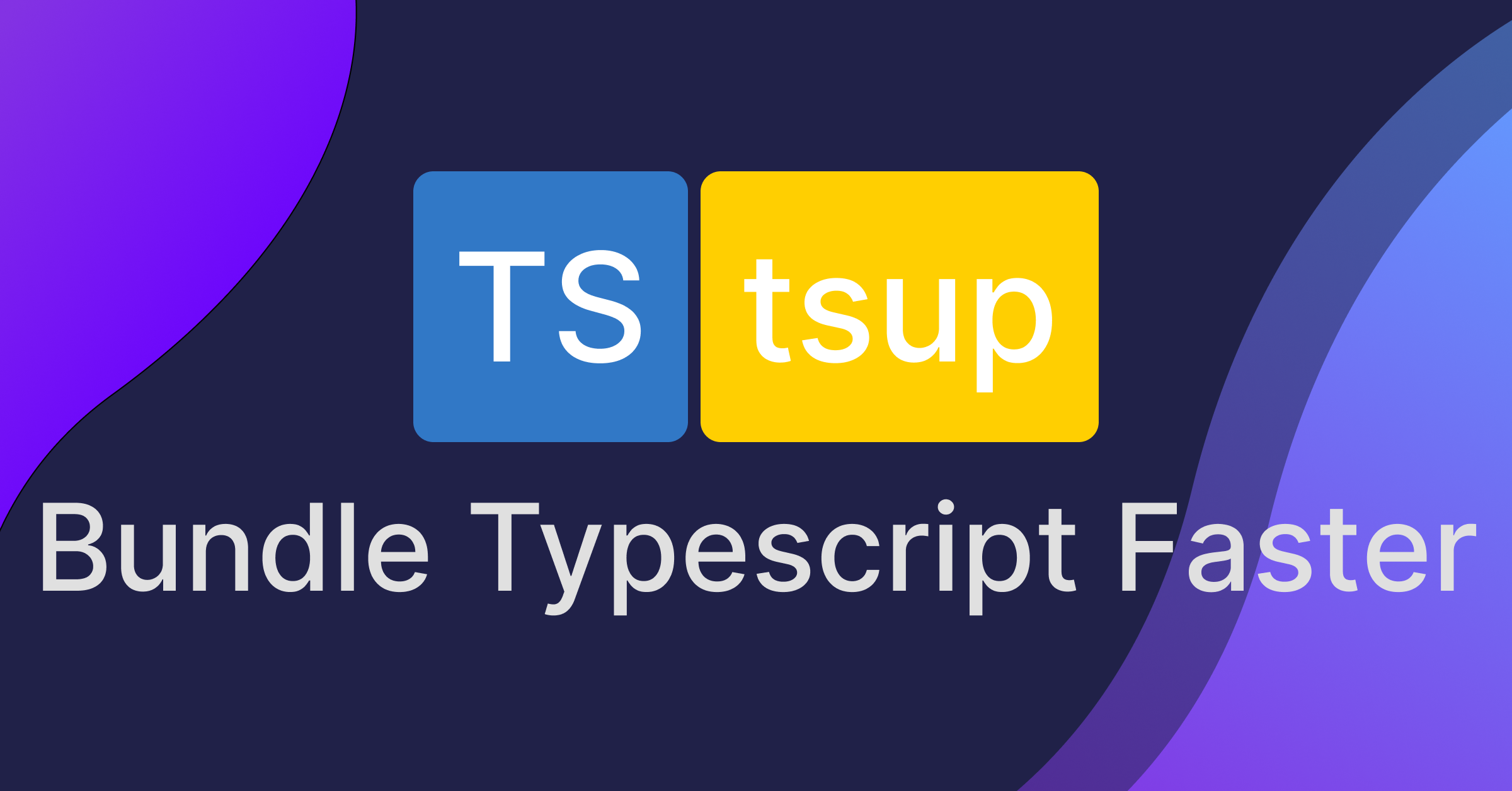
May 07, 2024
Building Your Own NPM Library (React, Tailwindcss, Tsup): Project Stepup
In the ever-evolving landscape of frontend development, staying ahead of the curve means embracing new tools and methodologies. One such evolution is the seamless integration of TypeScript libraries with Tailwind CSS, two powerful tools that, when combined, can significantly enhance the development process. In this blog post, we'll explore how to configure and integrate these tools to build robust and stylish frontend solutions efficiently.
