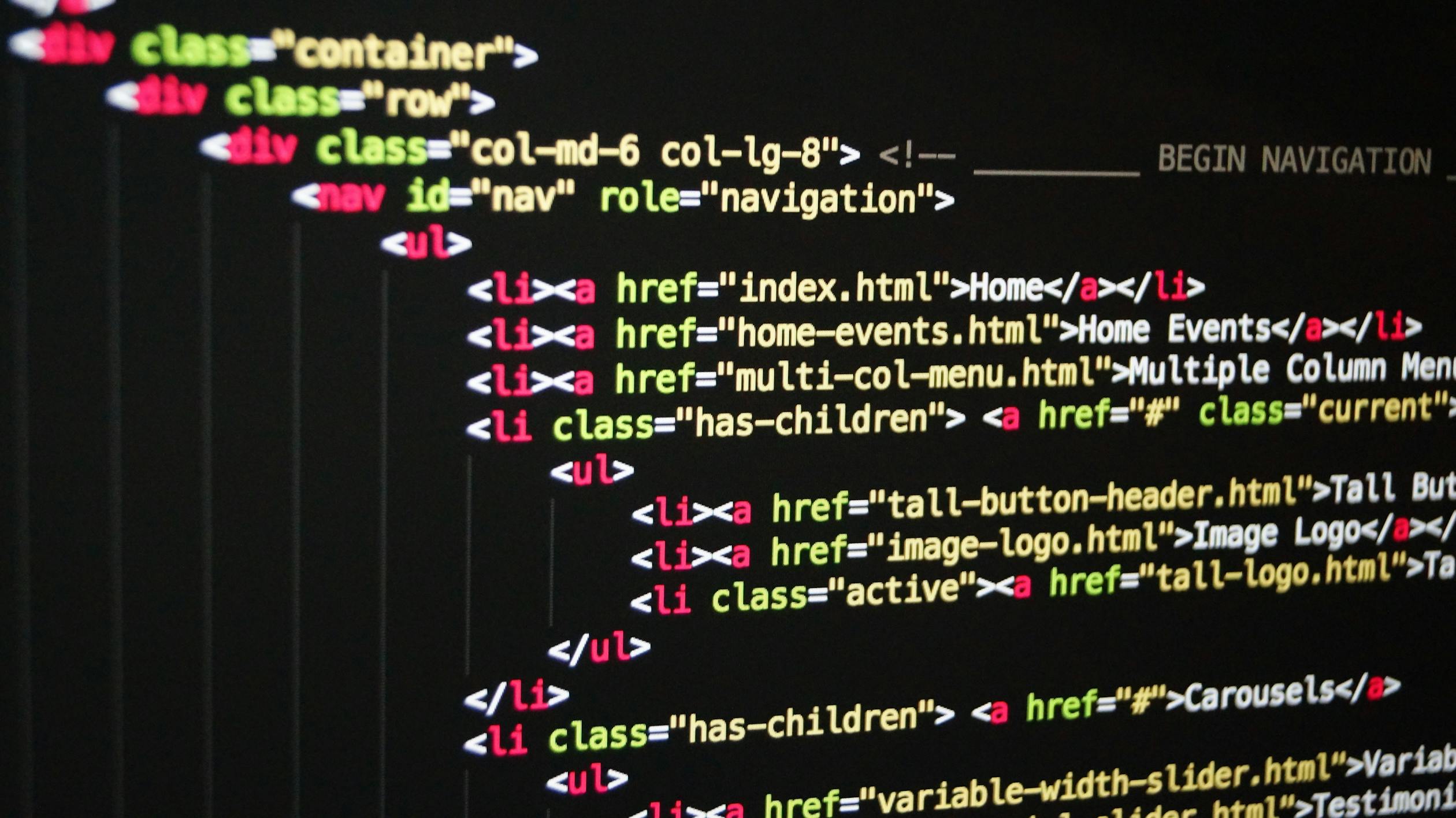
September 17, 2024
Python for Audio Processing: Building Your Music Visualizer
One of the most fascinating mixes of music and technology is music visualizers. These apps make music more exciting by turning sound into moving images. Python is simple to learn and includes robust audio and graphics packages, making it ideal for visualizers. This post will show you how to construct a basic music visualizer using Python to collect sound and create stunning visuals.
