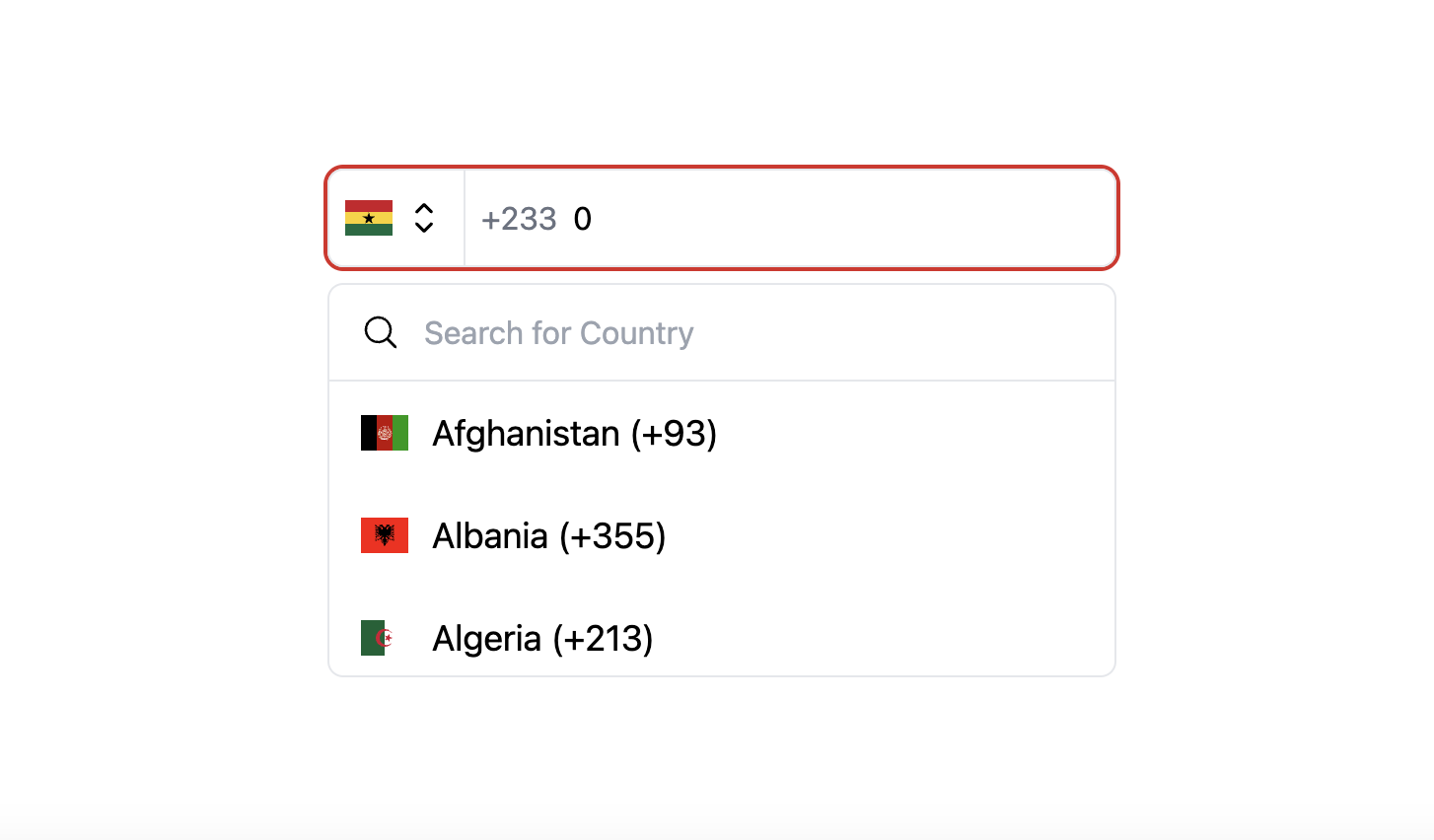
In modern web applications, forms often require user input for phone numbers. However, handling phone numbers can be complex due to different country codes, formats, and validation rules. In this tutorial, we'll explore how to create a custom phone input component in React that addresses these challenges using the libphonenumber-js library.
Prerequisites
To follow along with this tutorial, you'll need:
- Basic knowledge of React
- Node.js and npm installed on your development machine
Setting Up the Project
First, let's set up a new React project using Create React App:
npx create-react-app phone-input-demo
cd phone-input-demo
Next, install the necessary dependencies:
npm install libphonenumber-js
Creating the Phone Input Component
We'll start by creating a new file named PhoneInput.js in the src directory of our project. This file will contain our custom phone input component.
// PhoneInput.js
import { useState } from "react";
import Country from "../utils/countries"; // Assuming utils folder exists
import { LazySvg } from "./LazySvg";
export default function PhoneInput() {
// State variables
const [isOpen, setIsOpen] = useState(false);
const [search, setSearch] = useState("");
const [selectedIso2, setSelectedIso2] = useState("ca");
const [phone, setPhone] = useState("");
// Function to toggle dropdown
const toggleOpen = () => {
setIsOpen(!isOpen);
};
// Other component logic...
}
Integrating libphonenumber-js
We'll also create a Country.js file in a utils folder within our project to encapsulate logic related to country codes, formatting, and validation using libphonenumber-js.
// Country.js
import parsePhoneNumber, { AsYouType, isValidPhoneNumber } from "libphonenumber-js";
import examples from "libphonenumber-js/mobile/examples";
import data from "./data"; // Assuming data file with country information
export default class Country {
// Constructor and methods...
}
Building the User Interface
Our phone input component will consist of an input field for the phone number and a dropdown menu for selecting the country code. We'll use SVG icons to display flags representing each country.
// PhoneInput.js (continued)
// Inside PhoneInput component...
return (
<div className="w-full max-w-[400px] relative">
{/* Phone input field */}
<div className={`border rounded-lg group items-center gap-2 focus-within:ring-2 ...`}>
{/* Country code dropdown */}
{/* Input field */}
</div>
{/* Country dropdown */}
</div>
);
Handling User Interaction
We'll implement functionality for selecting a country code, inputting a phone number, and searching for countries in the dropdown menu.
// PhoneInput.js (continued)
// Inside PhoneInput component...
return (
<div className="w-full max-w-[400px] relative">
{/* Phone input field */}
<div className={`border rounded-lg group items-center gap-2 focus-within:ring-2 ...`}>
{/* Country code dropdown */}
{/* Input field */}
</div>
{/* Country dropdown */}
{isOpen && (
<div className="absolute max-h-[200px] flex flex-col overflow-hidden w-full ...">
{/* Search input */}
{/* List of countries */}
</div>
)}
</div>
);
Conclusion
In this tutorial, we've learned how to create a custom phone input component in React using the libphonenumber-js library. Our component allows users to select a country code, input a phone number, and provides validation and formatting as the user types. This component can be easily integrated into any React application, providing a seamless user experience for handling phone numbers.
Feel free to customize and extend this component to suit your specific requirements! Happy coding! ð
709 views