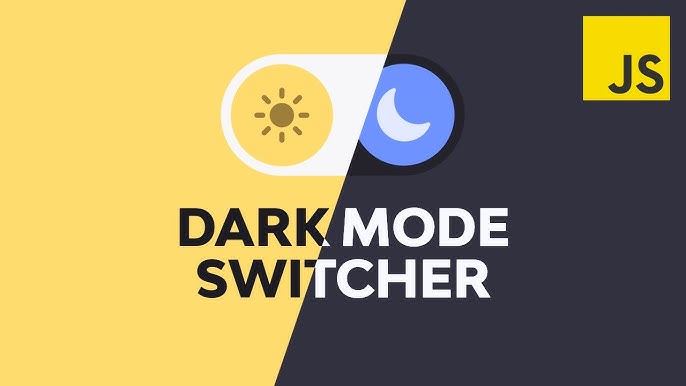
October 01, 2024
How to Build a Light/Dark Mode Toggle Using JavaScript and CSS Variables
With the growing popularity of dark mode, offering users the ability to toggle between light and dark themes has become an essential feature for web designers. This not only enhances the user experience but also provides more control over the website's appearance. In this article, we'll walk through how to create a light/dark mode toggle using JavaScript and CSS variables in just a few steps.
Step 1: Setting Up CSS Variables
To begin, we need to define our CSS variables for both light and dark themes. CSS variables make it easy to switch between styles by simply updating values. Start by declaring your default (light mode) variables within the :root selector.
:root {
--background-color: #ffffff;
--text-color: #000000;
}
These variables define the background colour and text colour for the light theme. Now, create a .dark-theme class to define the dark theme's styles:
.dark-theme {
--background-color: #000000;
--text-color: #ffffff;
}
This approach allows you to swap themes by simply applying or removing the .dark-theme class to your HTML elements, giving you full control over how the themes are applied throughout your site.
Step 2: Building the Toggle Button in HTML
Now that we have our CSS variables ready, the next step is to build a toggle button in HTML. A simple button will work fine for this tutorial:
<button id="theme-toggle">Toggle Light/Dark Mode</button>
This button will allow the user to switch between light and dark modes. We'll add functionality with JavaScript in the next step.
Step 3: JavaScript Logic for the Toggle
Now, let's write the JavaScript that toggles the dark mode. First, we'll need to select the toggle button and set up an event listener for clicks:
const toggleButton = document.getElementById('theme-toggle');
const body = document.body;
toggleButton.addEventListener('click', () => {
body.classList.toggle('dark-theme');
});
This script toggles the .dark-theme class on the body element each time the button is clicked. To ensure the user's theme preference is remembered on future visits, you can use localStorage:
if (localStorage.getItem('theme') === 'dark') {
body.classList.add('dark-theme');
}
toggleButton.addEventListener('click', () => {
body.classList.toggle('dark-theme');
let theme = body.classList.contains('dark-theme') ? 'dark' : 'light';
localStorage.setItem('theme', theme);
});
This code checks if a theme has been stored in localStorage and applies it when the page loads. Each time the button is clicked, the selected theme is saved for future visits.
Step 4: Testing and Debugging
Before deploying your light/dark mode toggle, it's important to test it across different browsers and devices to ensure compatibility. Check the responsiveness and consistency of the themes. If you encounter issues, verify that the CSS variables are being applied correctly and that the JavaScript event listener is functioning.
Common problems may include browser-specific quirks with CSS variables or JavaScript errors caused by incorrect selectors or logic.
Conclusion
Creating a light/dark mode toggle using JavaScript and CSS variables is a simple yet effective way to improve user experience on your website. By following the steps outlined above, you can easily implement this feature and allow your users to choose the theme they prefer. Adding such a toggle can significantly enhance engagement and accessibility on your site.
302 views