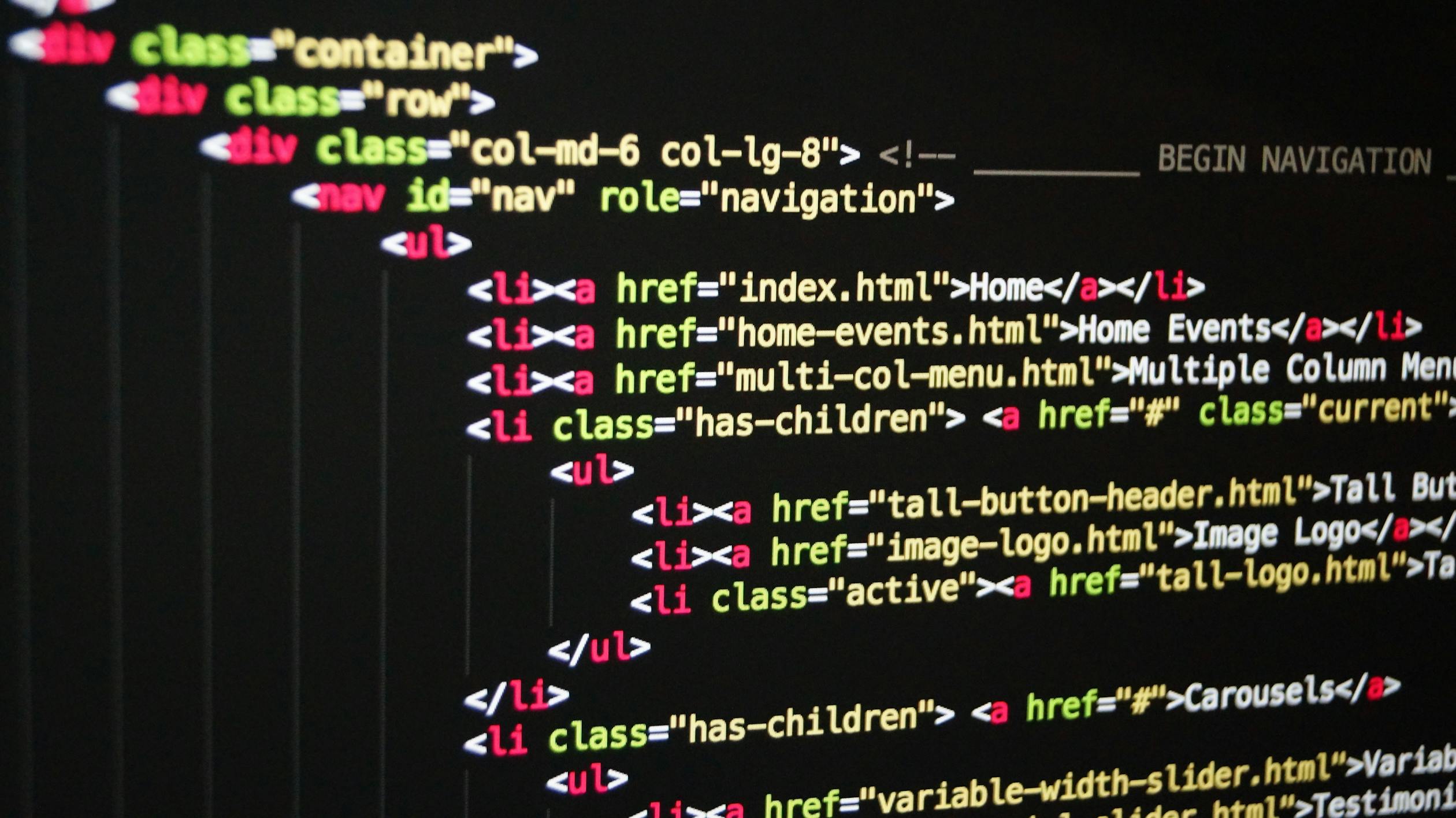
September 24, 2024
Automating Tasks with Python: Working with Files and Directories
Are you tired of spending time on tedious computer tasks? Is there a way to get rid of them? Imagine clicking one button to automate everything. Here comes Python! Python can automate file organization, folder creation, and document movement.
Besides saving time, automating these activities reduces errors. You can rename hundreds of files or organize your downloads folder with Python swiftly and precisely. This blog covers Python file and directory manipulation. You will learn to read, write, and manage files, create directories, and run basic scripts to simplify your life. Let me explore how Python can affect your file and folder management!
Why Automate File and Directory Tasks?
Python task automation is nice and useful! Let me explain:
Efficiency:
Automating file renaming and folder organization saves time. Python programs may repeat tasks in seconds instead of hours. You may concentrate on more essential things with increased productivity.
