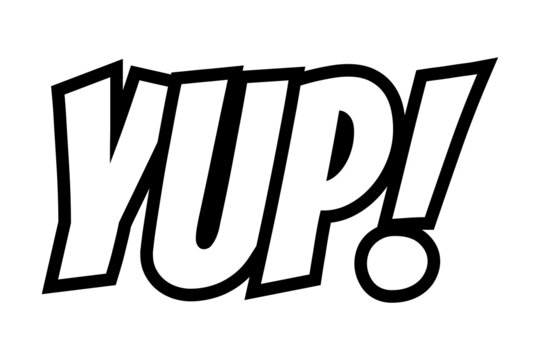
July 01, 2024
Ensuring Data Integrity with Implementation Validation in Next.js using Yup
In the world of web development, ensuring the integrity of user data is paramount. Whether you're building a simple contact form or a complex data-driven application, validating user input is essential for maintaining data consistency and preventing errors. With the rise of Next.js as a popular framework for building React applications, developers have access to powerful tools like Yup to streamline the validation process.
Yup is a JavaScript schema builder for value parsing and validation. It provides a simple and declarative way to define validation rules for data objects, making it an ideal choice for implementing validation in Next.js applications. In this blog post, we'll explore how Yup can be integrated into a Next.js project to validate user input and ensure data integrity.
Why Validation Matters
Before diving into the implementation details, let's briefly discuss why validation is crucial in web development. When users interact with web forms or input fields, they often provide data that needs to adhere to specific constraints. For example, an email address must have a valid format, a password must meet certain complexity requirements, and a date must be in a valid format.
Without proper validation, the data entered by users can lead to various issues such as:
- Data inconsistency
- Security vulnerabilities (e.g., SQL injection, XSS attacks)
- Application crashes or errors
- Poor user experience
By implementing validation, developers can ensure that the data submitted by users meets the required criteria, thereby reducing the risk of errors and maintaining the integrity of the application's data.
Integrating Yup with Next.js
Next.js provides a robust framework for building server-rendered React applications. Integrating Yup with Next.js is straightforward and can be done in a few simple steps:
Install Yup: Begin by installing Yup as a dependency in your Next.js project. You can do this using npm or yarn:
npm install yup
OR
yarn add yup
Define Validation Schema:
Next, define a validation schema using Yup to specify the validation rules for your data objects. You can create a separate module to encapsulate your validation logic, making it reusable across different parts of your application. Here's an example of how you might define a schema for a user registration form:
import * as Yup from 'yup';
const registrationSchema = Yup.object().shape({
username: Yup.string().required('Username is required'),
email: Yup.string().email('Invalid email address').required('Email is required'),
password: Yup.string().min(8, 'Password must be at least 8 characters').required('Password is required'),
});
export default registrationSchema;
In this schema, we're specifying that the username, email, and password fields are required, and we're also applying additional validation rules such as minimum password length and email format.
Perform Validation: Once you have defined your validation schema, you can use it to validate user input in your Next.js components or API routes. For example, you might validate form data before submitting it to a server or before updating state in a React component. Here's how you can use Yup to validate form data in a Next.js component:
import { useState } from 'react';
import { useFormik } from 'formik';
import registrationSchema from '../validation/registrationSchema';
function RegistrationForm() {
const [registrationError, setRegistrationError] = useState('');
const formik = useFormik({
initialValues: {
username: '',
email: '',
password: '',
},
validationSchema: registrationSchema,
onSubmit: async (values) => {
try {
// Perform registration logic
} catch (error) {
setRegistrationError('An error occurred during registration');
}
},
});
return (
<form onSubmit={formik.handleSubmit}>
<input
type="text"
name="username"
value={formik.values.username}
onChange={formik.handleChange}
onBlur={formik.handleBlur}
/>
{formik.touched.username && formik.errors.username && <div>{formik.errors.username}</div>}
{/* Repeat for other form fields (email, password) */}
<button type="submit">Register</button>
{registrationError && <div>{registrationError}</div>}
</form>
);
}
export default RegistrationForm;
In this example, we're using Formik for form management and Yup for validation. The validationSchema prop of the useFormik hook is set to our previously defined Yup schema (registrationSchema). Formik automatically handles form validation based on this schema, and any validation errors are displayed to the user.
Conclusion
Implementing validation in Next.js applications using Yup is a powerful way to ensure data integrity and enhance the user experience. By defining validation schemas and applying them to user input, developers can enforce data constraints and prevent errors from occurring. Whether you're building a simple form or a complex data-driven application, integrating Yup with Next.js provides a robust solution for validating user input and maintaining the integrity of your application's data.
461 views