The Rise of tsup and Tailwind CSS
Firstly, let's delve into the tools themselves. Tsup emerges as a no-config bundler crafted with esbuild, streamlining the process of building TypeScript libraries. On the other hand, Tailwind CSS revolutionizes styling with its utility-first approach, offering a comprehensive framework for creating visually appealing interfaces.
Bringing Them Together
While tsup and Tailwind CSS are often used independently, their synergy becomes apparent when building TypeScript libraries with stylish UI components. Combining their powers requires meticulous configuration, ensuring seamless compatibility and optimal performance.
Getting Started
The journey begins with setting up a new project directory and installing essential dependencies. With npm as our trusty companion, we initialize the project and install TypeScript, tsup, Tailwind CSS, and other necessary packages. These dependencies lay the foundation for our TypeScript library, empowering us to develop dynamic UI components with ease.
# Create a new project directory named `tsup-tailwind`.
# This should be replaced with the name of your library and used throughout.
mkdir tsup-tailwind
# Initialize `package.json`.
npm init -y
# Install the necessary development dependencies.
npm install -D typescript tsup tailwindcss autoprefixer
# More development dependencies. This post will use React for the UI components
# but this could be replaced with anything tsup/Tailwind both support.
npm install -D react react-dom @types/react @types/react-dom
Configuration: The Key to Harmony
Configuring each tool is crucial for smooth integration. We start by configuring TypeScript to enable JSX support and define compiler options tailored to our project's needs. Next, we set up tsup to bundle our TypeScript code into a distributable package, ensuring the inclusion of type definitions and sourcemaps for seamless debugging.
TypeScript Configuration
# Initialize the TypeScript configuration.
npx tsc --init
// tsconfig.json
{
"compilerOptions": {
"target": "es2016",
"module": "commonjs",
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true,
"strict": true,
"skipLibCheck": true,
"jsx": "react-jsx" // Enable JSX support
}
}
tsup Configuration
// tsup.config.ts
import { defineConfig } from "tsup";
export default defineConfig({
// The file we created above that will be the entrypoint to the library.
entry: ["src/index.tsx"],
// Enable TypeScript type definitions to be generated in the output.
// This provides type-definitions to consumers.
dts: true,
// Clean the `dist` directory before building.
// This is useful to ensure the output is only the latest.
clean: true,
// Sourcemaps for easier debugging.
sourcemap: true,
});
Tailwind CSS Configuration
Tailwind CSS configuration is equally vital, as it dictates how styles are generated and bundled. We initialize Tailwind CSS and tailor its configuration to our project's structure, specifying where to find CSS classes and customizing prefixes to avoid naming collisions. Additionally, we explore advanced Tailwind configurations, such as disabling global resets and implementing manual class-based dark mode.
# Initialize the Tailwind configuration.
npx tailwindcss init
// tailwind.config.js
module.exports = {
content: ["./src/**/*.tsx"],
prefix: "demo-", // Prefix to avoid naming collisions
corePlugins: {
preflight: false, // Disable global resets
},
darkMode: ["class", 'html[class~="dark"]'] // Manual class-based dark mode
};
Usage and Testing
Once configured, our TypeScript library with Tailwind CSS integration is ready for use. We explore how to import the package into a consuming application, ensuring that styles are seamlessly integrated. Furthermore, we share tips for local testing using tools like yalc, enabling developers to test changes locally before publishing to npm.
Conclusion
In conclusion, the integration of TypeScript libraries with Tailwind CSS offers a powerful toolkit for frontend developers. By leveraging the strengths of both tools and carefully configuring them, developers can streamline their development workflow, build stylish UI components, and deliver exceptional user experiences. As frontend tooling continues to evolve, embracing innovations like tsup and Tailwind CSS paves the way for faster, simpler, and more efficient frontend development.
Let's continue to explore and innovate, shaping the future of frontend development one line of code at a time!
346 views