Accuracy:
Manual file management might lead to blunders like deleting or renaming files. Automation follows precise instructions every time, reducing mistakes and ensuring everything happens as planned.
Scalability:
Automation simplifies everything from handling a few files to thousands. Python scripts can handle huge file operations easily, making them perfect for large projects or busy students.
Automating file and directory activities saves time, improves accuracy, and reduces stress!
Getting Started with Python File Operations
Python simplifies computer file management. Reading from and writing to files are two of the most usual things you will do. What does it mean?
- Reading Files: Reading a file involves opening and seeing its contents. You may use Python's open() function. It takes a few lines of code to read a text file containing notes.
- Writing Files: Write to a file involves adding or changing data. Use write() to add text, data, or anything else. This helps preserve results and generate reports automatically.
This short example illustrates it:
# Reading from a file
with open('example.txt', 'r') as file: # 'r' stands for 'read mode'
content = file.read() # Reads the content of the file
print(content) # Prints whatâs inside the file
# Writing to a file
with open('example.txt', 'w') as file: # 'w' stands for 'write mode'
file.write('Hello, this is a new line!') # Writes a new line in the file
Starting with example.txt, the code displays everything on the screen. It then writes a new line of text to the same file (or creates it if it does not exist) in the second portion. You can automate note updating, report creation, and data tracking with these basic functions.
Working with Directories in Python
Organizing files on your computer is made easier using directories, which are also called folders. Python makes it easy to create, delete, and navigate these folders.
- Creating Directories: Python's os.mkdir() function creates new folders. Like clicking âNew Folderâ but quicker, particularly for many folders.
- Deleting Directories: Use os.rmdir() to delete a folder. Note that this feature only works in empty folders.
- Navigating Directories: Use os.chdir() to switch directories. Change your location to work in another folder using this command. To view all files in a directory, use os.listdir().
A basic code example is following:
import os
# Creating a new directory
os.mkdir('NewFolder') # Creates a folder named 'NewFolder'
# Navigating to the new directory
os.chdir('NewFolder') # Changes the current directory to 'NewFolder'
# Listing files in the current directory
print(os.listdir()) # Prints the list of files and folders in 'NewFolder'
# Going back to the previous directory
os.chdir('..') # '..' means "go back one level up"
# Deleting the directory
os.rmdir('NewFolder') # Removes 'NewFolder' (only if it's empty)
Automating Common File Tasks
Python automatically renames, moves, and organizes files. This is great if you have many files and wish to organize them automatically.
- Renaming and Moving Files: Os.rename() and shutil.move() modify file names and locations in Python.
- Organizing Files by Type: Python can organize files by type, such as photos in one folder and papers in another, if your directories are messy.
- This little code organizes files automatically:
import os
import shutil
# Define the folder to organize
folder_path = 'Downloads' # Replace with the folder you want to organize
# Create subfolders for each file type
folders = {
'Images': ['.jpg', '.png', '.gif'],
'Documents': ['.pdf', '.docx', '.txt'],
'Videos': ['.mp4', '.mov'],
}
# Loop through each file in the main folder
for filename in os.listdir(folder_path):
file_path = os.path.join(folder_path, filename)
# Skip if it's a directory
if os.path.isdir(file_path):
continue
# Check each file type and move it to the appropriate folder
for folder, extensions in folders.items():
if any(filename.lower().endswith(ext) for ext in extensions):
# Create the folder if it doesn't exist
new_folder_path = os.path.join(folder_path, folder)
os.makedirs(new_folder_path, exist_ok=True)
# Move the file to the new folder
shutil.move(file_path, new_folder_path)
print(f"Moved {filename} to {folder}")
break
Handling Errors Gracefully
File and directory operations in Python do not always go as expected. This is where error handling helps! Try-except blocks can handle these problems without crashing your project.
Error Handling with try-except:
Code that might trigger an error goes in the try block. The except portion detects errors so you can handle them instead of letting the code fail.
Common Errors:
- FileNotFoundError: This error occurs when you access a missing file.
- PermissionError: Not having permission to read, write, or remove a file or folder causes this.
Hereâs an example:
import os
try:
# Trying to read a non-existent file
with open('nonexistent.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print("Error: The file was not found. Please check the file name.")
try:
# Trying to delete a directory without permission
os.rmdir('ProtectedFolder')
except PermissionError:
print("Error: You don't have permission to delete this folder.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Conclusion
Python simplifies file and directory administration, automating routine processes, reducing mistakes, and handling large-scale operations. Learn fundamental Python file operations, directory management, and automation scripts to save time and boost productivity. Implement these amazing features now to improve your productivity!
152 views