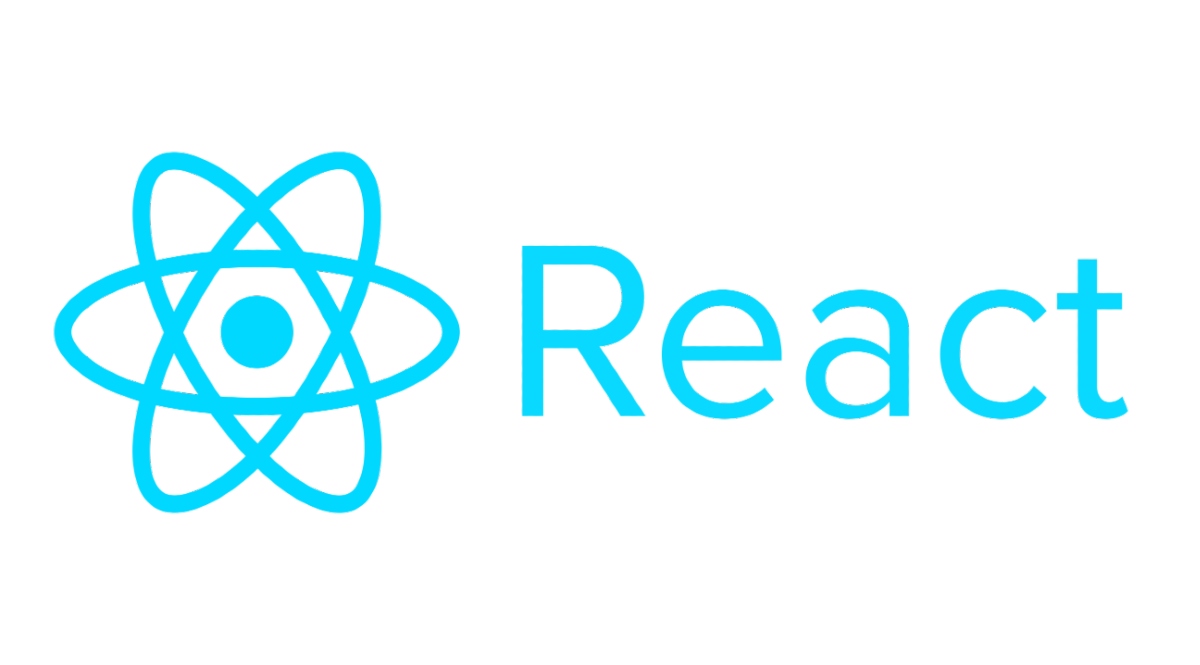
April 23, 2024
Bringing Your React App to Life: A Beginner's Guide to Server-Side Rendering
Are you tired of waiting for your React app to load, only to see a blank screen while it fetches data? Do you dream of a smoother user experience and better SEO for your application? If so, it might be time to consider implementing server-side rendering (SSR) into your React project.
In this guide, we'll take a journey together to learn how to implement SSR from scratch. Don't worry if you're new to the concept â we'll break it down step by step, with plenty of code examples to help you along the way.
What is Server-Side Rendering?
Before we dive into the implementation, let's quickly go over what SSR actually means. In traditional client-side rendering, the browser downloads a minimal HTML file, then JavaScript takes over to render the rest of the page. This approach often leads to slower initial load times and poorer SEO performance.
SSR, on the other hand, involves rendering the initial HTML on the server and sending it to the browser, which can improve performance, especially for users on slower connections. Additionally, search engines can easily crawl and index SSR pages, leading to better discoverability.
Getting Started
To begin, let's create a new React project. Open your terminal and run:
npx create-react-app my-ssr-app
cd my-ssr-app
Next, we'll install a few dependencies that we'll need later:
npm install express react-dom react-router-dom
Setting Up the Server
Now, let's create a simple Express server to handle SSR. Create a file named server.js in the root of your project directory and add the following code:
const express = require('express');
const React = require('react');
const ReactDOMServer = require('react-dom/server');
const { StaticRouter } = require('react-router-dom');
const App = require('./src/App').default;
const server = express();
const PORT = process.env.PORT || 3000;
server.use(express.static('build'));
server.get('*', (req, res) => {
const context = {};
const app = ReactDOMServer.renderToString(
<StaticRouter location={req.url} context={context}>
<App />
</StaticRouter>
);
const html = `
<!DOCTYPE html>
<html>
<head>
<title>SSR React App</title>
</head>
<body>
<div id="root">${app}</div>
<script src="/static/js/main.chunk.js"></script>
<script src="/static/js/vendors~main.chunk.js"></script>
<script src="/static/js/bundle.js"></script>
</body>
</html>
`;
res.send(html);
});
server.listen(PORT, () => {
console.log(`Server is listening on port ${PORT}`);
});
This code sets up an Express server that serves our React application. It renders the React app to a string using ReactDOMServer.renderToString() and sends it to the client as HTML. We're using StaticRouter from react-router-dom to handle routing on the server side.
Integrating with Your React App
Now, let's make some adjustments to our React app to make it SSR-ready. Open src/index.js and modify it as follows:
import React from 'react';
import ReactDOM from 'react-dom';
import { BrowserRouter } from 'react-router-dom';
import App from './App';
const renderMethod = module.hot ? ReactDOM.render : ReactDOM.hydrate;
renderMethod(
<BrowserRouter>
<App />
</BrowserRouter>,
document.getElementById('root')
);
Testing It Out
That's it! You've successfully implemented SSR in your React app. Now, start your server by running:
node server.js
Visit http://localhost:3000 in your browser, and you should see your React app rendered with SSR.
Wrapping Up
Implementing server-side rendering in a React app might seem daunting at first, but as you've seen, it's not as complex as it sounds. By following this guide and understanding the basics, you can significantly improve the performance and SEO of your application.
Remember, this is just the beginning. There are many ways to optimize and enhance your SSR setup, such as data fetching strategies, caching, and code splitting. Keep exploring, experimenting, and learning, and your React app will continue to evolve and improve.
290 views