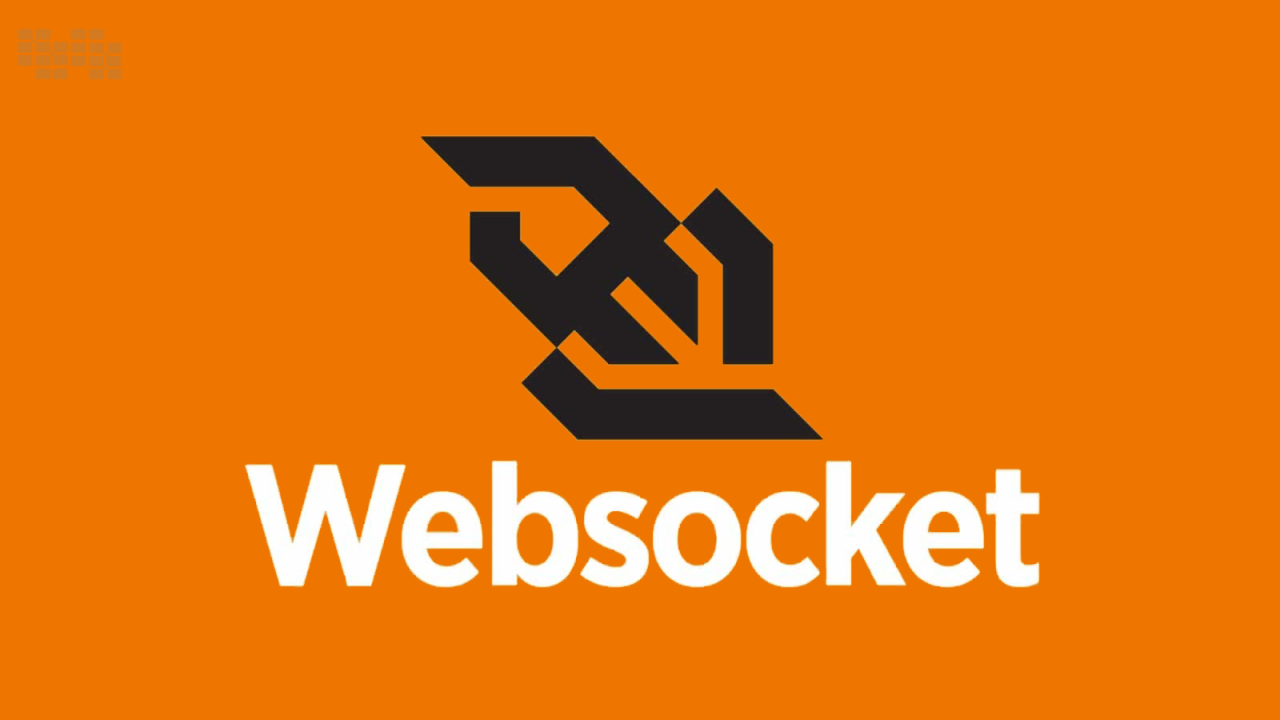
June 07, 2024
Implementing a Simple Chat Application using WebSocket
Share what you learn in this blog to prepare for your interview, create your forever-free profile now, and explore how to monetize your valuable knowledge.
Introduction:
WebSocket is a powerful communication protocol that enables real-time, full-duplex communication between a client and a server over a single, long-lived connection. In this article, we'll explore how to build a simple chat application using WebSocket in Python.
1. Setting up the Server:
import asyncio
import websockets
async def chat_server(websocket, path):
async for message in websocket:
# Broadcast the received message to all connected clients
await asyncio.gather(*[ws.send(message) for ws in connected])
async def main():
server = await websockets.serve(chat_server, "localhost", 8765)
await server.wait_closed()
connected = set()
asyncio.run(main())
2. Setting up the Client:
import asyncio
import websockets
async def chat_client():
async with websockets.connect("ws://localhost:8765") as websocket:
while True:
message = input("Enter message: ")
await websocket.send(message)
asyncio.run(chat_client())
3. Running the Application:
- Start the server by running the server code.
- Run the client code in multiple terminals to simulate multiple users.
- Users can now send messages, and the server will broadcast them to all connected clients.
Conclusion:
In this article, we've demonstrated how to implement a simple chat application using WebSocket in Python. WebSocket's real-time capabilities make it ideal for building interactive applications such as chat platforms, multiplayer games, and collaborative tools.
483 views
Please Login to create a Question