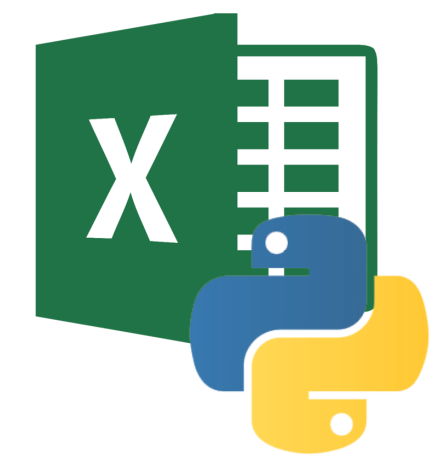
In today's data-driven world, Excel sheets remain a ubiquitous tool for organizing and analyzing data. Whether you're a software developer, data analyst, or business professional, knowing how to effectively read Excel sheets using programming languages like C# and Python can significantly enhance your data processing capabilities. In this blog post, we'll delve into the process of reading Excel sheets in both C# and Python, exploring libraries and techniques commonly used in each language.
Reading Excel Sheets in C#
ExcelDataReader Library
One of the popular libraries for reading Excel sheets in C# is ExcelDataReader. This library provides a simple and efficient way to read Excel files without needing Microsoft Office or Excel installed on the machine. Below is a basic example of how to use ExcelDataReader to read an Excel sheet:
using ExcelDataReader;
using System.IO;
class Program
{
static void Main(string[] args)
{
using (var stream = File.Open("example.xlsx", FileMode.Open, FileAccess.Read))
{
using (var reader = ExcelReaderFactory.CreateReader(stream))
{
// Iterate over rows and columns to process data
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
Console.Write(reader.GetValue(i) + "\t");
}
Console.WriteLine();
}
}
}
}
}
Reading Excel Sheets in Python
pandas Library
In the Python ecosystem, the pandas library is a powerful tool for data manipulation and analysis, including reading and writing Excel files. Here's a simple example of how to use pandas to read an Excel sheet:
import pandas as pd
# Read Excel sheet into a DataFrame
df = pd.read_excel("example.xlsx")
# Display the DataFrame
print(df)
Conclusion
Reading Excel sheets programmatically is a common task in both C# and Python development. In C#, the ExcelDataReader library offers a straightforward approach, while in Python, the pandas library provides a robust solution for data manipulation and analysis, including Excel file handling. By mastering these techniques, developers and data professionals can efficiently process Excel data in their applications, unlocking new possibilities for data-driven insights and decision-making. Whether you're working with financial data, sales reports, or any other tabular data stored in Excel, the ability to read and manipulate Excel sheets programmatically is a valuable skill worth mastering.
326 views