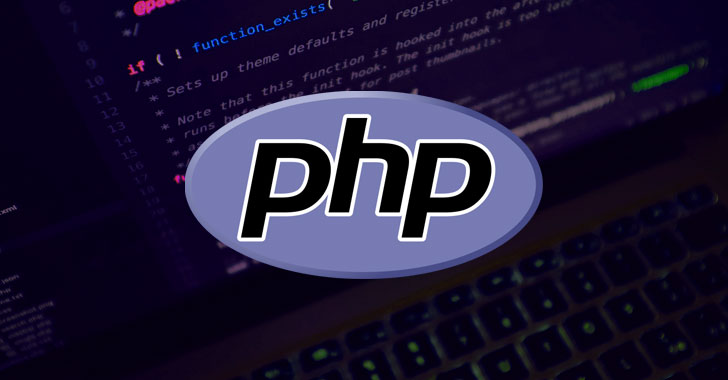
August 15, 2024
Understanding Return Type Declaration in PHP: Enhancing Clarity and Reliability
In the dynamic landscape of web development, PHP continues to be a cornerstone for building robust and scalable applications. With the introduction of features like return type declaration in PHP 7.0, developers have gained additional tools to enhance code clarity and reliability. Let's delve deeper into return type declaration with a variety of examples to showcase its usage and benefits.
Example 1: Basic Function with Return Type Declaration
function add(int $a, int $b): int {
return $a + $b;
}
$result = add(5, 3); // $result will be of type int (8)
In this example, the function add takes two integer parameters $a and $b and returns their sum as an integer. The return type declaration : int ensures that the function indeed returns an integer value.
Example 2: Function Returning Array
function getEvenNumbers(int $limit): array {
$evenNumbers = [];
for ($i = 0; $i <= $limit; $i++) {
if ($i % 2 === 0) {
$evenNumbers[] = $i;
}
}
return $evenNumbers;
}
$evenArray = getEvenNumbers(10); // $evenArray will be an array containing even numbers from 0 to 10
In this example, the function getEvenNumbers returns an array containing even numbers up to the specified limit. The return type declaration : array communicates the expected return type clearly.
Example 3: Nullable Return Type
function findElement(array $arr, $search): ?int {
$index = array_search($search, $arr);
return $index !== false ? $index : null;
}
$index = findElement([1, 2, 3, 4, 5], 3); // $index will be an integer or null
Here, the function findElement searches for an element in an array and returns its index if found, or null if not found. The return type declaration ?int indicates that the function may return an integer or null.
Example 4: Function Returning Object
class User {
public string $name;
public int $age;
public function __construct(string $name, int $age) {
$this->name = $name;
$this->age = $age;
}
}
function createUser(string $name, int $age): User {
return new User($name, $age);
}
$newUser = createUser("John Doe", 30); // $newUser will be an instance of User
In this example, the function createUser creates and returns a new User object. The return type declaration : User specifies that the function returns an object of type User.
Example 5: Recursive Function with Return Type Declaration
function factorial(int $n): int {
return ($n <= 1) ? 1 : $n * factorial($n - 1);
}
$result = factorial(5); // $result will be 120 (5!)
Here, the function factorial calculates the factorial of a given integer recursively. The return type declaration : int ensures that the function returns an integer value.
Conclusion
Return type declaration in PHP is a powerful feature that promotes code clarity, improves error detection, and enhances maintainability. By explicitly specifying the expected return types of functions, developers can write more robust and predictable code. These examples demonstrate the versatility and benefits of return type declaration, empowering developers to build high-quality PHP applications with confidence.
262 views