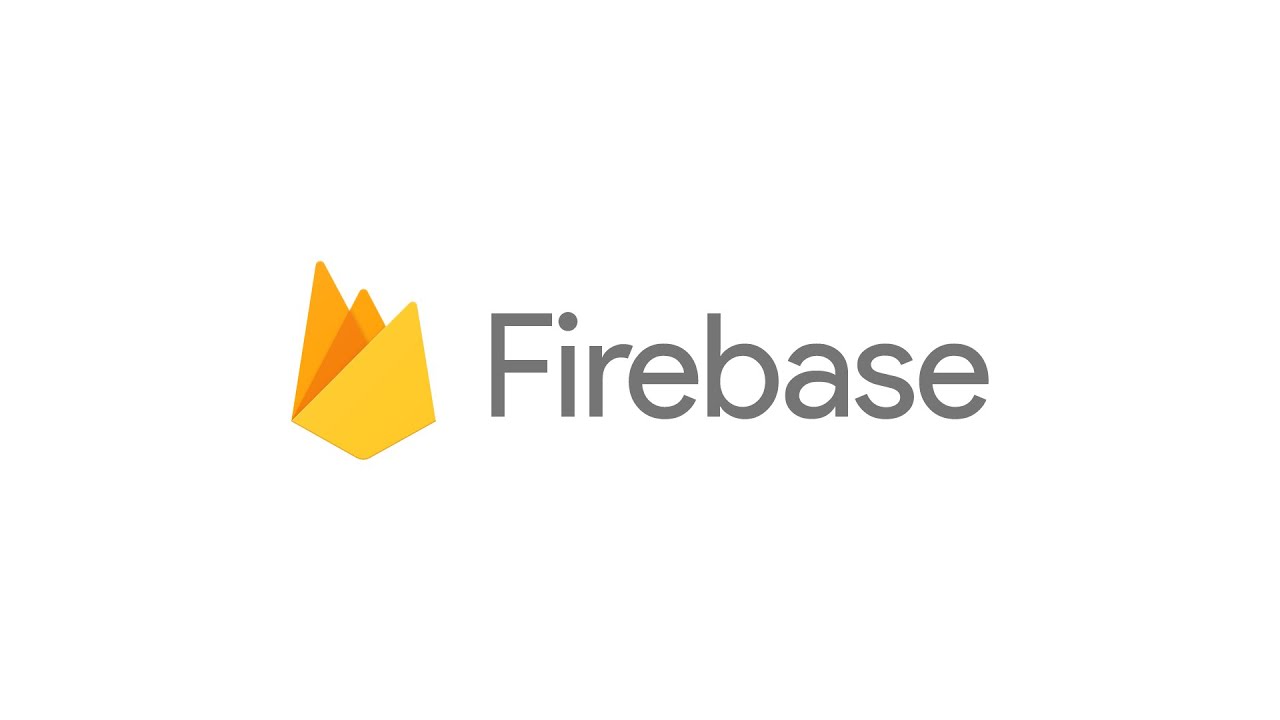
In the evolving landscape of app development, combining the power of SwiftUI for building modern user interfaces with Firebaseâs robust backend services can result in highly scalable and feature-rich applications. Whether you're a seasoned developer or just starting, integrating Firebase with SwiftUI can simplify backend management and accelerate your development process. This blog post will walk you through the essential steps and provide insights on effectively using Firebase in your SwiftUI projects.
Why Use Firebase with SwiftUI?
Benefits of Firebase:
- Real-time Database: Synchronize data across all clients in real-time.
- Authentication: Simplify user authentication with various providers.
- Cloud Firestore: Store and sync data at global scale.
- Analytics: Gain insights into user engagement and app performance.
- Crashlytics: Track, prioritize, and fix stability issues.
- Cloud Functions: Run backend code in response to events triggered by Firebase features.
Advantages of SwiftUI:
- Declarative Syntax: Build user interfaces by declaring what you want.
- Live Previews: See the immediate effect of code changes.
