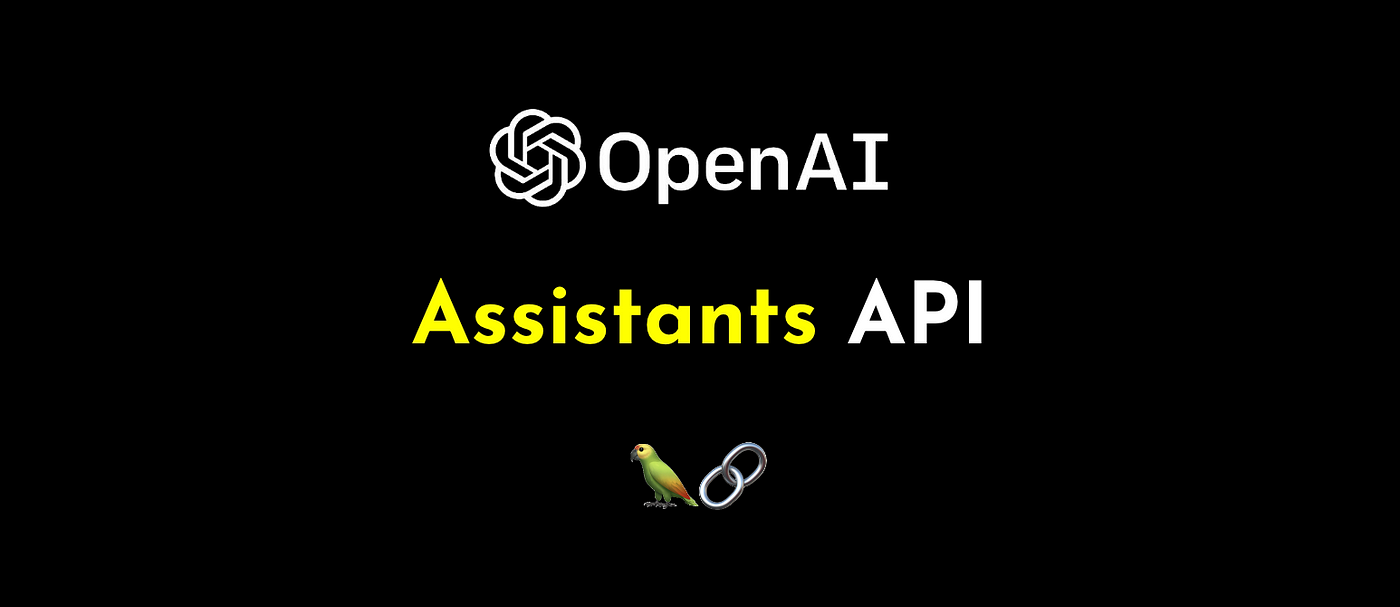
May 26, 2024
Building A Next.js Applications with OpenAI Assistant
Introduction
Next.js, a popular React framework, offers developers the ability to build fast and efficient web applications with ease. When combined with the power of OpenAI's assistant capabilities, you can create smarter, more interactive applications that enhance user experience. In this blog post, we'll explore how to integrate OpenAI's assistant into a Next.js application, providing practical examples and best practices.
Getting Started
Prerequisites
Before you begin, ensure you have the following:
Node.js installed on your machine.
Next.js set up in your project. If you haven't set it up yet, you can create a new Next.js app using:
npx create-next-app@latest
OpenAI API Key. You can obtain this by signing up on the OpenAI website.
Installing Dependencies
First, install the required dependencies. You'll need the openai package to interact with the OpenAI API:
npm install openai
Setting Up the OpenAI Client
Create a new file, lib/openai.js, in your Next.js project to set up the OpenAI client:
import { Configuration, OpenAIApi } from 'openai';
const configuration = new Configuration({
apiKey: process.env.OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
export default openai;
Make sure to add your OpenAI API key to your environment variables. Create a .env.local file in the root of your project:
OPENAI_API_KEY=your_openai_api_key
Creating an API Route
Next.js makes it easy to create API routes. We'll create an endpoint that interacts with the OpenAI API. Create a new file, pages/api/chat.js:
import openai from '../../lib/openai';
export default async function handler(req, res) {
if (req.method !== 'POST') {
return res.status(405).json({ message: 'Only POST requests are allowed' });
}
const { message } = req.body;
if (!message) {
return res.status(400).json({ message: 'Message is required' });
}
try {
const response = await openai.createChatCompletion({
model: 'gpt-4',
messages: [{ role: 'user', content: message }],
});
const assistantMessage = response.data.choices[0].message.content;
res.status(200).json({ message: assistantMessage });
} catch (error) {
res.status(500).json({ message: 'Something went wrong', error: error.message });
}
}
Building the Frontend
Now, let's build a simple chat interface where users can interact with the OpenAI assistant. Update pages/index.js with the following code:
import { useState } from 'react';
export default function Home() {
const [message, setMessage] = useState('');
const [response, setResponse] = useState(null);
const [loading, setLoading] = useState(false);
const handleSubmit = async (e) => {
e.preventDefault();
setLoading(true);
try {
const res = await fetch('/api/chat', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ message }),
});
const data = await res.json();
setResponse(data.message);
} catch (error) {
console.error('Error:', error);
setResponse('Sorry, something went wrong.');
} finally {
setLoading(false);
}
};
return (
<div style={{ padding: '2rem' }}>
<h1>Chat with OpenAI Assistant</h1>
<form onSubmit={handleSubmit}>
<textarea
value={message}
onChange={(e) => setMessage(e.target.value)}
rows="4"
cols="50"
placeholder="Type your message here"
/>
<br />
<button type="submit" disabled={loading}>
{loading ? 'Loading...' : 'Send'}
</button>
</form>
{response && (
<div style={{ marginTop: '1rem' }}>
<h2>Assistant's Response:</h2>
<p>{response}</p>
</div>
)}
</div>
);
}
Running Your Application
To see your OpenAI-powered Next.js application in action, run:
npm run dev
Navigate to http://localhost:3000 in your browser, and you should see your chat interface. Type a message and see how the OpenAI assistant responds.
Conclusion
Integrating OpenAI's assistant with a Next.js application can significantly enhance the interactivity and intelligence of your web app. This blog covered the basics of setting up the OpenAI client, creating an API route, and building a frontend interface. With this foundation, you can expand your application to include more advanced features and create a truly dynamic user experience.
872 views