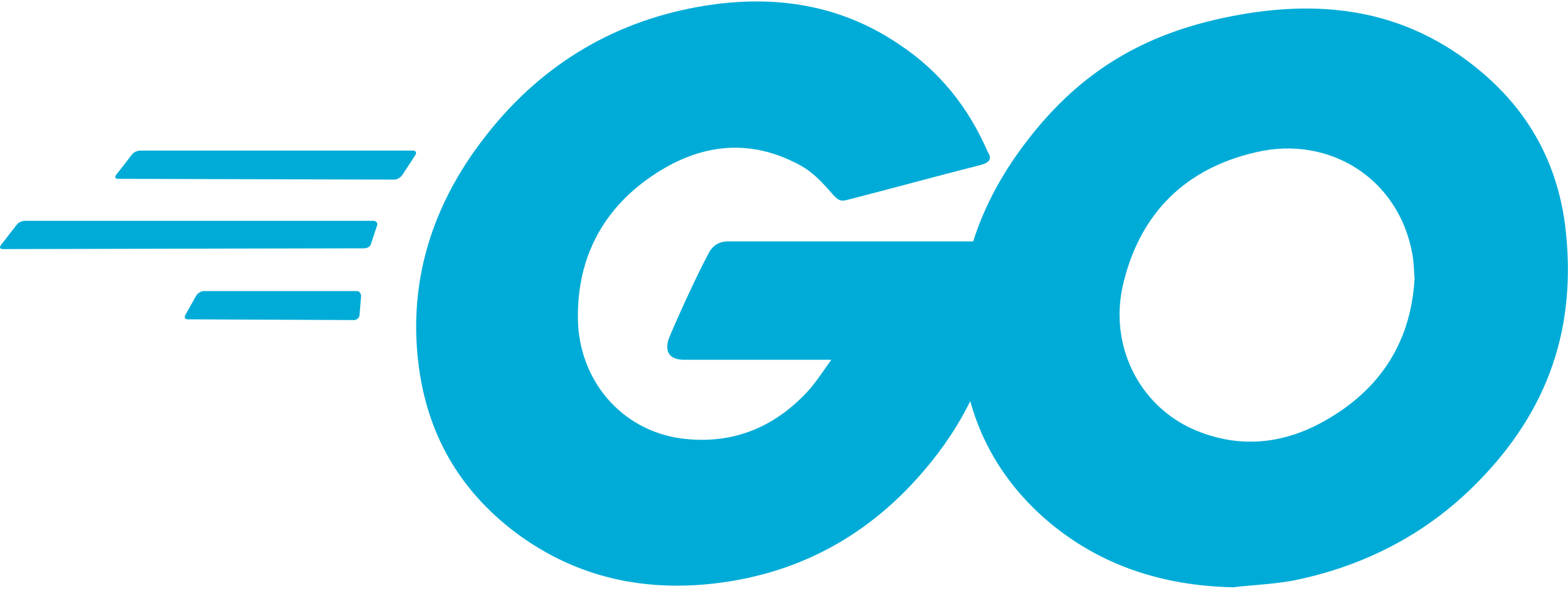
Structuring a Go project effectively is crucial for maintaining scalability, readability, and ease of collaboration. Whether you're developing a simple application or a complex system, following a well-defined project structure can make your development process more organized and efficient. In this guide, we'll explore best practices for structuring your Go project.
1. The Standard Project Layout
The Go community often adheres to a common project layout which helps in maintaining consistency across different projects. Here's a basic structure:
/myproject
|-- cmd/
| |--myapp/
| | |-- main.go
|-- pkg/
| |-- mylib/
| | |-- mylib.go
| | |-- mylib_test.go
|internal/
| |-- app/
| |-- app.go
| |-- app_test.go
|-- api/
| |-- v1/
| |-- api.go
| |-- api_test.go
|-- configs/
| |-- config.yaml
|-- web/
| |-- static/
| |-- templates/
|-- scripts/
| |-- build.sh
|-- docs/
| |-- README.md
|-- .gitignore
|-- go.mod
|-- main.go
Key Directories and Their Purposes
cmd/: This directory contains the entry points for your applications. Each subdirectory under cmd
represents a separate executable. For instance, cmd/myapp
contains the main function for the myapp
executable.
pkg/: This directory holds libraries and packages that can be used by your project and potentially by external projects. These packages are meant to be reusable and are intended for public use.
internal/: Code under internal
is meant to be private and accessible only within your project. This is a great place for code that you don't want others to import and use.
api/: This directory is often used to define your API schemas, such as protocol buffers, GraphQL schemas, or OpenAPI/Swagger specs.
configs/: Configuration files, such as YAML, JSON, or TOML files, are stored here.
web/: This directory contains web-related resources such as HTML templates, static files, and frontend assets.
scripts/: Useful scripts for building, packaging, and other automated tasks are kept here.
docs/: Documentation for your project. This can include README files, design documents, or API documentation.
main.go: The primary entry point for your application, typically when you have a single application in the project.
2. Go Modules
Since Go 1.11, Go modules have been the standard for dependency management. A go.mod
file at the root of your project specifies your module's dependencies. Run go mod init myproject
to create this file if you haven't already. Use go mod tidy
to add missing and remove unused dependencies.
3. Packages and Naming Conventions
Package Naming: Package names should be short, concise, and lowercase. Avoid underscores and camelCase. The package name should reflect its functionality. For example, http
, math
, time
.
File Naming: Use descriptive names that give a clear idea of what the file contains. For instance, user_service.go
for a file handling user-related logic.
4. Internal Packages
Using the internal
directory allows you to create packages that are not accessible to other projects. This is useful for encapsulating code that should not be exposed to external use. For example, if you have utility functions or core business logic that should only be used within your project, place them in internal
.
5. Configuration Management
Storing configuration files in a dedicated configs
directory helps in managing different environments (development, staging, production). Use a consistent format like YAML or JSON and consider using a package like viper
for managing configurations.
6. Separation of Concerns
Organize your code in a way that separates different concerns. For example, keep your HTTP handlers, business logic, and database interactions in separate packages. This makes your codebase more modular and easier to maintain.
7. Testing
Place your test files in the same package as the code they test, and suffix them with _test.go
. For instance, tests for mylib.go
should be in mylib_test.go
. This keeps your tests close to the code, making it easier to find and maintain them.
8. Documentation
Maintaining good documentation is essential. Use docs/
for extensive documentation, and write clear, concise comments in your code. Tools like godoc
can generate documentation from your comments.
Conclusion
Following these best practices will help you maintain a clean and efficient Go project structure. This not only makes your codebase easier to navigate and maintain but also makes it more approachable for new contributors. Remember that while these guidelines are helpful, the most important thing is to choose a structure that works best for your specific project and team.
1.3k views