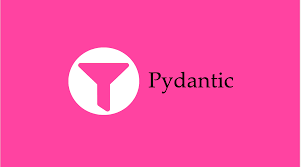
In the world of programming, one of the most crucial aspects of writing robust and maintainable code is ensuring type safety. Type safety helps catch errors early in the development process, reduces debugging time, and enhances the overall reliability of the codebase. While Python is known for its flexibility and ease of use, it has historically been criticized for its lack of strong typing. However, with libraries like Pydantic, Python developers can now enjoy the benefits of type safety without sacrificing the language's flexibility.
What is Pydantic?
Pydantic is a data validation and settings management library for Python. It's designed to make it easy to define data schemas and validate input data against those schemas. Pydantic leverages Python's type hinting system to define the structure of data and enforce type safety at runtime. It provides a concise syntax for defining data models and comes with built-in support for common data types, validation rules, and serialization/deserialization.
Defining Data Models with Pydantic
One of the key features of Pydantic is its ability to define data models using Python's type hints. Let's consider a simple example of defining a data model for a user:
from pydantic import BaseModel
class User(BaseModel):
id: int
username: str
email: str
In this example, we've defined a User class that inherits from BaseModel. The class attributes id, username, and email represent the fields of the user object, each annotated with its respective type hint.
Type Validation and Coercion
Once we've defined a data model using Pydantic, we can create instances of that model and validate input data against it:
user_data = {"id": 1, "username": "john_doe", "email": "john@example.com"}
user = User(**user_data)
Pydantic will automatically validate the input data against the defined schema and raise an error if the data doesn't match the expected types. Additionally, Pydantic can coerce input data to the specified types if possible, providing a seamless conversion mechanism.
Handling Optional and Default Values
Pydantic supports optional and default values for fields, making it easy to define flexible data models:
from typing import Optional
class Item(BaseModel):
name: str
description: Optional[str] = None
price: float = 0.0
In this example, the description field is optional, and the price field has a default value of 0.0. Pydantic ensures that even if these fields are omitted from the input data, the resulting object will have the correct structure and type.
Serialization and Deserialization
Pydantic also provides built-in support for serialization and deserialization of data objects. This allows seamless conversion between Python objects and JSON-compatible data:
user_json = user.json()
print(user_json)
# Output: {"id": 1, "username": "john_doe", "email": "john@example.com"}
user_dict = user.dict()
print(user_dict)
# Output: {'id': 1, 'username': 'john_doe', 'email': 'john@example.com'}
Conclusion
Pydantic is a powerful library that brings type safety and data validation to Python in an elegant and intuitive way. By leveraging Python's type hinting system, Pydantic allows developers to define clear and concise data models, validate input data, and ensure type safety at runtime. With its support for optional fields, default values, and serialization/deserialization, Pydantic simplifies the task of working with complex data structures and enhances the reliability and maintainability of Python codebases. Whether you're building web applications, APIs, or data processing pipelines, Pydantic is a valuable tool for ensuring the integrity of your data and the robustness of your code.
579 views