Tools and Libraries You'll Need
You will need a few Python libraries to help you record sound, process audio, and make graphics for your music visualizer:
1. PyAudio:
This library collects real-time microphone or computer audio. It lets your program listen to sound and understand it in real-time.
Installation: pip install pyaudio
2. NumPy:
NumPy handles huge data sets like audio waves. It excels at math tasks like audio frequency analysis.
Installation: pip install numpy
3. Matplotlib:
Visuals come from this library. It can make music-responsive graphs, waveforms, and other shapes.
Installation: pip install matplotlib
4. FFmpeg (optional):
FFmpeg can record and convert visualizers to videos. Also, it can record both the sound and the images at the same time.
Capturing Audio Input Using PyAudio
Set up PyAudio to record microphone sounds. This Python library offers real-time sound listening.
So, let's start by doing this:
import pyaudio
p = pyaudio.PyAudio()
# Open stream to capture audio
stream = p.open(format=pyaudio.paInt16, channels=1, rate=44100, input=True, frames_per_buffer=1024)
# Read audio data
data = stream.read(1024)
This code opens an audio stream for microphone listening. To make images, we can use the audio data that the program gets in small chunks. This is how Python captures real-time sound!
Analyzing Audio Frequencies with Fast Fourier Transform (FFT)
Fast Fourier Transform (FFT) math breaks down sound into frequency components. This helps us identify auditory notes (frequencies). Analyzing these frequencies lets us match sound to images.
In Python, use NumPy to apply FFT:
import numpy as np
# Convert audio data to numpy array
audio_data = np.frombuffer(data, dtype=np.int16)
# Apply FFT to extract frequency components
frequencies = np.fft.fft(audio_data)
# Get the magnitude (amplitude) of the frequencies
amplitude = np.abs(frequencies)
First, we turn the audio data into a NumPy array. FFT splits the audio stream into its frequency components. Amplitude shows how powerful each frequency is, so we can make visualizations that fluctuate with the music.
If you know how sound waves and frequencies work together, you can make images that "dance" to the beat of the music.
Creating the Visual Representation
Now that we know the sound levels, we can turn them into pictures! We can use Matplotlib to show the frequency spectrum (how strong each sound frequency is) as waves or bar charts that move along with the music.
Here's some simple code that will make a bar chart that moves to the audio:
import matplotlib.pyplot as plt
# Plot the frequency spectrum
plt.bar(range(len(amplitude)), amplitude)
plt.xlabel('Frequency')
plt.ylabel('Amplitude')
plt.title('Real-time Audio Visualization')
plt.show()
Each bar in this bar chart represents a sound frequency, and its height indicates its amplitude (loudness).
Change bar colours, shapes, and animations to modify visually. A rolling average of amplitudes may smooth bar response. Using waves, rings, or other shapes could also help you make visual effects that match different sound frequencies.
Enhancing Your Visualizer
Interactive graphics, such as bars that move and change colour when music plays, can make your visualizer more interesting. You might animate the bars to expand, decrease, or change colour as frequency or loudness rises. You may also display a waveform and frequency bars simultaneously.
Use gradual transitions or audio averaging to smooth visuals. Limit visual components or use efficient coding approaches such as updating just regularly changing graphics for optimal efficiency.
Exporting the Visualizer as a Video
This library can help you save your visualizer as a video. Record your visuals using screen recording software. After that, use FFmpeg to change the recording into a video file:
ffmpeg -framerate 30 -i input.mp4 -c:v libx264 -r 30 -pix_fmt yuv420p output.mp4
This command turns the file you saved into a video that plays smoothly.
Conclusion
To create a music visualizer using Python, we captured audio, analyzed frequencies, and created visualizations. Try multiple visual and audio techniques to make your project stand out. Expand your skill set with extra tools and libraries for complex projects. Have fun coding!
410 views