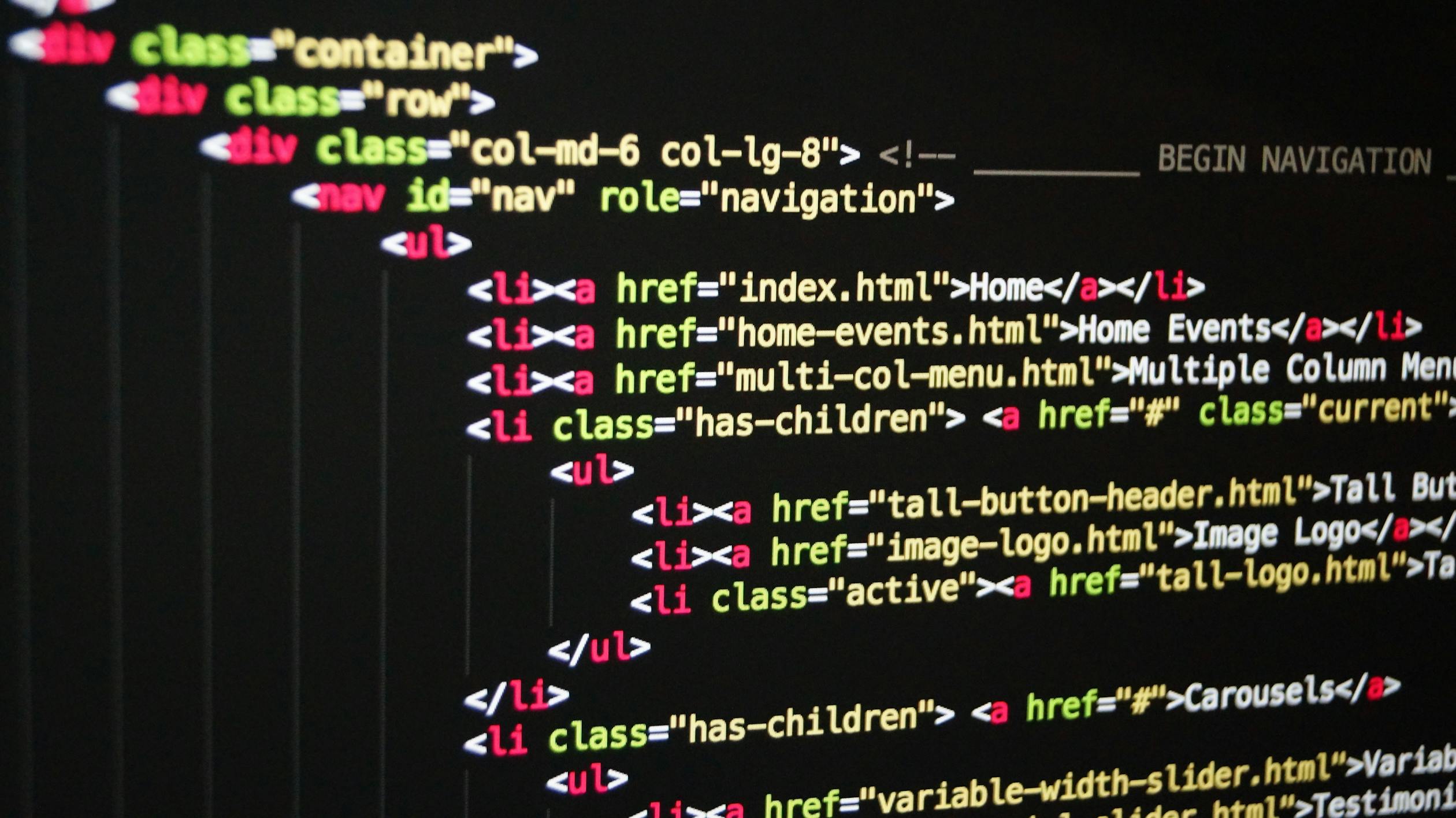
October 13, 2023
The Python 'Class' class - Object oriented programming part
Purpose
This document will introduce beginners to the object-oriented programming in Python via a hands-on tutorial.
