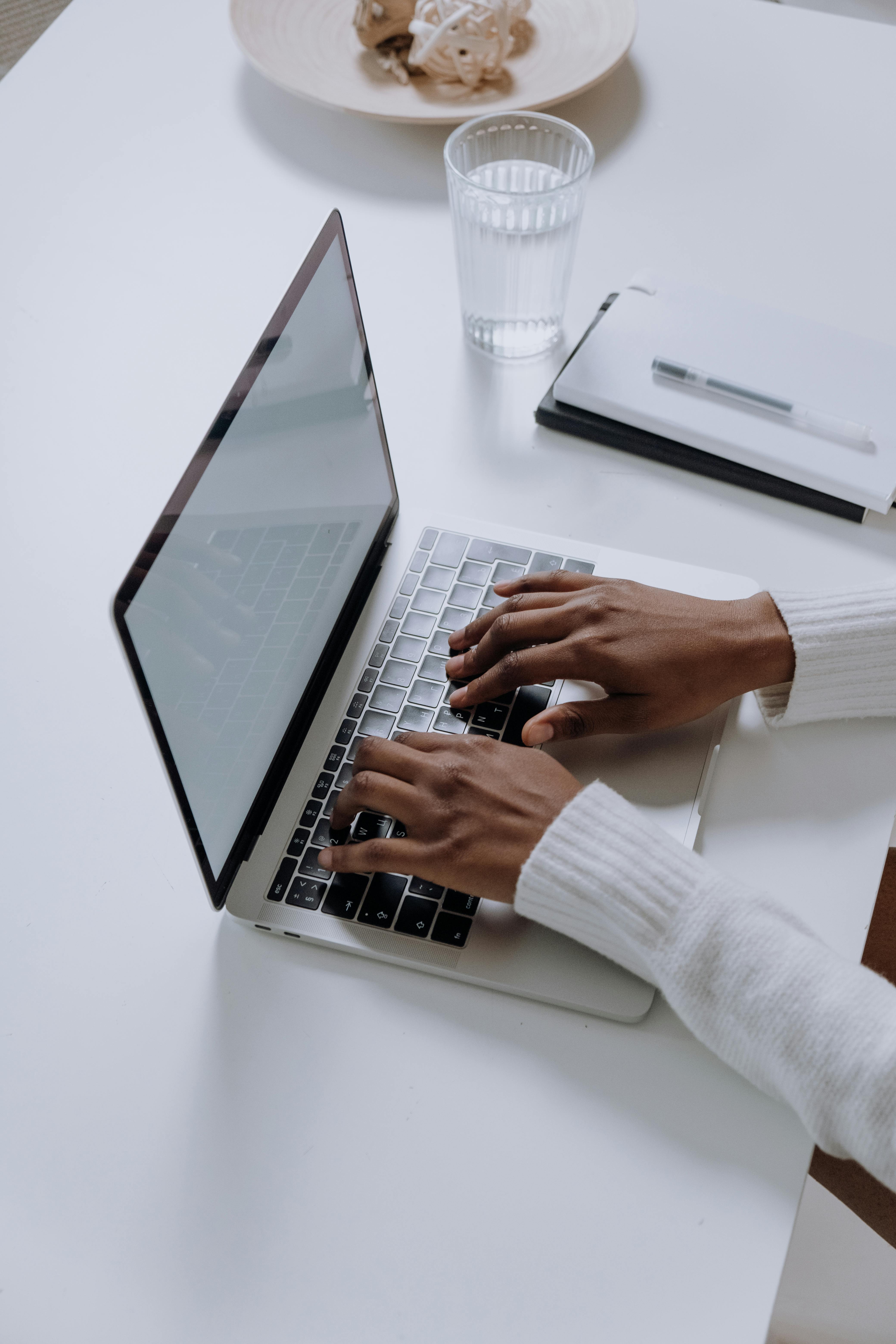
July 23, 2024
Simplify Input Handling in SwiftUI: Allowing Only Numeric Input
Introduction:
In our increasingly digital world, simplifying user interactions has become paramount. Whether it's in a finance app, a calculator, or a simple input form, ensuring that users can input data quickly and accurately is crucial. One common requirement is to limit user input to numeric values, allowing for both integers and decimals. In this blog post, we'll explore how to achieve this in SwiftUI, Apple's modern framework for building user interfaces, and delve into a situation where this capability could be invaluable.
Scenario:
Imagine you're developing a budgeting application to help users track their expenses. You want users to input transaction amounts quickly and easily, but you also need to ensure that only valid numeric values are accepted. Additionally, allowing decimal input is necessary to accurately capture fractional amounts.
Implementation:
Using SwiftUI, we can leverage the TextField component to capture user input and then filter out non-numeric characters. Here's how we can achieve this:
import SwiftUI
struct ContentView: View {
@State private var userInput = ""
var body: some View {
VStack {
TextField("Enter transaction amount", text: $userInput)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding()
.onChange(of: userInput) { newValue in
// Filter out non-numeric characters and allow decimal point
self.userInput = newValue.filter { "0123456789.".contains($0) }
// Remove multiple decimal points
if let range = self.userInput.range(of: ".",
options: .backwards),
self.userInput.filter({ $0 == "." }).count > 1 {
self.userInput.removeSubrange(range.lowerBound..<self.userInput.endIndex)
}
}
Text("Transaction amount: \(userInput)")
.padding()
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Explanation:
- The TextField component captures user input into the userInput variable.
- The onChange modifier listens for changes in the userInput and filters out non-numeric characters, allowing only digits (0-9) and the decimal point ('.').
- Additionally, it ensures that only one decimal point is allowed by removing any subsequent occurrences.
- Finally, the filtered input is displayed in a Text view below the text field.
Conclusion:
By implementing input handling logic that allows only numeric input, we've simplified the user experience in our budgeting application. Users can now input transaction amounts quickly and accurately, without the hassle of dealing with non-numeric characters. This approach not only enhances usability but also ensures the integrity of the data captured by the application.
In conclusion, when designing user interfaces, especially in scenarios where precise numeric input is required, leveraging SwiftUI's capabilities to filter and validate user input can greatly improve the user experience and the overall effectiveness of your application.
579 views