- Seamless Integration: Works perfectly with existing UIKit components.
Combining these two technologies can significantly reduce development time and improve app functionality.
Getting Started
Step 1: Create a Firebase Project
- Go to the Firebase Console.
- Click on âAdd Projectâ and follow the prompts to set up your new project.
- Once created, navigate to the Project Overview page.
Step 2: Add Firebase to Your iOS App
- Click on the iOS icon to add an iOS app.
- Register your app by entering the iOS bundle ID.
- Download the GoogleService-Info.plist file and add it to your Xcode project.
- Add the Firebase SDK to your project by updating your Podfile:
platform :ios, '13.0'
use_frameworks!
target 'YourAppName' do
pod 'Firebase/Analytics'
pod 'Firebase/Auth'
pod 'Firebase/Firestore'
# Add other Firebase pods if needed
end
- Run pod install to install the dependencies.
Step 3: Initialize Firebase in Your SwiftUI App
Open your AppDelegate.swift and configure Firebase:
import UIKit
import Firebase
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
FirebaseApp.configure()
return true
}
}
Using Firebase in SwiftUI
Authentication Example
Setting Up Authentication View
Create a new SwiftUI view for user authentication:
import SwiftUI
import FirebaseAuth
struct AuthView: View {
@State private var email = ""
@State private var password = ""
@State private var isAuthenticated = false
@State private var errorMessage = ""
var body: some View {
VStack {
TextField("Email", text: $email)
.padding()
.background(Color(.secondarySystemBackground))
.autocapitalization(.none)
SecureField("Password", text: $password)
.padding()
.background(Color(.secondarySystemBackground))
Button(action: signIn) {
Text("Sign In")
.foregroundColor(.white)
.padding()
.background(Color.blue)
.cornerRadius(8)
}
if !errorMessage.isEmpty {
Text(errorMessage)
.foregroundColor(.red)
.padding()
}
}
.padding()
}
private func signIn() {
Auth.auth().signIn(withEmail: email, password: password) { authResult, error in
if let error = error {
errorMessage = error.localizedDescription
} else {
isAuthenticated = true
}
}
}
}
Using Authentication State
In your main app file, check for authentication state and navigate accordingly:
import SwiftUI
import Firebase
@main
struct YourApp: App {
@UIApplicationDelegateAdaptor(AppDelegate.self) var delegate
var body: some Scene {
WindowGroup {
if Auth.auth().currentUser != nil {
ContentView()
} else {
AuthView()
}
}
}
}
Firestore Example
Setting Up Firestore
Create a Firestore database in the Firebase console and add some test data. Next, integrate Firestore in your SwiftUI view.
Fetching Data
Create a view to display Firestore data:
import SwiftUI
import FirebaseFirestore
import FirebaseFirestoreSwift
struct ContentView: View {
@ObservedObject private var viewModel = ItemsViewModel()
var body: some View {
NavigationView {
List(viewModel.items) { item in
Text(item.name)
}
.navigationTitle("Items")
.onAppear() {
viewModel.fetchItems()
}
}
}
}
class ItemsViewModel: ObservableObject {
@Published var items = [Item]()
private var db = Firestore.firestore()
func fetchItems() {
db.collection("items").addSnapshotListener { (querySnapshot, error) in
guard let documents = querySnapshot?.documents else {
print("No documents")
return
}
self.items = documents.compactMap { queryDocumentSnapshot -> Item? in
return try? queryDocumentSnapshot.data(as: Item.self)
}
}
}
}
struct Item: Identifiable, Codable {
@DocumentID var id: String?
var name: String
}
Conclusion
Integrating Firebase with SwiftUI allows developers to leverage powerful backend services while building sleek and responsive user interfaces. By following this guide, you should be able to set up Firebase in your SwiftUI project and start using features like Authentication and Firestore. Firebaseâs extensive suite of tools paired with SwiftUIâs modern approach to UI development creates a productive environment for building exceptional apps.
628 views