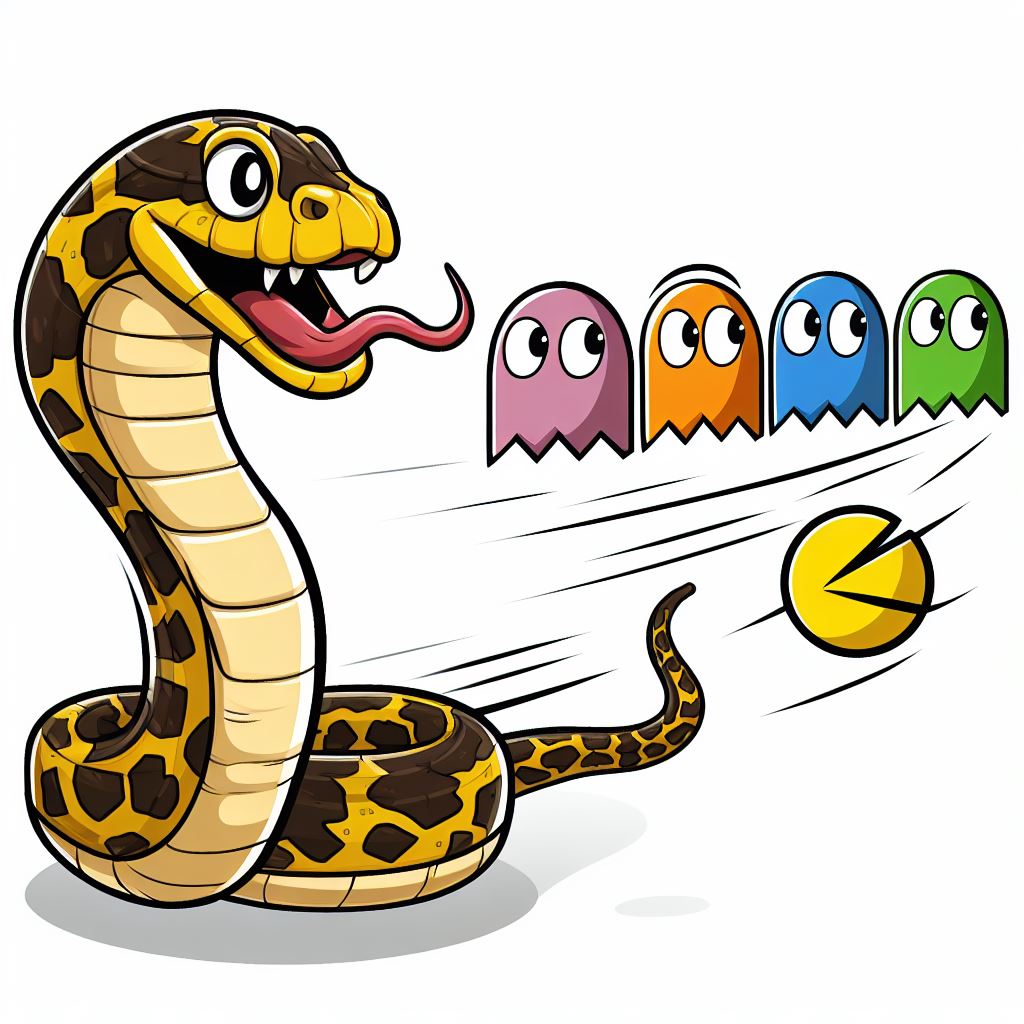
Audience
Newbies to programming or to Python.
Prerequistes
- Python interpreter installed on your computer - you can learn more about installing Python on your machine by checking out the pre-requisites in my article
- text editor or integrated development environment (IDE)
Introduction
Most languages you have heard of support object-orientated programming (OOP) in some way, so too does Python. There are many ways to explain OOP, but I find the best to be by example. Think of Pac-Man. There are four distinct 'ghosts', but each are essentially the same. In OOP terms, the four ghosts would be each be objects or instances of the 'ghost' class. They may have slightly different properties, but they are clones stamped out from the same template.
