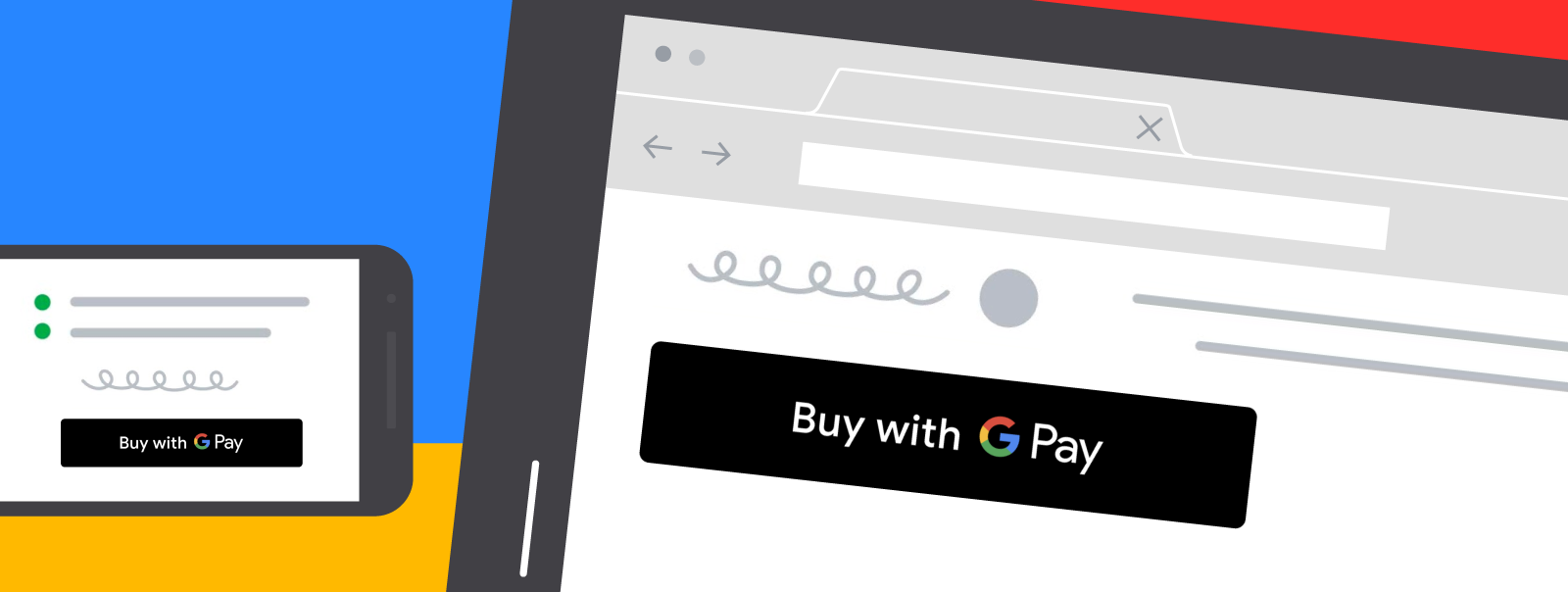
June 05, 2024
Implementing Google Payment in React Native
In today's digital world, integrating a seamless payment gateway is crucial for any app handling transactions. Google Pay is a popular choice due to its wide user base and secure infrastructure. In this blog, weâll walk you through the steps to implement Google Pay in a React Native application.
Prerequisites
Before we start, ensure you have the following:
- React Native development environment: You should have Node.js, npm, React Native CLI, and Android Studio installed.
- Google Play Console Account: To use Google Pay, you need to register your application on the Google Play Console.
Step 1: Install Required Packages
First, we need to install the necessary packages for Google Pay. React Native doesnât have built-in support for Google Pay, so weâll use the react-native-google-pay package.
Run the following command to install it:
npm install react-native-google-pay
Link the package to your React Native project:
npx react-native link react-native-google-pay
For newer versions of React Native (0.60+), auto-linking should handle this, but manual linking is still required for native modules.
