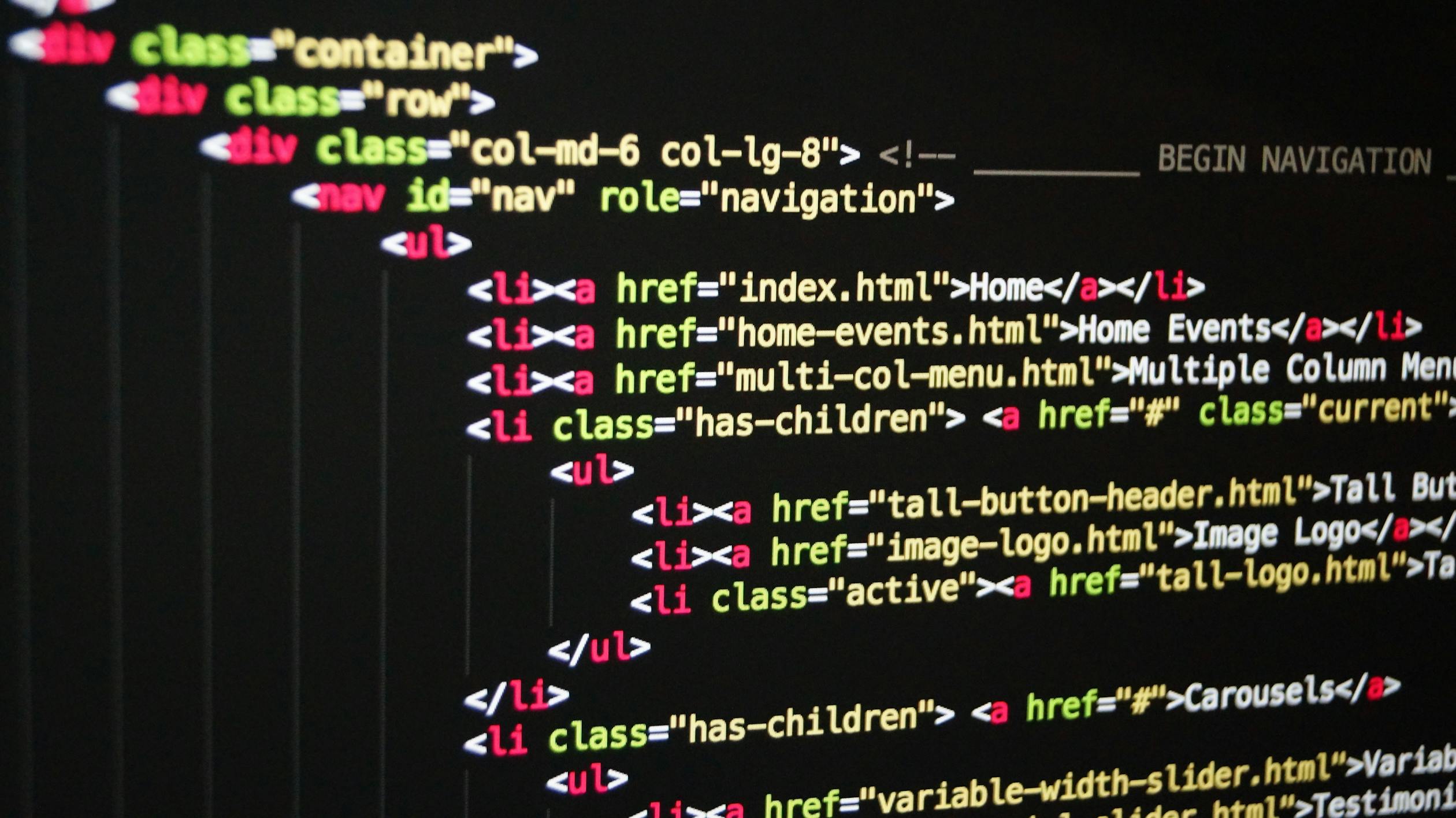
September 26, 2023
Optimizing React.js Performance for a Seamless User Experience
As a front-end developer, I understand the importance of delivering a fast and responsive user experience. In this article, I'll share my insights and best practices for optimizing React.js applications to ensure your users have a seamless experience, regardless of the devices and browsers they use.
Introduction
React.js is a popular JavaScript library for building user interfaces, but like any technology, it can face performance challenges, especially as your application grows. To address these challenges, you need to employ various optimization techniques.
Profiling and Identifying Performance Bottlenecks
Before you can optimize your React.js application, you need to identify performance bottlenecks. React provides a built-in tool called the React DevTools Profiler, which allows you to visualize and analyze component rendering times. Here's how you can use it:
import React, { Profiler } from 'react';
function MyComponent() {
// TODO component logic here
}
function onRenderCallback(
id,
phase,
actualDuration,
baseDuration,
startTime,
commitTime,
interactions
) {
// Log performance data
console.log(`Rendered component: ${id}`);
console.log(`Actual duration: ${actualDuration} ms`);
}
function App() {
return (
<Profiler id="my-component" onRender={onRenderCallback}>
<MyComponent />'
</Profiler>
);
}
Code Splitting
Code splitting is a technique that allows you to split your JavaScript code into smaller chunks, loading only the necessary code for a specific route or feature. This can significantly reduce the initial load time of your application. React provides a built-in way to achieve code splitting using React.lazy and Suspense:
import React, { lazy, Suspense } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
Optimizing React.js performance is crucial for providing a smooth user experience. By profiling, code splitting, and using memoization, you can address performance bottlenecks and ensure your React applications run efficiently.
375 views