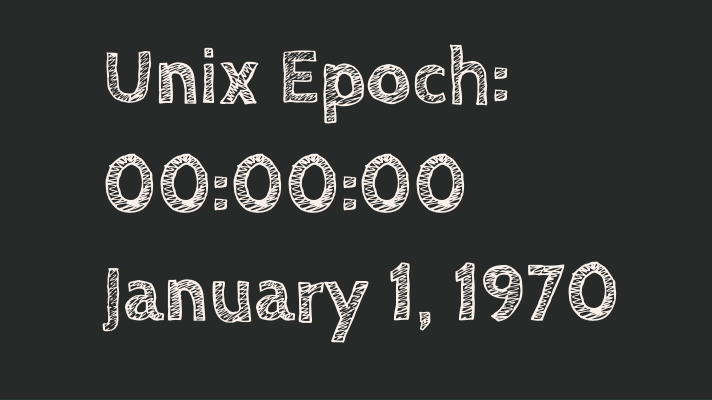
April 11, 2024
Understanding Epoch Time in JavaScript and How to Remove Milliseconds
Introduction
In modern web development, understanding time is crucial for various tasks, such as scheduling events, managing timestamps, or synchronizing data across different systems. Epoch time, also known as Unix time or POSIX time, serves as a fundamental concept for representing time in many programming languages, including JavaScript. In this article, we'll delve into what epoch time is, its significance in JavaScript, and how to remove milliseconds from epoch time.
What is Epoch Time?
Epoch time is a standard for representing time as the number of seconds that have elapsed since the Unix epoch - 00:00:00 Coordinated Universal Time (UTC) on January 1, 1970, not counting leap seconds. It's essentially a timestamp that serves as a reference point for measuring time in various computing systems. This system simplifies timekeeping by providing a consistent, linear representation of time across different platforms and programming languages.
Epoch Time in JavaScript:
In JavaScript, obtaining the current epoch time is straightforward, thanks to the built-in Date.now() method. This method returns the number of milliseconds that have elapsed since the Unix epoch. While milliseconds granularity is often useful, there are situations where you may need epoch time without the millisecond part, for example, when interacting with APIs or databases that expect timestamps in seconds rather than milliseconds.
Removing Milliseconds in JavaScript:
To remove milliseconds from epoch time in JavaScript, we can leverage simple arithmetic operations and functions provided by the language. Here's a basic approach:
// Get current epoch time in milliseconds
let epochTimeInMillis = Date.now();
// Convert epoch time to seconds (remove milliseconds)
let epochTimeInSeconds = Math.floor(epochTimeInMillis / 1000);
In this snippet, Math.floor() is used to round down the result of dividing the epoch time by 1000, effectively removing the milliseconds part and leaving us with epoch time in seconds.
Alternatively, you can use parseInt() to achieve the same result:
// Get current epoch time in milliseconds
let epochTimeInMillis = Date.now();
// Convert epoch time to seconds (remove milliseconds)
let epochTimeInSeconds = parseInt(epochTimeInMillis / 1000);
Both methods produce the current epoch time without the millisecond part, allowing for cleaner representation and compatibility with systems that expect timestamps in seconds.
Conclusion:
Epoch time is a fundamental concept in JavaScript and other programming languages, providing a standardized way to represent time as the number of seconds since the Unix epoch. By understanding how to obtain epoch time and remove milliseconds in JavaScript, developers can ensure compatibility and consistency when working with timestamps across different systems and platforms. Whether it's scheduling tasks, managing data, or interacting with external APIs, epoch time serves as a reliable foundation for timekeeping in modern web development.
567 views