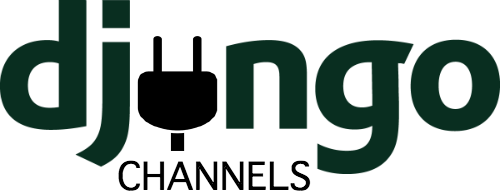
August 12, 2024
Real-Time Live Updates in Django Using WebSockets and Django Channels
In the realm of web development, providing real-time updates to users has become increasingly important. Whether it's live chat applications, collaborative editing tools, or dynamic dashboards, users expect information to update instantly without the need for manual refreshes. Traditionally, achieving real-time updates in web applications has been challenging, often requiring workarounds like polling or long-polling techniques. However, with the advent of WebSockets and frameworks like Django Channels, developers can implement real-time functionality seamlessly. In this article, we'll delve into how to incorporate real-time live updates into a Django application using WebSockets and Django Channels.
Understanding WebSockets
WebSockets represent a significant advancement in web technology, allowing for full-duplex communication channels over a single, long-lived TCP connection. Unlike traditional HTTP requests, where the client initiates communication and the server responds, WebSockets enable both the client and server to initiate communication independently. This bidirectional communication capability makes WebSockets ideal for real-time applications.
Introducing Django Channels
Django Channels is a powerful extension to the Django web framework that enables handling WebSockets, background tasks, and other asynchronous code alongside traditional HTTP requests. By integrating Channels into a Django project, developers can build applications that support real-time features without resorting to hacks or workarounds.
Setting Up Django Channels
To get started with Django Channels, you first need to install it in your Django project. You can do this using pip:
pip install channels
Once installed, you need to add Channels to your Django project's settings:
# settings.py
INSTALLED_APPS = [
# Other installed apps
'channels',
]
Next, you'll need to configure your routing to include WebSocket consumers. This involves creating a routing.py file within your Django app:
# routing.py
from channels.routing import ProtocolTypeRouter, URLRouter
from channels.auth import AuthMiddlewareStack
from django.urls import path
from myapp.consumers import MyConsumer
application = ProtocolTypeRouter({
"websocket": AuthMiddlewareStack(
URLRouter([
path("ws/myapp/", MyConsumer.as_asgi()),
])
),
})
Here, MyConsumer is a class that defines how your application handles WebSocket connections.
Implementing Real-Time Updates
With the setup in place, implementing real-time updates becomes relatively straightforward. Inside your MyConsumer class, you can define methods to handle various WebSocket events, such as connect, disconnect, and receive. When a client connects to the WebSocket URL defined in your routing configuration, the connect method is called. This is where you can perform any necessary setup, such as subscribing the client to relevant channels or groups.
# consumers.py
from channels.generic.websocket import WebsocketConsumer
class MyConsumer(WebsocketConsumer):
def connect(self):
# Accept the connection
self.accept()
# Subscribe the client to a specific group
async_to_sync(self.channel_layer.group_add)("realtime_updates", self.channel_name)
def disconnect(self, close_code):
# Unsubscribe the client from the group
async_to_sync(self.channel_layer.group_discard)("realtime_updates", self.channel_name)
def receive(self, text_data):
# Handle incoming messages from the client
pass
In this example, clients connecting to the WebSocket endpoint /ws/myapp/ will be subscribed to the realtime_updates group.
Broadcasting Updates
Once clients are subscribed to the appropriate groups, you can broadcast updates to them whenever relevant events occur. This could be triggered by user actions, changes to data in the database, or external events. Django Channels provides a convenient way to broadcast messages to groups using the group_send method.
# In your Django views or wherever appropriate
from asgiref.sync import async_to_sync
from channels.layers import get_channel_layer
channel_layer = get_channel_layer()
# Broadcast a message to the 'realtime_updates' group
async_to_sync(channel_layer.group_send)(
"realtime_updates",
{
"type": "send_realtime_update",
"content": "New data available!",
}
)
Updating the Client
Finally, on the client-side, you need to handle incoming WebSocket messages and update the UI accordingly. This typically involves writing JavaScript code to establish a WebSocket connection and define event handlers for receiving messages.
// JavaScript code in your frontend
const socket = new WebSocket("ws://localhost:8000/ws/myapp/");
socket.onmessage = function(event) {
// Handle incoming messages from the server
const message = JSON.parse(event.data);
// Update UI based on the received message
};
With this setup, your Django application can seamlessly deliver real-time updates to clients via WebSockets, providing a smoother and more responsive user experience.
Conclusion
Incorporating real-time functionality into Django applications using WebSockets and Django Channels opens up a world of possibilities for creating dynamic and interactive web experiences. By following the steps outlined in this article, you can empower your Django projects with live updates, enabling features like live chat, real-time notifications, and collaborative editing. Embracing these technologies not only enhances user engagement but also demons
793 views