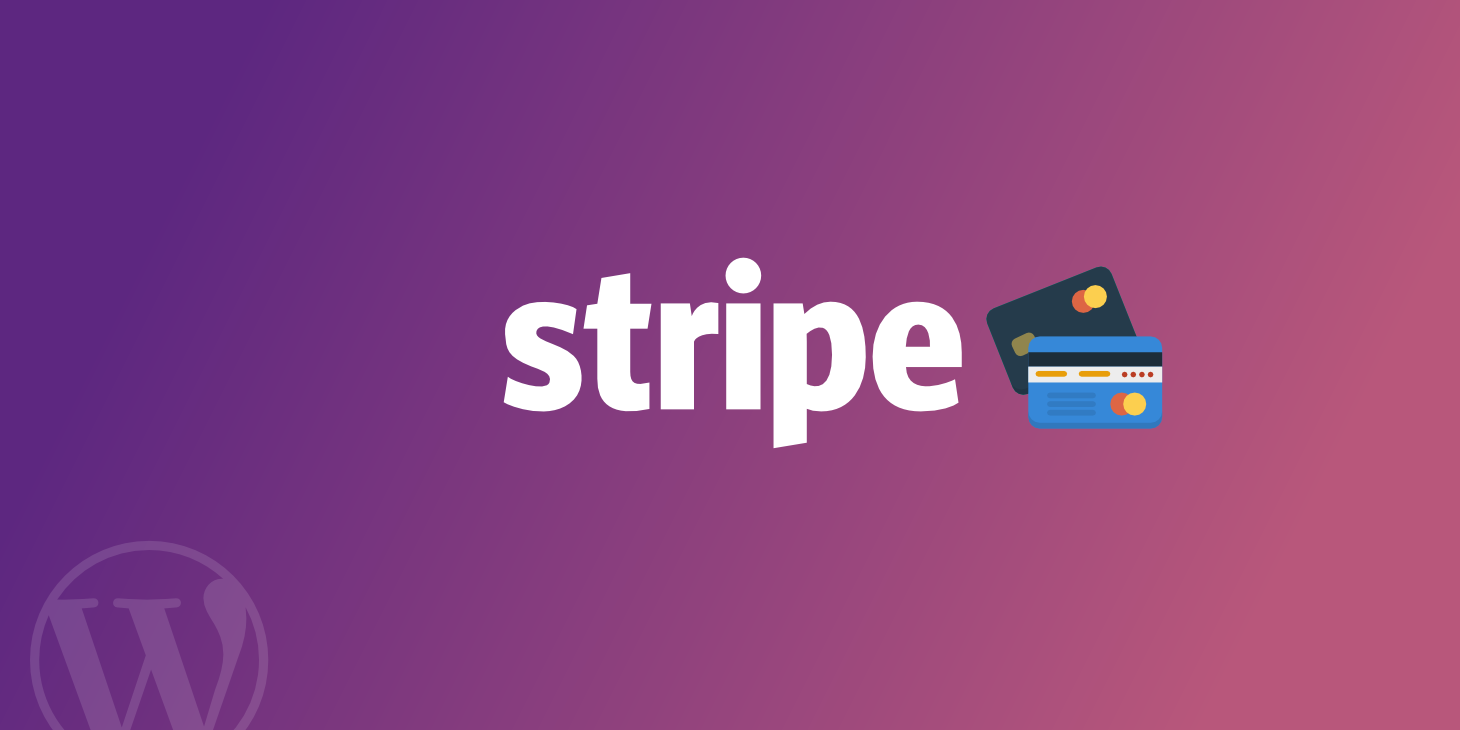
May 17, 2024
Streamlining Payment Webhooks with Stripe and Node.js
In the world of e-commerce and digital transactions, ensuring smooth and secure payment processing is paramount. For businesses operating online, integrating a reliable payment gateway like Stripe can be a game-changer. However, simply integrating Stripe isn't enough; handling events and updates from Stripe, particularly through webhooks, is crucial for maintaining accurate records and providing a seamless user experience.
In this guide, we'll delve into implementing payment webhooks with Stripe using Node.js. By the end, you'll have a robust system in place to handle various payment-related events effortlessly.
Understanding Stripe Webhooks
Before diving into implementation, let's grasp the concept of webhooks. Webhooks are HTTP callbacks triggered by specific events. In the context of Stripe, these events could be payment success, failure, disputes, or subscription cancellations.
By setting up webhooks, your application can receive real-time notifications about these events, allowing you to update your database, trigger actions, or send notifications to users accordingly.
Setting Up Your Stripe Account
To get started, you'll need a Stripe account. Once logged in:
- Navigate to the "Developers" section.
- Click on "Webhooks" to configure webhook endpoints.
- Generate a signing secret, which will be used to verify webhook payloads.
Building the Node.js Application
Now, let's dive into the Node.js implementation:
Initialize Your Project
Start by creating a new Node.js project and installing the necessary dependencies:
mkdir stripe-webhook-demo
cd stripe-webhook-demo
npm init -y
npm install express stripe
Set Up Express Server
Create an index.js file and set up a basic Express server:
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
app.use(express.json());
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Handle Stripe Webhooks
Implement the route to handle incoming webhook events:
const stripe = require('stripe')(process.env.STRIPE_SECRET_KEY);
app.post('/webhook', async (req, res) => {
const sig = req.headers['stripe-signature'];
let event;
try {
event = stripe.webhooks.constructEvent(req.body, sig, process.env.STRIPE_WEBHOOK_SECRET);
} catch (err) {
console.error(err.message);
return res.status(400).send(`Webhook Error: ${err.message}`);
}
// Handle the event
switch (event.type) {
case 'payment_intent.succeeded':
// Handle successful payment
break;
case 'payment_intent.payment_failed':
// Handle failed payment
break;
// Handle other events as needed
default:
console.log(`Unhandled event type: ${event.type}`);
}
res.json({ received: true });
});
Run Your Application
Before running the application, make sure to set environment variables for your Stripe secret key and webhook secret:
export STRIPE_SECRET_KEY=your_secret_key
export STRIPE_WEBHOOK_SECRET=your_webhook_secret
Now, start your server:
node index.js
Conclusion
Implementing payment webhooks with Stripe and Node.js opens up a world of possibilities for handling payment-related events in your application. By following this guide, you've established a solid foundation to receive, process, and respond to webhook events seamlessly.
Remember to handle events securely, validate signatures, and implement error handling to ensure the reliability and security of your payment system. With Stripe's robust API and Node.js's flexibility, you're well-equipped to build powerful, transactional applications.
548 views