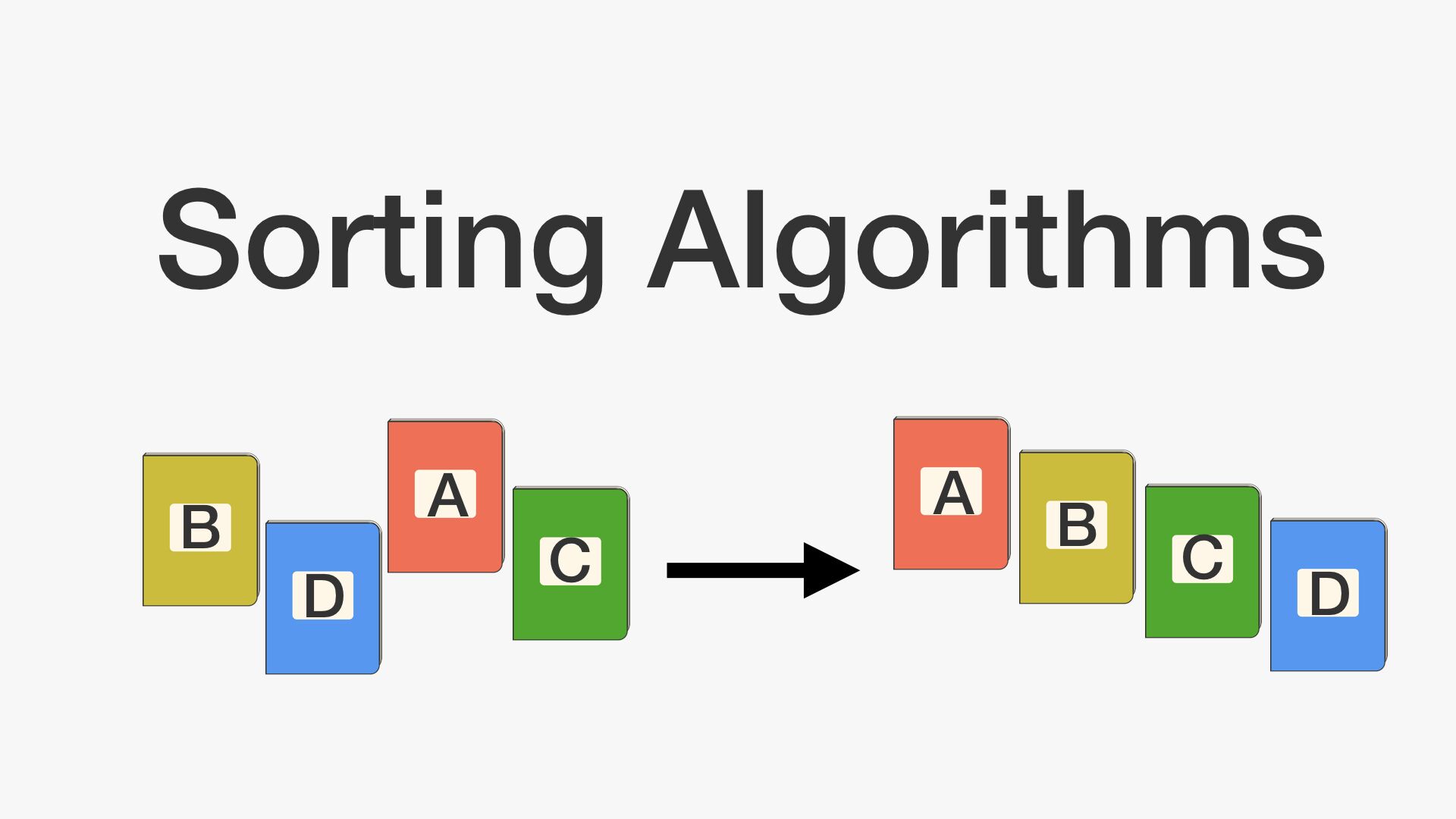
June 26, 2024
Exploring Sorting Algorithms in JavaScript: Bubble Sort, Insertion Sort, and Selection Sort
Sorting algorithms are fundamental tools in computer science, used to rearrange elements in a collection into a particular order. JavaScript provides a versatile platform for implementing and experimenting with various sorting algorithms. In this article, we'll explore three commonly used sorting algorithms: Bubble Sort, Insertion Sort, and Selection Sort, and implement them using JavaScript.
Bubble Sort
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. The pass through the list is repeated until the list is sorted.
function bubbleSort(arr) {
const n = arr.length;
let swapped;
do {
swapped = false;
for (let i = 0; i < n - 1; i++) {
if (arr[i] > arr[i + 1]) {
[arr[i], arr[i + 1]] = [arr[i + 1], arr[i]];
swapped = true;
}
}
} while (swapped);
return arr;
}
// Example usage
const array = [64, 34, 25, 12, 22, 11, 90];
console.log("Bubble Sort:", bubbleSort(array));
Insertion Sort
Insertion Sort builds the final sorted array one item at a time by repeatedly picking the next element and inserting it into the sorted portion of the array.
function insertionSort(arr) {
const n = arr.length;
for (let i = 1; i < n; i++) {
let key = arr[i];
let j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j = j - 1;
}
arr[j + 1] = key;
}
return arr;
}
// Example usage
const array = [64, 34, 25, 12, 22, 11, 90];
console.log("Insertion Sort:", insertionSort(array));
Selection Sort
Selection Sort divides the input list into two parts: the sublist of items already sorted and the sublist of items remaining to be sorted. It repeatedly selects the smallest element from the unsorted sublist and moves it to the end of the sorted sublist.
function selectionSort(arr) {
const n = arr.length;
for (let i = 0; i < n - 1; i++) {
let minIndex = i;
for (let j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
if (minIndex !== i) {
[arr[i], arr[minIndex]] = [arr[minIndex], arr[i]];
}
}
return arr;
}
// Example usage
const array = [64, 34, 25, 12, 22, 11, 90];
console.log("Selection Sort:", selectionSort(array));
Conclusion
Sorting algorithms play a crucial role in various computer science applications. Understanding different sorting algorithms, such as Bubble Sort, Insertion Sort, and Selection Sort, provides insight into algorithmic efficiency and helps in making informed decisions when dealing with large datasets. JavaScript's flexibility allows for easy implementation and experimentation with these algorithms, aiding in the understanding of their mechanics and performance characteristics.
267 views