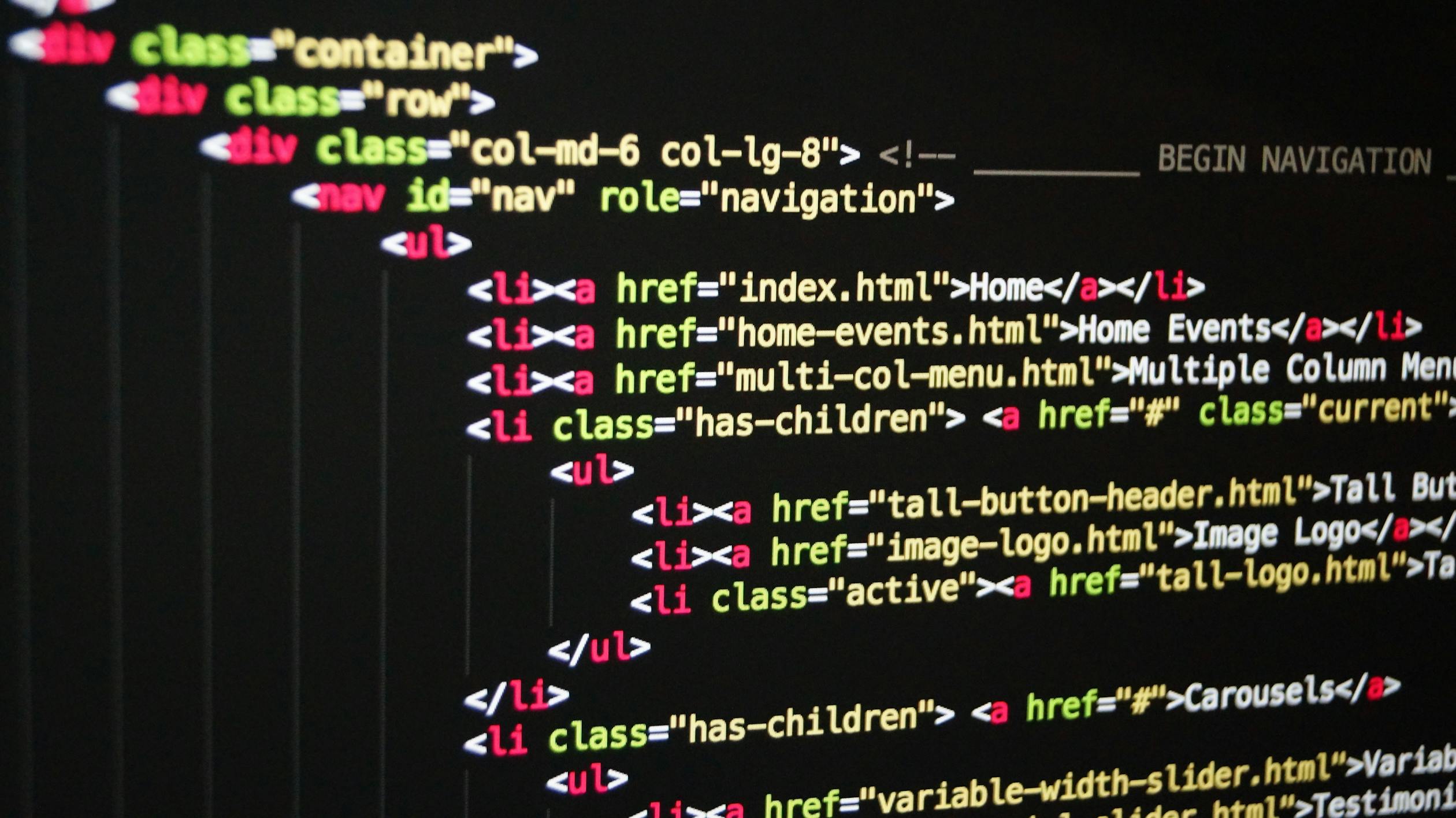
June 01, 2024
Getting Started with React Native and Expo: A Beginner's Guide
Getting Started with React Native and Expo: A Beginner's Guide
INTRODUCTION
In this series, we will explore React Native, a powerful framework for building mobile applications for both Android and iOS. We'll use Expo, a framework that simplifies the development process, providing essential tools and APIs.
What is React Native?
According to the official documentation, "React Native allows developers who know React to create native apps. At the same time, native developers can use React Native to gain parity between native platforms by writing common features once." Essentially, React Native is a collection of special React components that are compiled into native UI elements for Android, iOS, Web, and TV devices. It is used alongside React.js, a library for building user interfaces, to create fully functional mobile applications. While React.js is platform-agnostic, React Native provides the necessary components and APIs for building mobile applications, making it comparable to React DOM in web development.
Prerequisites for Learning React Native
To effectively learn React Native, you should have a foundational knowledge of React.js, as it is fully integrated into React Native. The code is written in React.js but uses React Native components, which are compiled into their respective native iOS and Android counterparts. Additionally, this series will use TypeScript, so familiarity with TypeScript is essential.
Under the Hood of React Native
React Native uses JSX, just like web-based React.js. The JavaScript code in JSX is not compiled but runs at runtime with the help of Node.js, hosted within the application by React Native. However, React Native uses special components like the View
and Text
components.
import React from 'react';
import { View, Text } from 'react-native';
const App = () => (
<View>
<Text>Hello, React Native!</Text>
</View>
);
export default App;
These components are compiled into their native equivalents: View
becomes android.view
on Android and UIView
on iOS. Similarly, the TextInput
component in React Native corresponds to EditText
in Android and UITextField
in iOS.
Installation
First, ensure Node.js is installed on your machine nodejs. Throughout this series, we'll use Expo, a framework that simplifies the setup and configuration process, providing tools like file-based routing, high-quality libraries, and plugins to modify native code without managing native files.
Starting a React Native App with Expo
To create a new Expo project, run:
npx create-expo-app -t expo-template-blank-typescript
This command will ensure we are starting up a new project from scratch without expo startup templates.
Follow the prompts to name your project. Once installation is complete, navigate into your project folder and start the application on Android or iOS.
Exploring the Folder Structure
- assets
: It stores app assets like icons and images.
- node_modules
: Contains third-party packages.
- package.json
: Lists project dependencies.
- package-lock.json
: Locks dependency versions.
- babel.config.js
: Configures code transpilation (leave unchanged unless necessary).
- app.json
: Configures app settings for Expo builds (e.g., name, version, icon).
- App.tsx
: The main code file, containing a JSX component.
Previewing Your Application
To preview your application, download the Expo Go app from your device's app store. Run the following command to start the Expo development server:
npm run start
Scan the QR code displayed in your terminal with the Expo Go app. On iOS, use your camera to scan the code. This will link your app to Expo Go, allowing you to see changes in real time.
Using Simulators
If you don't have access to a physical Android or iOS device, you can use emulators. Android Studio offers an Android emulator, while Xcode provides an iOS simulator (only available on macOS). For more information, refer to the official documentation: Android Studio and Xcode.
Conclusion
In this first post, we've set up our development environment and explored the basics of React Native and Expo. In the next post, we'll dive deeper into building and styling components. Stay tuned!
Github Repo: https://github.com/Intuneteq/react-native-tutorials
React Native Doc: https://docs.expo.dev/
1.4k views