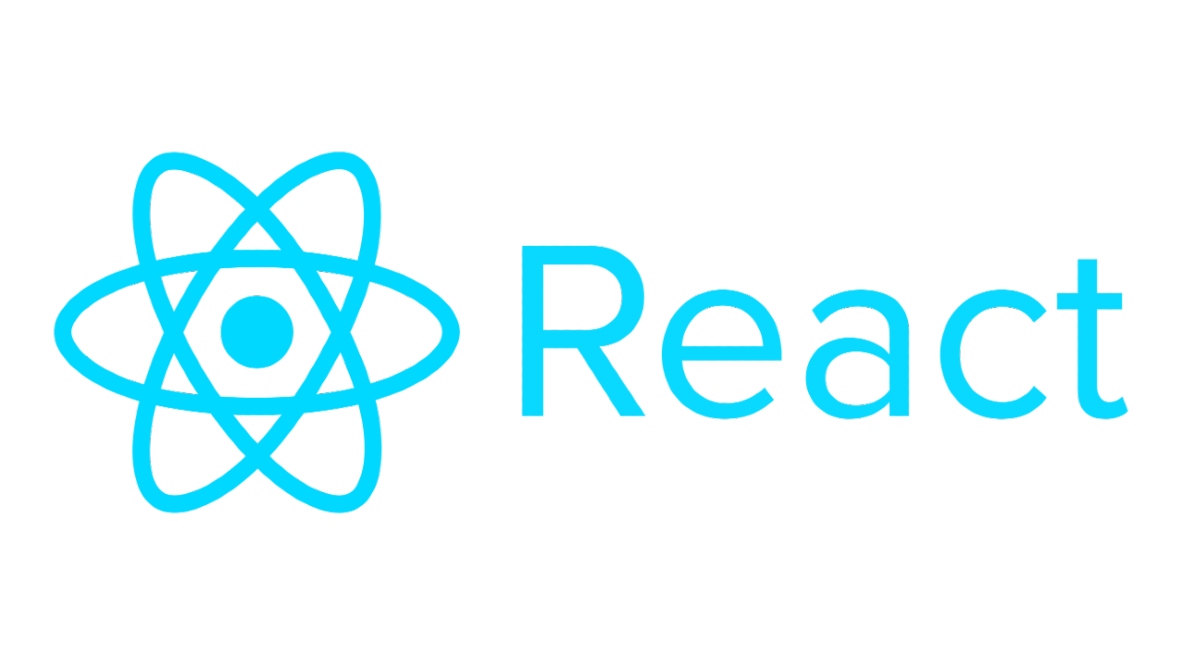
In the realm of web development, performance optimization is not just a luxury; it's a necessity. Users expect fast, responsive applications, and anything less can result in frustration and abandonment. In the world of React, a JavaScript library for building user interfaces, optimizing performance is both an art and a science. Let's delve into the strategies and best practices for maximizing performance in React applications.
Understanding React Performance
Before diving into optimization techniques, it's crucial to understand how React works under the hood. React utilizes a virtual DOM (Document Object Model) to efficiently update the actual DOM. When state or props change, React re-renders components and updates the virtual DOM. It then calculates the difference (referred to as "diffing") between the previous and current virtual DOM states and applies only the necessary changes to the actual DOM, minimizing expensive DOM manipulations.
Identifying Performance Bottlenecks
The first step in optimizing React performance is identifying bottlenecks. Common culprits include:
- Excessive Renders: Components re-render unnecessarily, consuming CPU cycles.
- Large Component Trees: Deep component trees can slow down rendering and reconciliation.
- Inefficient State Management: Poorly managed state can lead to unnecessary re-renders.
- Slow Component Mounting/Un-mounting: Complex components can take longer to mount or unmount, impacting performance.
- Network Latency: High network latency can cause delays in fetching data, affecting perceived performance.
Strategies for Optimization
1. Memoization with React.memo() and useMemo()
Memoization is a technique for caching the results of expensive function calls. In React, React.memo() and useMemo() can memoize functional components and computed values, respectively, preventing unnecessary re-renders.
const MemoizedComponent = React.memo(MyComponent);
2. Component Lazy Loading
Lazy loading enables components to load asynchronously, improving initial load times and reducing bundle size. React's lazy() function and Suspense component facilitate lazy loading effortlessly.
const LazyComponent = React.lazy(() => import('./LazyComponent'));
3. Virtualization for Large Lists
Rendering large lists efficiently can be challenging. Virtualization libraries like React Virtualized or React Window help by rendering only the items visible in the viewport, rather than the entire list.
4. Code Splitting
Splitting your code into smaller chunks and loading them asynchronously can reduce initial load times. React's React.lazy() and Suspense make code splitting straightforward.
5. Profiling and Performance Monitoring
Tools like React DevTools and Chrome DevTools provide insights into your application's performance. Use profiling to identify performance bottlenecks and monitor improvements over time.
Conclusion
Performance optimization is an ongoing process in React development. By understanding React's internals and employing optimization techniques like memoization, lazy loading, virtualization, and code splitting, you can create blazing-fast React applications that deliver exceptional user experiences. Remember, optimizing performance isn't just about speed; it's about creating applications that are responsive, efficient, and delightful to use.
951 views