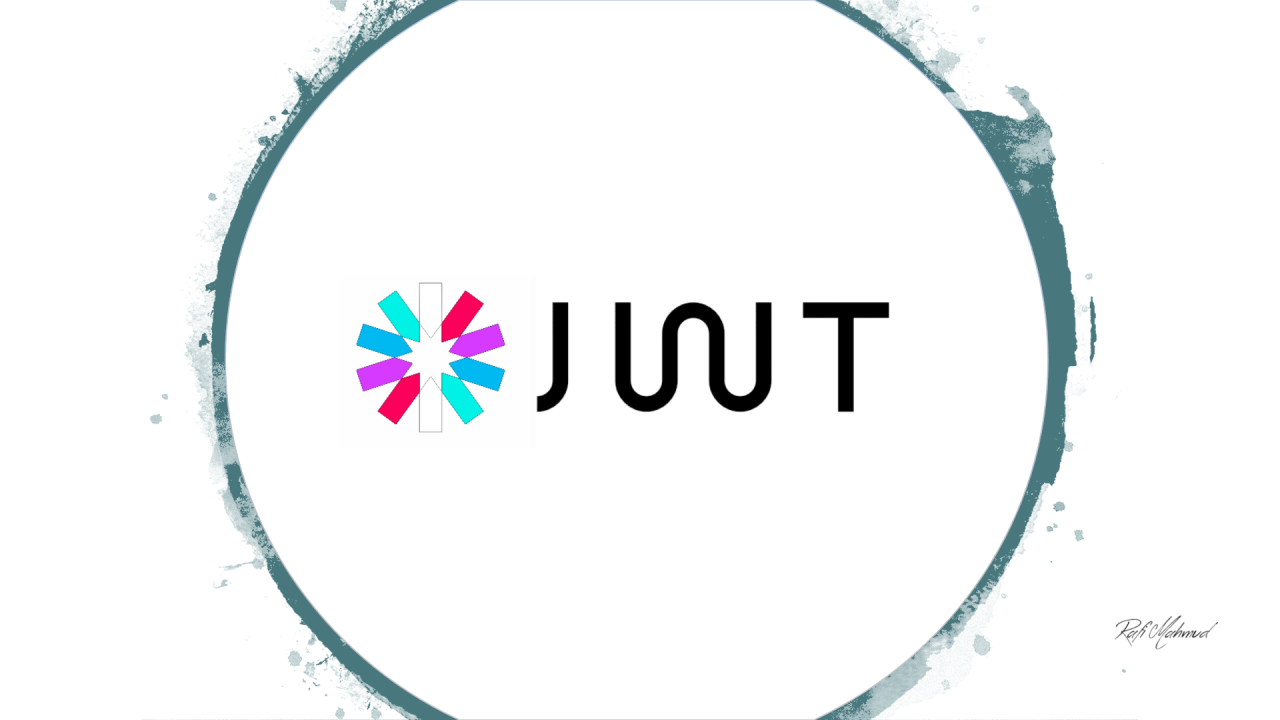
April 05, 2024
Exploring the Differences Between Sessions-Cookies Authentication and JWT Authentication in Node.js with Express.js
Authentication is a fundamental aspect of web development, ensuring that users are who they claim to be before granting access to resources or features. In the realm of Node.js web applications built with Express.js, two popular authentication methods stand out: sessions-cookies authentication and JSON Web Token (JWT) authentication. Each has its own strengths and weaknesses, and understanding the differences between them is crucial for building secure and efficient web applications. In this blog post, we'll delve into the nuances of both authentication methods and explore their implementation in a Node.js environment using Express.js.
Sessions-Cookies Authentication
Sessions-cookies authentication is a traditional method widely used in web development. It relies on server-side sessions and browser cookies to manage user authentication. Here's how it typically works:
Login Process: When a user logs in, the server validates the credentials and creates a session for the user. A unique session identifier (session ID) is generated and stored on the server. Meanwhile, a session cookie containing the session ID is sent to the client's browser.
Subsequent Requests: With each subsequent request, the client's browser sends the session cookie back to the server. The server then looks up the session associated with the provided session ID and validates the user's identity.
Session Management: Sessions can be stored either in memory on the server or in a persistent data store such as a database. The server maintains session state and manages session expiration.
JSON Web Token (JWT) Authentication
JWT authentication is a stateless authentication method that has gained popularity due to its simplicity and scalability. It utilizes digitally signed tokens to verify the authenticity of users. Here's how it works:
Token Generation: When a user logs in, the server generates a JWT containing relevant user information (e.g., user ID, roles) and signs it using a secret key. The signed JWT is then sent to the client.
Token Verification: With each subsequent request, the client sends the JWT in the request headers. The server verifies the integrity of the token by validating the signature using the secret key. If the signature is valid, the server extracts the user information from the token.
Statelessness: Unlike sessions, JWT authentication is stateless, meaning the server does not need to store session data. This makes JWTs ideal for distributed and scalable applications.
Implementation in Node.js with Express.js
Let's take a closer look at how you can implement both authentication methods in a Node.js application using Express.js:
Sessions-Cookies Authentication Implementation
const express = require('express');
const session = require('express-session');
const app = express();
app.use(session({
secret: 'your-secret-key',
resave: false,
saveUninitialized: true,
cookie: { secure: true }
}));
app.post('/login', (req, res) => {
// Validate credentials
// If valid, create session
req.session.userId = 'user123'; // Example user ID
res.send('Login successful');
});
app.get('/profile', (req, res) => {
// Retrieve user data from session
const userId = req.session.userId;
// Fetch user profile using userId
res.send('User profile: ' + userId);
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
JWT Authentication Implementation
const express = require('express');
const jwt = require('jsonwebtoken');
const app = express();
const secretKey = 'your-secret-key';
app.post('/login', (req, res) => {
// Validate credentials
// If valid, generate JWT
const token = jwt.sign({ userId: 'user123' }, secretKey);
res.json({ token });
});
app.get('/profile', authenticateToken, (req, res) => {
// User is authenticated, retrieve profile
res.send('User profile: ' + req.user.userId);
});
function authenticateToken(req, res, next) {
const authHeader = req.headers['authorization'];
const token = authHeader && authHeader.split(' ')[1];
if (token == null) return res.sendStatus(401);
jwt.verify(token, secretKey, (err, user) => {
if (err) return res.sendStatus(403);
req.user = user;
next();
});
}
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Conclusion
Both sessions-cookies authentication and JWT authentication offer different approaches to user authentication in Node.js applications. Sessions-cookies authentication relies on server-side sessions and browser cookies for user verification, while JWT authentication uses digitally signed tokens for stateless authentication.
When choosing between these methods, consider factors such as scalability, security, and ease of implementation. Sessions-cookies authentication may be simpler to implement for smaller applications, while JWT authentication offers better scalability and flexibility for larger, distributed systems.
By understanding the differences and implementations of these authentication methods, you can choose the one that best fits your project's requirements and build secure, efficient Node.js applications with Express.js
596 views