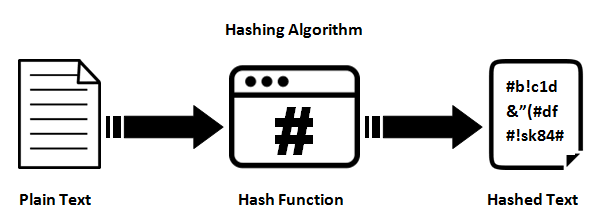
August 21, 2024
Understanding MD5 Hashing and Its Applications in JavaScript
In the world of computer science and cybersecurity, hashing algorithms play a pivotal role in ensuring data integrity and security. One such algorithm that has been widely used is MD5 (Message Digest Algorithm 5). Despite its age and some vulnerabilities discovered over time, MD5 remains relevant, especially in specific use cases. In this article, we'll delve into MD5, its workings, and its usage in JavaScript.
What is MD5?
MD5 is a widely-used cryptographic hash function that produces a 128-bit (16-byte) hash value. It was designed by Ronald Rivest in 1991 to replace the earlier MD4 algorithm. MD5 is often used to verify data integrity because it produces a unique fixed-size hash value from variable-length input data.
How Does MD5 Work?
MD5 operates by repeatedly taking a 512-bit block of input data and processing it through a series of modular arithmetic operations. The algorithm breaks the input message into chunks of 512 bits (64 bytes) and processes each chunk sequentially. At the end of processing, MD5 produces a 128-bit hash value, typically represented as a 32-character hexadecimal number.
The key properties of MD5 include:
- Deterministic: Given the same input, MD5 will always produce the same output.
- Fast Computation: MD5 is relatively fast to compute, making it suitable for applications that require quick hashing.
- Fixed Output Size: Regardless of the input size, MD5 always produces a fixed-size output.
Usage of MD5 in JavaScript
In JavaScript, MD5 hashing can be implemented using various libraries or built-in functions. One popular library for MD5 hashing in JavaScript is the crypto-js library. Below is a simple example demonstrating how to calculate the MD5 hash of a string using crypto-js:
const CryptoJS = require("crypto-js");
const inputString = "Hello, MD5!";
const hash = CryptoJS.MD5(inputString).toString();
console.log("MD5 Hash:", hash);
In this example, we first import the crypto-js library. Then, we define an input string (inputString) whose MD5 hash we want to calculate. We use the MD5 function provided by crypto-js to compute the hash, and finally, we convert the hash to a string representation for easy display.
Common Use Cases
While MD5 is no longer considered secure for cryptographic purposes due to vulnerabilities such as collision attacks, it still finds utility in various non-security-critical applications. Some common use cases include:
- Checksum Verification: MD5 can be used to verify the integrity of data during transmission or storage by comparing the hash of the received data with the original hash.
- Data Deduplication: MD5 hashes can be used to identify duplicate files or data chunks efficiently.
- Hash-based Data Retrieval: In databases or caching systems, MD5 hashes can serve as keys for quick data retrieval.
Conclusion
MD5, despite its age and known vulnerabilities, remains relevant for certain applications where cryptographic security is not the primary concern. In JavaScript, MD5 hashing can be easily implemented using libraries like crypto-js, providing developers with a convenient way to incorporate hash functionality into their applications. However, it's crucial to understand the limitations of MD5 and use it judiciously based on the specific requirements of the application.
224 views