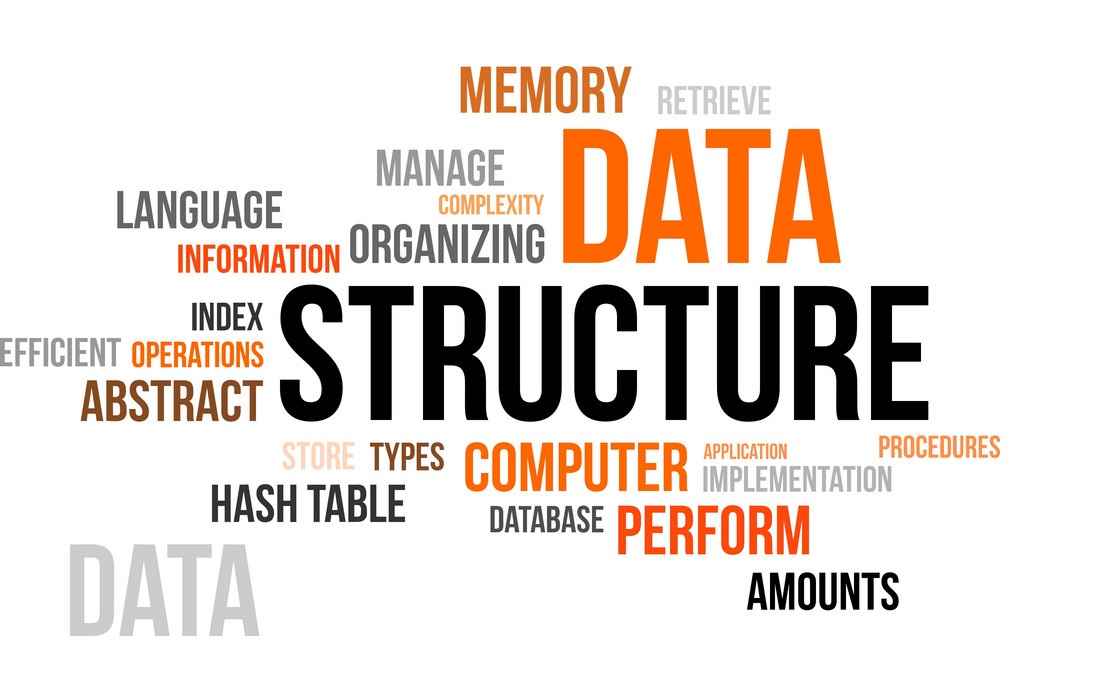
April 18, 2024
Exploring Classic Algorithms in JavaScript: Palindrome Check, Caesar Cipher, and Common Character Finder
In the vast world of computer science, certain algorithms stand the test of time. Whether it's for their simplicity, elegance, or practical applications, these classics continue to be relevant in modern programming. In this blog post, we'll delve into three such timeless algorithms: Palindrome Check, Caesar Cipher, and Common Character Finder. We'll explore their concepts, implementations in JavaScript, and how they can be applied in real-world scenarios.
Palindrome Check: A palindrome is a word, phrase, number, or other sequences of characters that reads the same forward and backward (ignoring spaces, punctuation, and capitalization). Palindrome checking is a common problem in programming interviews and is useful in various applications such as validating input or analyzing data.
Implementation in JavaScript:
function isPalindrome(str) {
const cleanedStr = str.toLowerCase().replace(/[^a-z0-9]/g, '');
const reversedStr = cleanedStr.split('').reverse().join('');
return cleanedStr === reversedStr;
}
// Test cases
console.log(isPalindrome("A man, a plan, a canal, Panama")); // true
console.log(isPalindrome("racecar")); // true
console.log(isPalindrome("hello")); // false
Caesar Cipher: The Caesar cipher is one of the simplest and most widely known encryption techniques. It's a type of substitution cipher where each letter in the plaintext is shifted a certain number of places down or up the alphabet. Despite its simplicity, the Caesar cipher was used by Julius Caesar himself to communicate secretly.
Implementation in JavaScript:
function caesarCipher(str, shift) {
return str.replace(/[a-zA-Z]/g, (char) => {
const base = char.charCodeAt(0) < 97 ? 65 : 97;
return String.fromCharCode((char.charCodeAt(0) - base + shift) % 26 + base);
});
}
// Test case
console.log(caesarCipher("Hello, World!", 3)); // "Khoor, Zruog!"
Common Character Finder: Finding common characters in two strings is a common problem in programming. It can be useful in tasks like determining the similarity between texts or identifying shared elements in datasets.
Implementation in JavaScript:
function commonCharacters(str1, str2) {
const set1 = new Set(str1);
const common = new Set([...str2].filter(char => set1.has(char)));
return [...common].join('');
}
// Test case
console.log(commonCharacters("abcdef", "cdegh")); // "cde"
Conclusion:
These classic algorithmsââ¬âPalindrome Check, Caesar Cipher, and Common Character Finderââ¬âdemonstrate fundamental principles of computer science and can be powerful tools in various programming scenarios. Whether you're solving interview questions, encrypting sensitive data, or analyzing text, understanding these algorithms lays a solid foundation for tackling more complex problems in the realm of software development.
426 views