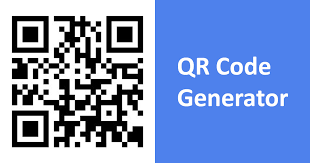
May 01, 2024
QR Code Generation with JavaScript: A Comprehensive Guide
In the modern digital age, QR (Quick Response) codes have become ubiquitous. From accessing websites and making payments to sharing contact information, QR codes offer a convenient way to bridge the physical and digital worlds seamlessly. And if you're a developer looking to integrate QR code generation into your web applications, JavaScript provides a powerful and flexible solution. In this guide, we'll explore how to create QR codes using JavaScript, unlocking a world of possibilities for your projects.
Understanding QR Codes
Before diving into the code, let's take a moment to understand what QR codes are and how they work. A QR code is a two-dimensional barcode that stores information both horizontally and vertically, allowing it to hold significantly more data than a traditional barcode. QR codes consist of black squares arranged on a white background, and they can encode various types of data, including URLs, text, contact information, and more.
Choosing a JavaScript Library
While it's possible to create QR codes from scratch using JavaScript, leveraging a specialized library can simplify the process and provide additional features. One popular library for generating QR codes is qrcode.js. This lightweight library allows you to generate QR codes dynamically with just a few lines of code.
Getting Started with qrcode.js
To begin, you'll need to include the qrcode.js library in your project. You can either download the library and include it manually or use a package manager like npm or yarn to install it.
<script src="https://cdn.jsdelivr.net/npm/qrcode@1.4.4/qrcode.min.js"></script>
Once you've included the library, you can start generating QR codes in your JavaScript code. Here's a basic example:
// Select the element where you want to render the QR code
const qrCodeContainer = document.getElementById('qr-code');
// Generate the QR code
new QRCode(qrCodeContainer, {
text: 'https://www.onlycoders.net',
width: 200,
height: 200,
});
In this example, we're creating a new QRCode instance and passing in the container element where we want to render the QR code. We also specify the text to encode, as well as the desired width and height of the QR code.
Customizing QR Codes
qrcode.js provides various options for customizing the appearance and behavior of QR codes. You can adjust parameters such as size, color, error correction level, and more to suit your needs. Here are a few examples of customization options:
// Customize the QR code
new QRCode(qrCodeContainer, {
text: 'Hello, world!',
width: 300,
height: 300,
colorDark: '#000000',
colorLight: '#ffffff',
correctLevel: QRCode.CorrectLevel.H,
});
Integrating QR Codes into Your Application
Once you've generated a QR code, you can integrate it into your web application in various ways. For example, you could display it on a web page for users to scan with their mobile devices, or you could generate QR codes dynamically in response to user actions.
Conclusion
In this guide, we've explored how to create QR codes using JavaScript with the qrcode.js library. By harnessing the power of JavaScript, you can generate dynamic QR codes that enhance the functionality and user experience of your web applications. Whether you're building a website, a mobile app, or a desktop application, QR codes offer a versatile solution for connecting the physical and digital worlds. With the knowledge gained from this guide, you're well-equipped to incorporate QR code generation into your projects and unlock a world of possibilities.
420 views