Step 2: Configure Android Settings
Modify android/app/build.gradle
Add Google Play services to your project by including it in your android/app/build.gradle file:
dependencies {
implementation 'com.google.android.gms:play-services-wallet:18.1.3'
}
Update Android Manifest
Open android/app/src/main/AndroidManifest.xml and add the following permissions and meta-data:
<uses-permission android:name="android.permission.INTERNET" />
<application ... >
...
<meta-data
android:name="com.google.android.gms.wallet.api.enabled"
android:value="true" />
</application>
Step 3: Set Up Google Pay API
To use Google Pay, you need to configure a payment profile in the Google Pay API. Hereâs a basic configuration:
const allowedCardNetworks = ["AMEX", "DISCOVER", "JCB", "MASTERCARD", "VISA"];
const allowedAuthMethods = ["PAN_ONLY", "CRYPTOGRAM_3DS"];
const baseRequest = {
apiVersion: 2,
apiVersionMinor: 0,
};
const tokenizationSpecification = {
type: 'PAYMENT_GATEWAY',
parameters: {
gateway: 'example', // Change 'example' to your payment gateway
gatewayMerchantId: 'exampleGatewayMerchantId', // Your gateway merchant ID
},
};
const cardPaymentMethod = {
type: 'CARD',
parameters: {
allowedAuthMethods,
allowedCardNetworks,
},
tokenizationSpecification,
};
const googlePayRequestData = {
...baseRequest,
allowedPaymentMethods: [cardPaymentMethod],
transactionInfo: {
totalPriceStatus: 'FINAL',
totalPrice: '10.00',
currencyCode: 'USD',
},
merchantInfo: {
merchantName: 'Example Merchant', // Your merchant name
},
};
Step 4: Implement Google Pay in React Native
Now, letâs implement the Google Pay button and handle the payment process.
Import and Initialize Google Pay
In your React Native component, import the necessary modules and initialize Google Pay:
import React from 'react';
import { View, Button, Alert } from 'react-native';
import GooglePay from 'react-native-google-pay';
GooglePay.setEnvironment(GooglePay.ENVIRONMENT_TEST);
const allowedPaymentNetworks = ['VISA', 'MASTERCARD'];
const allowedPaymentMethods = ['PAN_ONLY', 'CRYPTOGRAM_3DS'];
const requestData = {
cardPaymentMethod: {
tokenizationSpecification: {
type: 'PAYMENT_GATEWAY',
gateway: 'example',
gatewayMerchantId: 'exampleGatewayMerchantId',
},
allowedCardNetworks: allowedPaymentNetworks,
allowedAuthMethods: allowedPaymentMethods,
},
transactionInfo: {
totalPriceStatus: 'FINAL',
totalPrice: '10.00',
currencyCode: 'USD',
},
merchantInfo: {
merchantName: 'Example Merchant',
},
};
Add Google Pay Button and Handle Payment
Add a button in your component to initiate the Google Pay payment process:
const GooglePayButton = () => {
const onPress = () => {
GooglePay.isReadyToPay(allowedPaymentNetworks, allowedPaymentMethods)
.then((ready) => {
if (ready) {
GooglePay.requestPayment(requestData)
.then((token) => {
// Send a token to your backend to process the payment
Alert.alert('Payment Success', `Token: ${token}`);
})
.catch((error) => Alert.alert('Payment Error', error.message));
} else {
Alert.alert('Google Pay is not available on this device.');
}
})
.catch((error) => Alert.alert('Error', error.message));
};
return (
<View>
<Button title="Pay with Google Pay" onPress={onPress} />
</View>
);
};
export default GooglePayButton;
Step 5: Testing
Before going live, test the Google Pay integration thoroughly. Use Googleâs test cards and ensure transactions are processed correctly.
- Run your app: Use npx react-native run-android to start the application on an emulator or a physical device.
- Test the payment flow: Ensure the button appears and that the payment process completes successfully using Googleâs test environment.
Conclusion
Implementing Google Pay in your React Native app enhances the user experience by providing a fast and secure payment method. By following this guide, you can integrate Google Pay seamlessly into your app. Always remember to test thoroughly and comply with Googleâs policies and guidelines to ensure a smooth transaction process for your users.
405 views