OOP is based on the concepts of classes, and instances (objects). Classes are the blueprint and instances are entities built from that blueprint.
An object has two important concepts:
- what it has (attributes)
- what it does (behaviours).
In our Pac-Man ghost example, each ghost has among many other things a name and a colour. These are properties. Each ghost does several things like move or spawn.
Most programming languages, Python included, express all of this with classes, we're going to learn how.
Step-by-step
Step 1 - Create a class
- let's start by creating our very first class. In your IDE or text editor create a new file called ghost.py - in this file, we will define our Ghost class.
class Ghost:
name = 'none'
colour = 'none
- save this file.
We cannot run anything now, there is nothing to run. We have defined a class called Ghost and given it two attributes (commonly called properties): name and colour.
- Now let's define some behaviours for our Ghost - we are going to add a method - a function that belongs to a class - to Ghost.
class Ghost:
name = 'none'
colour = 'none'
def say_name(self):
print("I am " + self.name)
NOTE: In the code above, we use a special variable called 'self'. Python uses 'self' to refer to a class' methods or properties.
Step 2 - Import the class into a Python program and create a ghost
- In the same directory as ghost.py, create a file called demo.py and add this line.
import ghost
What we have done here is taken our ghost class and made it available to our new program. Now let's create a ghost and have it say its name!
- create a ghost
import ghost
def main():
ghost1 = ghost.Ghost()
ghost1.say_name()
main()
In the first line, we created a variable called 'ghost1' by instantiating the Ghost class. On the next line, we invoked its 'say_name' function. You should see the output:
I am none
Well, 'none' is not a name befitting a Pac-Man ghost. Let's fix that.
Step 3 - Add a constructor to the Ghost class
A constructor is a special method in a class that sets (initialises) its properties when an instance of the class is created. In Python, the constructor function is always called "__init__".
- Add a constructor - add the __init__ function to ghost.py as shown below. Also change the print statement in the say_name function as shown.
class Ghost:
name = 'none'
colour = 'none'
def say_name(self):
print("I am " + self.name + " and my colour is " + self.colour)
def __init__(self, name, colour):
self.name = name
self.colour = colour
Two things to notice about the init function.
- The first argument is always 'self'.
- The other arguments can be whatever you like
Our init function accepts two arguments - name, and colour. It then uses them to set the corresponding class properties.
NOTE: some find it good practice to use arguments with the same name as the properties - I am doing that in this example - but it is not necessary. Here 'self' becomes useful for distinguishing between the two. You could use argument names such as 'input_name'.
Save ghost.py
- return to demo.py and change the third line as shown. Now, when we instantiate the Ghost class, we pass it a name and colour parameter.
import ghost
def main():
ghost1 = ghost.Ghost('Blinky', 'red')
ghost1.say_name()
main()
Run demo.py. The output should be:
I am Blinky and my colour is red
We've given our ghost a name and set its colour!
Step 4 - Repeat, repeat, repeat!
Classes allow us to create (theoretically) as many copies as we like with minimal duplication. So, instead of creating a class for every ghost, we can instantiate them using the generic ghost class!
- create the other ghosts - change demo.py as follows:
import ghost
def main():
ghost1 = ghost.Ghost('Blinky', 'red')
ghost1.say_name()
ghost2 = ghost.Ghost('Pinky', 'pink')
ghost2.say_name()
ghost3 = ghost.Ghost('Inky', 'cyan')
ghost3.say_name()
ghost4 = ghost.Ghost('Clyde', 'orange')
ghost4.say_name()
main()
Save and run demo.py
I am Blinky and my colour is red
I am Pinky and my colour is pink
I am Inky and my colour is cyan
I am Clyde and my colour is orange
Next steps
There is so much more to explore in object-orientated programming. For now, practice and get familiar with your Ghost class by trying the following exercises:
- create your own ghosts
- add your own method to the ghost class to print the name of a game you'd rather be playing
- create and import your own 'pacman' class
428 views