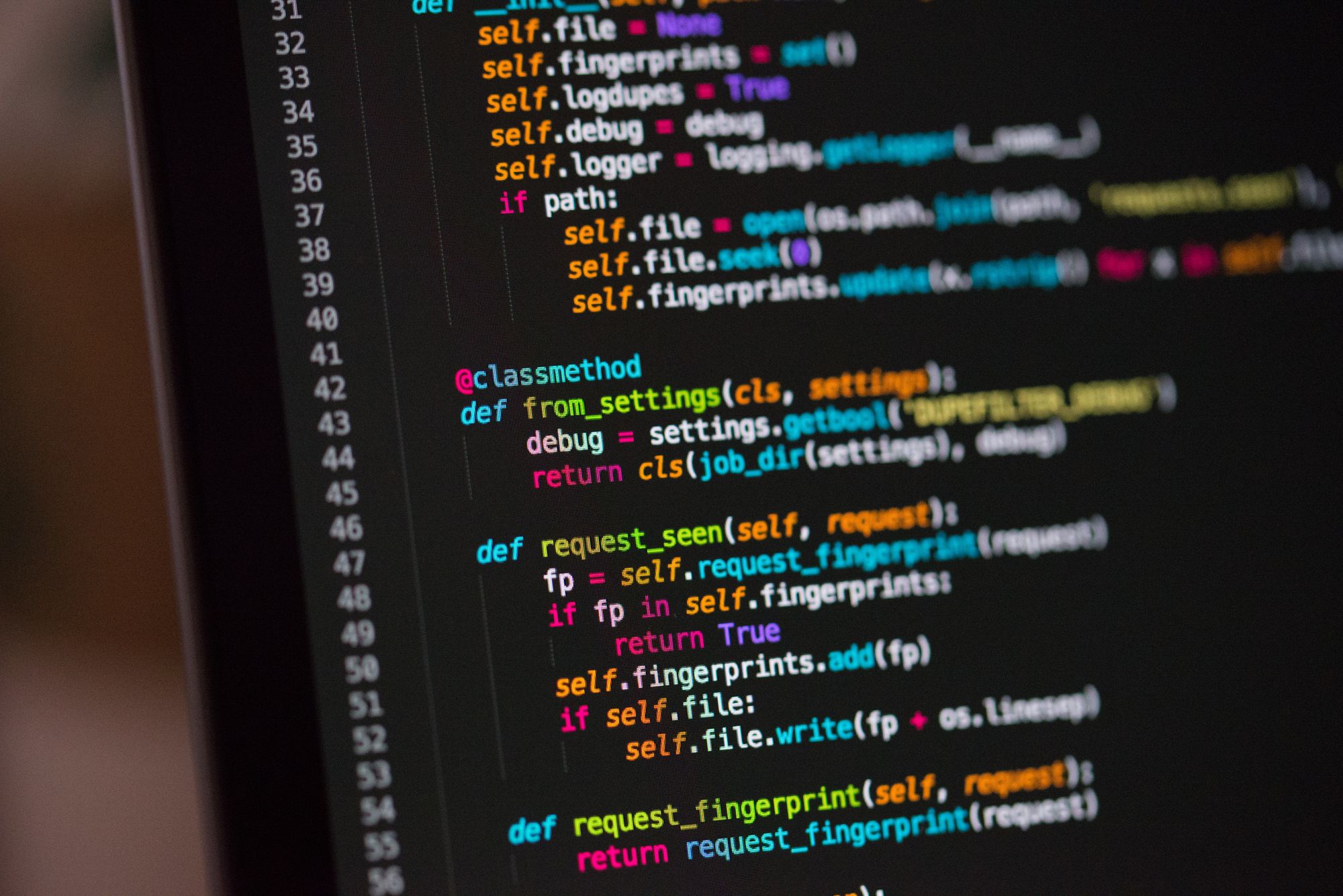
June 24, 2024
Mastering Medium-Level Array Interview Questions: A Step-by-Step Guide
Array-based questions are a staple in technical interviews, testing candidates' problem-solving skills and understanding of fundamental data structures. Medium-level array questions often present unique challenges that require a combination of intuition and algorithmic thinking to solve efficiently. In this article, we'll tackle four such medium-level array interview questions, unraveling the thought process behind each solution.
Finding the Missing Number:
Problem: Given an array containing n distinct numbers taken from 0, 1, 2, ..., n, find the one that is missing from the array.
Solution: This problem can be efficiently solved using the mathematical formula for the sum of the first n natural numbers. We calculate the expected sum and subtract the sum of the given array to find the missing number. The code for this solution looks like this:
def missingNumber(nums):
n = len(nums)
expected_sum = n * (n + 1) // 2
actual_sum = sum(nums)
return expected_sum - actual_sum
Maximum Subarray:
Problem: Find the contiguous subarray within an array (containing at least one number) that has the largest sum and return its sum.
Solution: Kadane's algorithm offers an efficient solution to this problem, iterating through the array and keeping track of the maximum subarray sum encountered so far. At each step, we either extend the current subarray or start a new subarray from the current element. The code for Kadane's algorithm implementation:
def maxSubArray(nums):
max_sum = current_sum = nums[0]
for num in nums[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
Rotate Array:
Problem: Given an array, rotate the array to the right by k steps, where k is a non-negative integer.
Solution: There are multiple approaches to solve this problem, such as using extra space, reversing the array in parts, or using cyclic replacements. One of the efficient solutions involves using cyclic replacements. We repeatedly swap elements to achieve the rotation. The code for this solution:
def rotate(nums, k):
n = len(nums)
k %= n
count = start = 0
while count < n:
current = start
prev = nums[start]
while True:
next_idx = (current + k) % n
nums[next_idx], prev = prev, nums[next_idx]
current = next_idx
count += 1
if start == current:
break
start += 1
Product of Array Except Self:
Problem: Given an array nums of n integers where n > 1, return an array output such that output[i] is equal to the product of all the elements of nums except nums[i].
Solution: We can solve this problem by calculating the prefix and suffix products for each element. The product of all elements except itself can be obtained by multiplying the corresponding prefix and suffix products. The code for this solution:
def productExceptSelf(nums):
n = len(nums)
result = [1] * n
left_product = right_product = 1
for i in range(n):
result[i] *= left_product
result[n - 1 - i] *= right_product
left_product *= nums[i]
right_product *= nums[n - 1 - i]
return result
By mastering these medium-level array interview questions and understanding the logic behind each solution, you'll be better equipped to tackle similar problems and showcase your problem-solving skills effectively in technical interviews. Remember, practice and understanding are key to success in tackling array-based challenges.
520 views