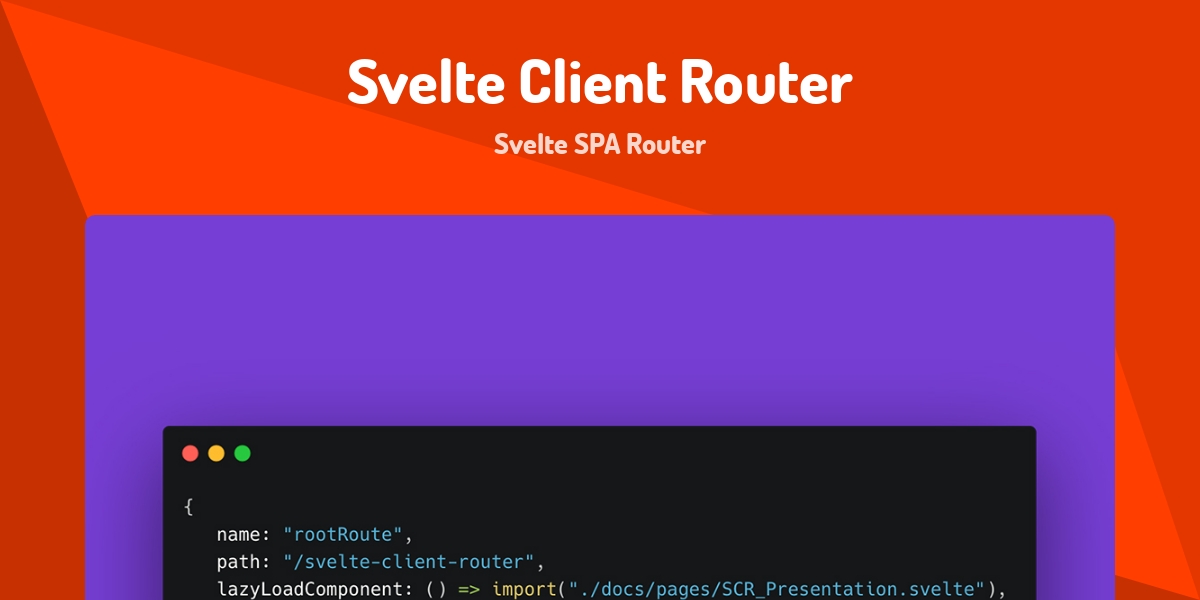
April 30, 2024
Exploring Routing in Svelte: A Comprehensive Guide
Routing is a fundamental aspect of web development, enabling users to navigate between different pages or views within a single-page application (SPA). While Svelte is renowned for its simplicity and performance, it also provides robust routing capabilities through various libraries. In this guide, we'll delve into routing in Svelte, exploring different approaches and libraries to implement navigation in your Svelte applications.
Why Routing Matters
Routing allows users to navigate seamlessly within an application, accessing different views or pages based on their interactions. In traditional web development, each page load triggers a full server request, resulting in slower navigation. SPAs address this issue by loading resources dynamically, providing a smoother user experience.
Svelte Routing Options
SvelteKit: SvelteKit, the official framework for building Svelte applications, comes with built-in routing capabilities. It offers file-based routing, making it easy to create routes by organizing files in the src/routes directory. SvelteKit automatically handles routing based on the file structure, simplifying the development process.
Svelte-Spa-Router: Svelte-Spa-Router is a lightweight routing library specifically designed for Svelte applications. It provides a simple API for defining routes and handling navigation. With Svelte-Spa-Router, you can create dynamic routes, nested routes, and handle route parameters with ease.
Page.js: Page.js is a minimalist routing library that can be used with Svelte applications. While it's not Svelte-specific, it integrates seamlessly with Svelte components, providing a flexible routing solution. Page.js allows you to define routes using a concise syntax and handle route changes using event listeners.
Getting Started with Svelte-Spa-Router
Let's explore how to implement routing in a Svelte application using Svelte-Spa-Router.
Installation: First, install Svelte-Spa-Router using npm:
npm install --save-dev svelte-spa-router
Creating Routes: Define your routes in the App.svelte component using the <Router> and <Route> components provided by Svelte-Spa-Router
<!-- App.svelte -->
<script>
import { Router, Route } from 'svelte-spa-router';
import Home from './routes/Home.svelte';
import About from './routes/About.svelte';
import Contact from './routes/Contact.svelte';
</script>
<Router>
<Route path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Router>
Creating Route Components: Create individual Svelte components for each route. For example, Home.svelte, About.svelte, and Contact.svelte.
Navigating Between Routes: Use the <Link> component provided by Svelte-Spa-Router to navigate between routes.
<!-- Home.svelte -->
<script>
import { Link } from 'svelte-spa-router';
</script>
<h1>Welcome to the Home Page</h1>
<Link to="/about">About</Link>
<Link to="/contact">Contact</Link>
Conclusion
Routing is an essential aspect of building modern web applications, enabling seamless navigation between different views. In Svelte, you have several options for implementing routing, ranging from built-in solutions like SvelteKit to lightweight libraries like Svelte-Spa-Router and Page.js. Experiment with different approaches to find the one that best suits your project's needs, and enjoy building dynamic, navigable Svelte applications!
599 views