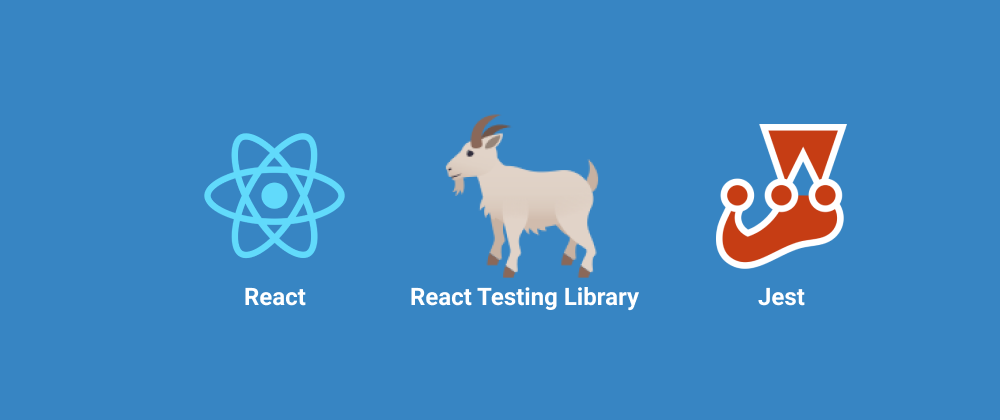
July 12, 2024
Mastering React Application Testing with React Testing Library
In the world of web development, ensuring the reliability and stability of your applications is paramount. Testing plays a crucial role in achieving this, allowing developers to identify and rectify bugs early in the development process. When it comes to testing React applications, one of the most popular and effective tools in a developer's arsenal is React Testing Library (RTL). In this article, we'll delve into the fundamentals of testing React applications using RTL, accompanied by real code examples to illustrate its usage and benefits.
Introduction to React Testing Library (RTL)
React Testing Library is a lightweight and intuitive testing library for React applications. It focuses on testing your application from the user's perspective, encouraging developers to write tests that resemble how users interact with the application. This approach ensures that tests are not tightly coupled to implementation details, making them more robust and resilient to changes in the codebase.
RTL provides a set of utilities to render React components into the DOM, interact with them, and assert on their behavior. Its API is designed to be simple and accessible, making it easy for developers of all skill levels to write effective tests.
Setting Up Your Testing Environment
Before diving into writing tests with RTL, let's ensure our testing environment is properly set up. You can use tools like Jest as your test runner and React Testing Library for rendering and testing React components. Ensure that these dependencies are installed in your project:
npm install --save-dev @testing-library/react @testing-library/jest-dom jest
Once installed, you can configure Jest to work with React Testing Library by adding the following configuration to your package.json:
"jest": {
"preset": "react",
"setupFilesAfterEnv": [
"@testing-library/react/cleanup-after-each",
"@testing-library/jest-dom/extend-expect"
]
}
Writing Your First Test
Let's write a simple test for a hypothetical Button component that increments a counter when clicked. First, create a new file named Button.test.js and add the following code:
import React from 'react';
import { render, fireEvent } from '@testing-library/react';
import Button from './Button';
test('increments counter when clicked', () => {
const { getByText } = render(<Button />);
const button = getByText('Click me');
fireEvent.click(button);
expect(button).toHaveTextContent('1');
});
In this test, we render the Button component, simulate a click event using fireEvent.click, and then assert that the button's text content has changed to '1'.
Testing React Components with RTL
RTL provides a rich set of utilities for testing React components. Some common functions you'll use include:
- render: Renders a React component into the DOM.
- fireEvent: Simulates user events like clicks, typing, etc.
- getBy* queries: Finds elements in the rendered component.
- waitFor: Waits for asynchronous actions to complete.
Let's consider another example where we have a LoginForm component that validates user input:
import React, { useState } from 'react';
function LoginForm() {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const [error, setError] = useState('');
const handleSubmit = () => {
if (!username || !password) {
setError('Please enter both username and password');
} else {
// Logic to submit the form
}
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
placeholder="Username"
value={username}
onChange={(e) => setUsername(e.target.value)}
/>
<input
type="password"
placeholder="Password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
<button type="submit">Login</button>
{error && <div data-testid="error">{error}</div>}
</form>
);
}
export default LoginForm;
To test this component, we can write the following test:
import React from 'react';
import { render, fireEvent, waitFor } from '@testing-library/react';
import LoginForm from './LoginForm';
test('validates login form', async () => {
const { getByPlaceholderText, getByText, getByTestId } = render(<LoginForm />);
// Attempt to submit form without entering any data
fireEvent.submit(getByText('Login'));
// Error message should appear
await waitFor(() => expect(getByTestId('error')).toHaveTextContent('Please enter both username and password'));
// Enter valid username and password
fireEvent.change(getByPlaceholderText('Username'), { target: { value: 'user' } });
fireEvent.change(getByPlaceholderText('Password'), { target: { value: 'pass' } });
// Submit form
fireEvent.submit(getByText('Login'));
// Assert on successful submission
expect(/* Assert on success condition */).toBeTruthy();
});
In this test, we simulate form submission without entering any data, validate the appearance of the error message, and then submit the form with valid credentials. Finally, we assert on the successful submission of the form.
Conclusion
React Testing Library simplifies the process of testing React applications by encouraging developers to write tests that focus on user behavior rather than implementation details. By following the principles of RTL and leveraging its intuitive API, developers can create robust and maintainable test suites that ensure the reliability and stability of their applications. With real code examples, we've explored how to write tests for React components using RTL, empowering developers to build high-quality React applications with confidence.
296 views