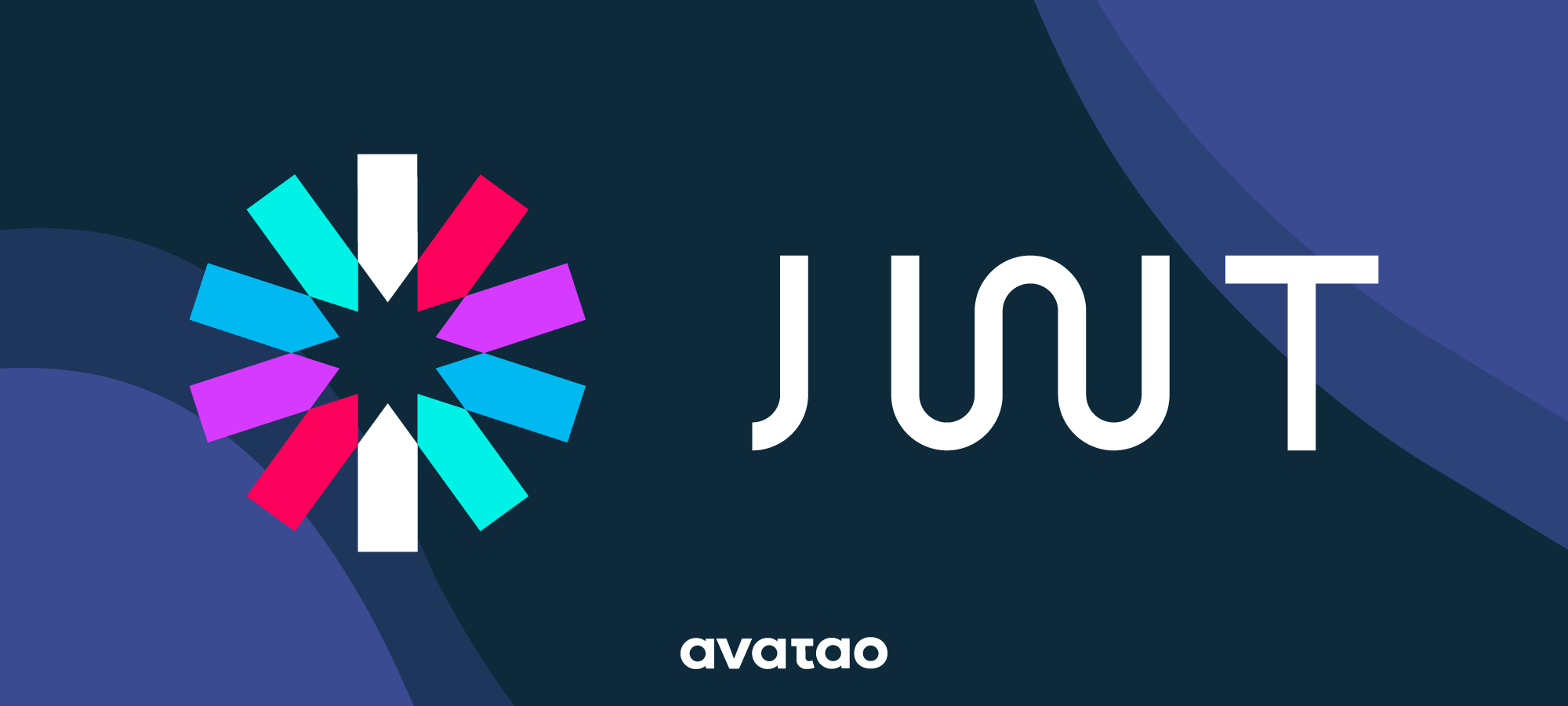
July 08, 2024
Refreshing JWT Tokens in React Applications: Best Practices and Implementation
In today's web development landscape, security is paramount. One widely adopted method for securing web applications is JSON Web Tokens (JWT). JWT provides a secure way of transmitting information between parties as a JSON object. However, JWT tokens have a finite lifespan, and managing their expiration and refreshing them is essential for maintaining a secure application.
In this blog post, we'll delve into the importance of refreshing JWT tokens in React applications, explore best practices for implementation, and provide a practical guide on how to achieve this in your projects.
Why Refresh JWT Tokens?
JWT tokens come with an expiration time, typically set by the server when the token is issued. This expiration time is crucial for security purposes, as it limits the window of opportunity for attackers to misuse stolen tokens. Once a token expires, the user must re-authenticate to obtain a new one.
Refreshing JWT tokens is a mechanism to ensure uninterrupted user sessions without requiring users to repeatedly log in. By refreshing tokens before they expire, applications can maintain a seamless user experience while maintaining security.
Best Practices for Implementing Token Refreshing
1. Set a Reasonable Expiration Time:
When issuing JWT tokens, set a reasonable expiration time based on your application's security requirements. A balance must be struck between security and usability. Too short an expiration time might inconvenience users with frequent re-authentication, while too long a duration increases the risk of token misuse.
2. Use Refresh Tokens:
Refresh tokens are long-lived tokens used to obtain new JWT tokens without requiring the user to re-enter their credentials. These refresh tokens should be securely stored on the client side, such as in an HTTP-only cookie, to mitigate the risk of theft.
3. Implement Token Refresh Endpoints:
Create server-side endpoints specifically for refreshing JWT tokens. These endpoints should verify the validity of the refresh token and issue a new JWT token if the refresh token is valid.
4. Handle Token Expiration Gracefully:
In your React application, implement logic to detect when a JWT token is about to expire. Trigger the token refresh process before the token expiration to prevent interruptions in user sessions.
Implementing Token Refreshing in a React Application
Now, let's see how we can implement token refreshing in a React application using Axios for HTTP requests and JWT-decode for decoding JWT tokens.
import axios from 'axios';
import jwt_decode from 'jwt-decode';
const refreshToken = async () => {
try {
const refreshToken = localStorage.getItem('refreshToken');
const response = await axios.post('/api/refresh-token', { refreshToken });
const { accessToken, newRefreshToken } = response.data;
// Update the JWT token and refresh token in local storage
localStorage.setItem('accessToken', accessToken);
localStorage.setItem('refreshToken', newRefreshToken);
return accessToken;
} catch (error) {
// Handle token refresh failure (e.g., redirect to login page)
console.error('Token refresh failed:', error);
throw error;
}
};
const api = axios.create({
baseURL: 'https://your-api.com',
});
// Interceptor to automatically refresh JWT token if expired
api.interceptors.request.use(
async (config) => {
const accessToken = localStorage.getItem('accessToken');
const decodedToken = jwt_decode(accessToken);
const currentTime = Date.now() / 1000;
if (decodedToken.exp < currentTime) {
// Token has expired, refresh it
const newAccessToken = await refreshToken();
config.headers.Authorization = `Bearer ${newAccessToken}`;
} else {
// Token is still valid, use it
config.headers.Authorization = `Bearer ${accessToken}`;
}
return config;
},
(error) => {
return Promise.reject(error);
}
);
export default api;
In this example, we've created an Axios instance with an interceptor that automatically refreshes the JWT token before making any HTTP request if the token has expired. The refreshToken function sends a request to the server to obtain a new JWT token using the refresh token stored in local storage.
Conclusion
Refreshing JWT tokens in React applications is crucial for maintaining both security and usability. By following best practices and implementing token refreshing logic, you can ensure that your application remains secure while providing a seamless user experience. With the provided implementation example, you can integrate token refreshing into your React pro
722 views