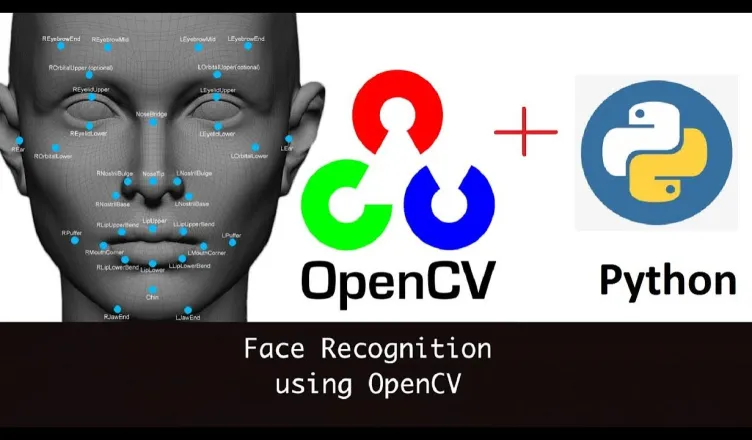
September 27, 2024
Python and OpenCV: Building a Real-Time Face Detection System
Have you ever thought about the mechanism behind recognizing your face when your phone unlocks? It's face detection that is ruling behind this. It's a main element of computer vision that makes our interaction with devices more engaging.
But again, the question is how all this is done and how you can create your own face detection system. This can be done using Python and OpenCV. Let me guide you through the steps of creating a seamless face detection system for yourself.
Setting Up Your Environment
But before getting started in development, first make sure your system environment is properly set up.
Installing Python and OpenCV:
First of all, install the latest version of Python on your system. Next, install the OpenCV library that will handle all your system's computer vision tasks. Run the following code in the Command Prompt:
pip install opencv-python
Other Libraries to Install:
So, once you've successfully installed Python and OpenCV, you can install some other libraries. Install cv2 and numpy (for array manipulation) if you're dealing with any pre-processing steps. Run this code to install them:
pip install numpy
Basic Overview of OpenCV's Face Detection Algorithm
So, you've installed all main elements; now let's get a brief overview of the main technology behind Face Detection. There's a core algorithm for OpenCV's face detection, the Haar Cascade Classifier. In 2001, this algorithm was introduced by Paul Viola and Michael Jones. This walks through a series of steps to detect if an image has a face in it.
This process is very complex at the backend, like analyzing the position and size of an image and also scanning the features like edge and texture that match a human face. The reason we use the Haar Cascade Classifier for face detection is its speed and efficiency.
There are more advanced and accurate face detection algorithms in the market, too, like Deep Neural Networks (DNNs). But as our face detection system is on a basic level so, I'll stick to the Haar Cascade Classifier. No doubt it's fast and efficient, too.
Writing the Code for Real-Time Face Detection
Loading the Pre-trained Cascade Classifier:
OpenCV contains many pre-trained classifiers to detect faces, which is very easy for beginners. To use one of these, first load the Haar Cascade XML file for front-end face detection.
import cv2
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
Note: If you didn't get an XML file while OpenCV's installation, make sure you download it from OpenCV's official repository.
Accessing the Webcam for Real-Time Video Capture:
As you know, when your phone unlocks by recognizing your face, it basically uses real-time face detection. So, for this purpose, you've to give access to your webcam feed. Use OpenCV's this code:
cap = cv2.VideoCapture(0)
This will open your device's main camera.
Detecting Faces in Real-Time:
Now you've set up everything, once the webcam is live, face detection will start with this piece of code:
while True:
ret, frame = cap.read() # Capture frame-by-frame
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) # Convert to grayscale
faces = face_cascade.detectMultiScale(gray, 1.3, 5) # Detect faces
for (x, y, w, h) in faces:
cv2.rectangle(frame, (x, y), (x+w, y+h), (255, 0, 0), 2) # Draw rectangle around faces
cv2.imshow('Face Detection', frame) # Display the resulting frame
if cv2.waitKey(1) & 0xFF == ord('q'): # Press 'q' to quit
break
cap.release()
cv2.destroyAllWindows()
This code captures your live webcam feed, changes each frame of feed in grayscale that makes processing easier, and then uses the detectMultiScale function to detect faces. There will be rectangular borders around the detected face.
Conclusion:
Now, your own face detection application is ready to use, using Python and OpenCV. This app was just a foundation of the computer vision field. You can get a hands-on grip on Python and the latest deep learning face detection algorithms to add more efficient features like eye or smile detection to your application. Happy Coding!
170 views